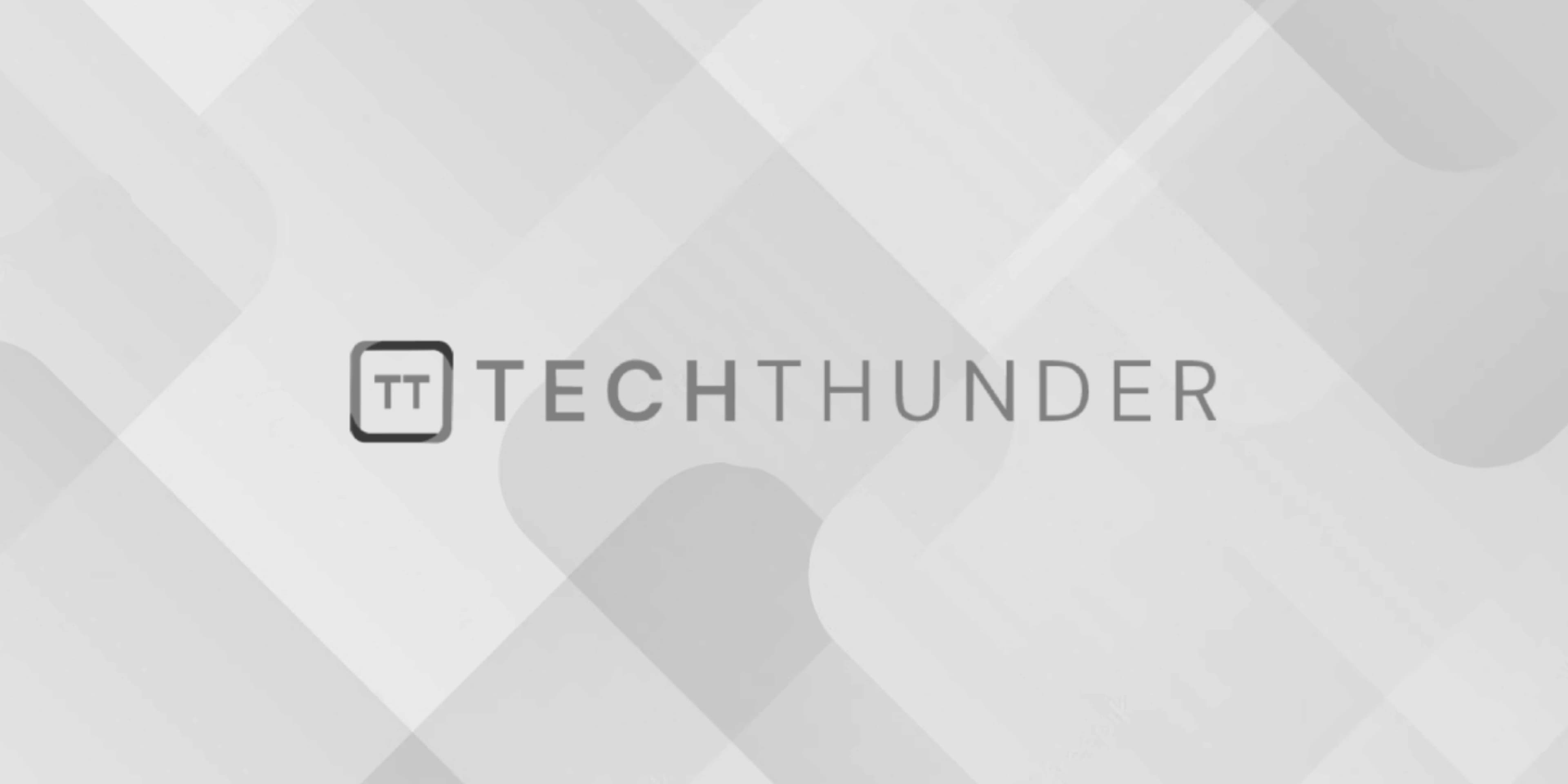
218 views
PHP find value in an array
In PHP, you can find a specific value in an array using various methods, depending on what you want to achieve. Here are some common approaches to find a value in an array:
- Using in_array() function:
Thein_array()
function checks if a given value exists in an array and returns true if the value is found, or false otherwise.
Example:
$fruits = array("apple", "banana", "orange", "grape");
$searchValue = "banana";
if (in_array($searchValue, $fruits)) {
echo "$searchValue exists in the array.";
} else {
echo "$searchValue does not exist in the array.";
}
- Using array_search() function:
Thearray_search()
function searches for a value in an array and returns its corresponding key (index) if found, or false if not found.
Example:
$fruits = array("apple", "banana", "orange", "grape");
$searchValue = "banana";
$key = array_search($searchValue, $fruits);
if ($key !== false) {
echo "$searchValue found at index $key in the array.";
} else {
echo "$searchValue not found in the array.";
}
- Using array_keys() function:
Thearray_keys()
function returns all the keys (indexes) of an array that contain a specific value. If the value is not found, it returns an empty array.
Example:
$fruits = array("apple", "banana", "orange", "grape");
$searchValue = "banana";
$keys = array_keys($fruits, $searchValue);
if (!empty($keys)) {
echo "$searchValue found at indexes: " . implode(", ", $keys);
} else {
echo "$searchValue not found in the array.";
}
- Using array_filter() function:
Thearray_filter()
function can be used with a custom callback function to filter an array and return all elements that match a specific value.
Example:
$fruits = array("apple", "banana", "orange", "grape");
$searchValue = "banana";
$filteredArray = array_filter($fruits, function ($value) use ($searchValue) {
return $value === $searchValue;
});
if (!empty($filteredArray)) {
echo "$searchValue exists in the array.";
} else {
echo "$searchValue does not exist in the array.";
}
Choose the method that best suits your needs based on whether you want to check for the existence of the value, retrieve its key (index), or obtain all keys (indexes) containing the value.