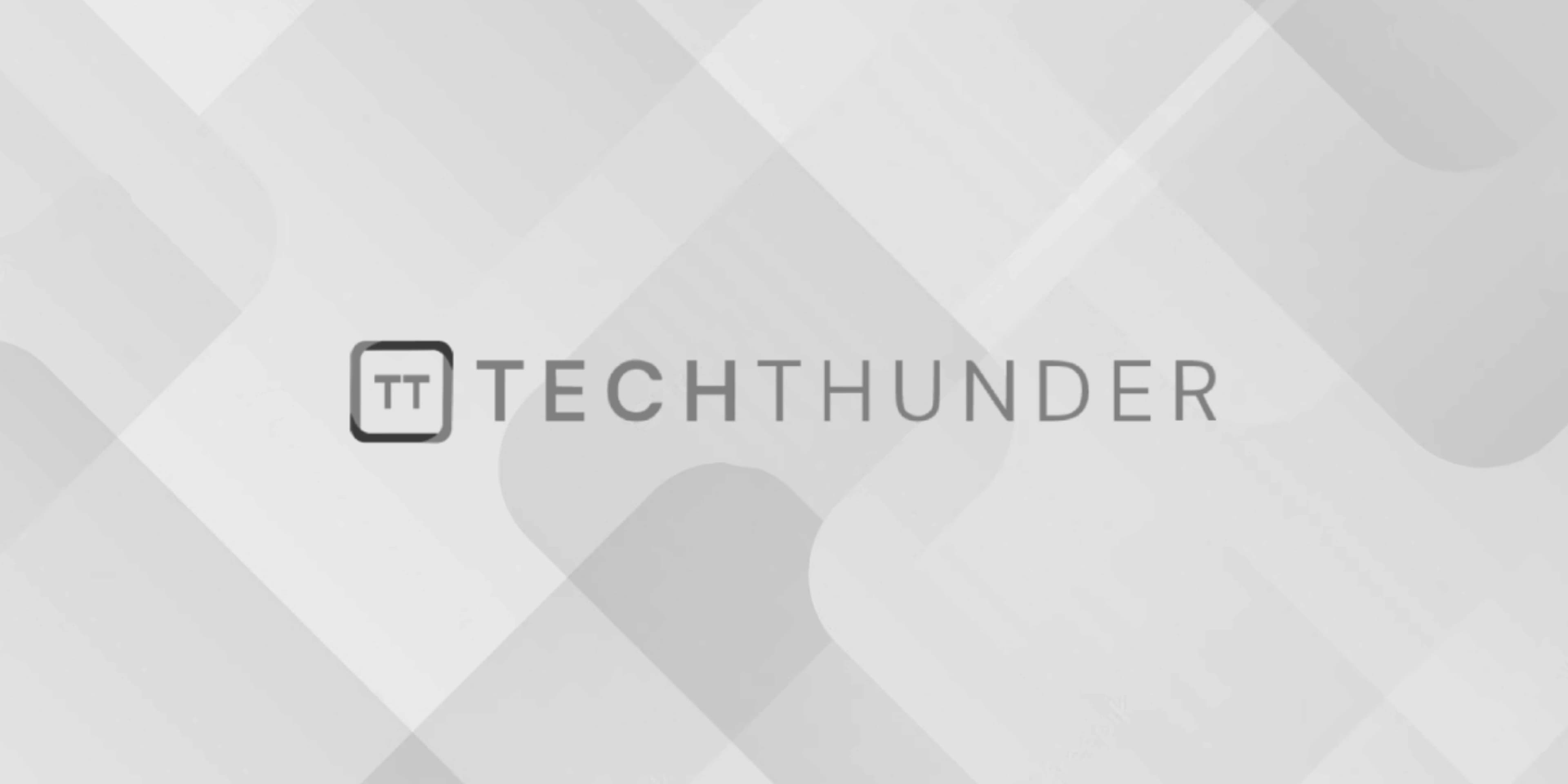
PHP require_once
In PHP, require_once
is a language construct used to include and execute a specified file in a PHP script. It is similar to the require
statement but with one important difference: require_once
ensures that the file is included only once in the script, even if it is called multiple times. This prevents duplicate inclusion of the same file and avoids errors that may arise from redeclaring functions or classes.
The require_once
statement is commonly used to include files that contain important functions, configurations, or class definitions that are needed throughout the application.
Syntax:
require_once 'path/to/file.php';
Example:
Let’s assume we have a file named config.php
that contains some configuration settings and a file named functions.php
that contains custom functions.
config.php:
<?php
$config = array(
'db_host' => 'localhost',
'db_user' => 'username',
'db_pass' => 'password',
'db_name' => 'database'
);
?>
functions.php:
<?php
function add($a, $b) {
return $a + $b;
}
?>
main.php:
<?php
// Include the config.php file
require_once 'config.php';
// Use the configuration settings
echo $config['db_host']; // Output: localhost
// Include the functions.php file
require_once 'functions.php';
// Use the add function from functions.php
$result = add(10, 5);
echo $result; // Output: 15
?>
In this example, main.php
includes config.php
and functions.php
using require_once
. If main.php
is included in other scripts or files using require_once
, the config.php
and functions.php
files will not be included again, ensuring that the configuration settings are not redefined, and the add
function is not redeclared.
Using require_once
helps manage file inclusion effectively in PHP applications and prevents common errors related to redeclaration of functions or variables when files are included multiple times.