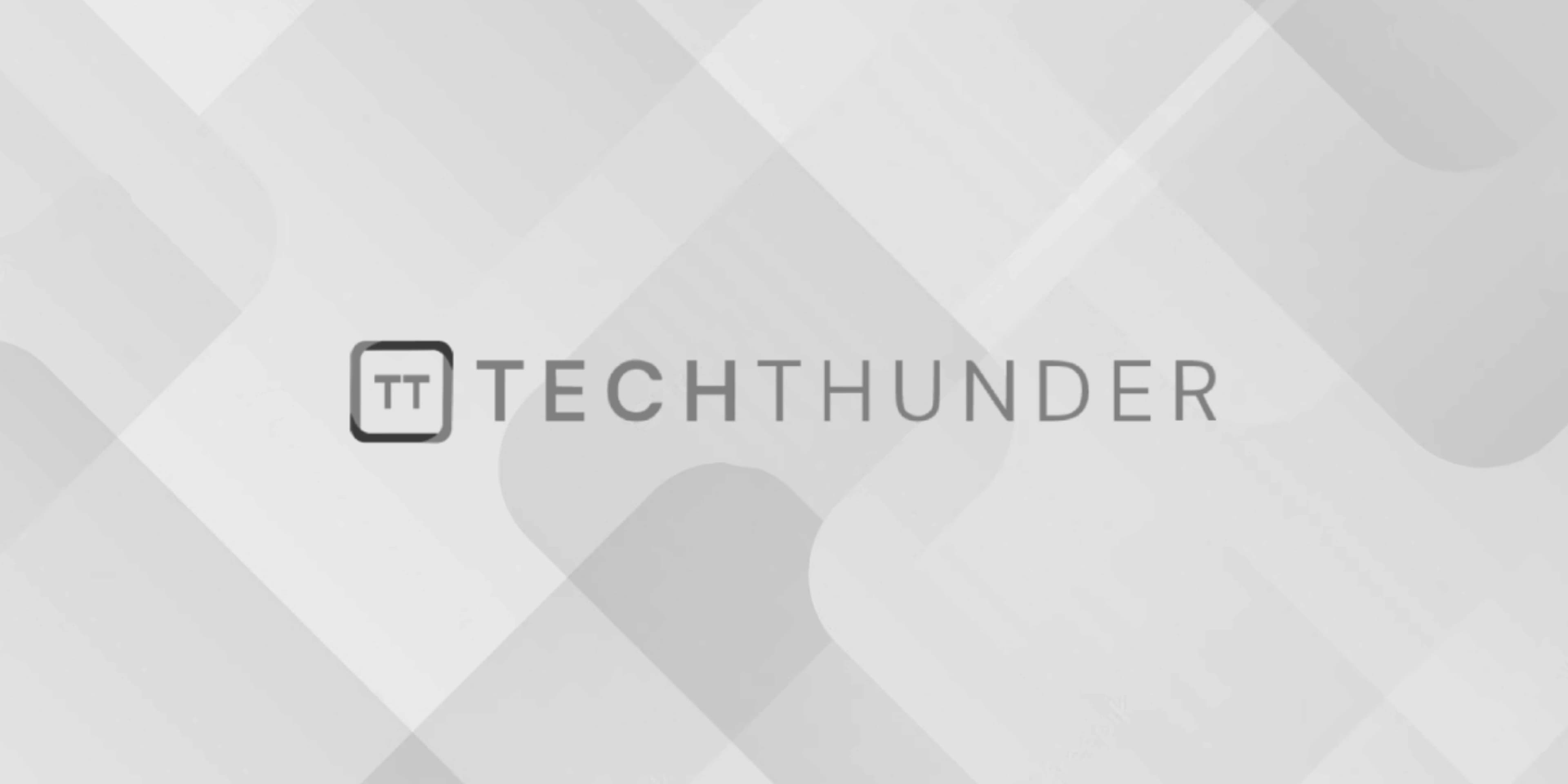
PHP isset() function
In PHP, isset()
is a built-in function used to determine whether a variable has been set and is not null
. It is commonly used to check if a variable exists before attempting to use it to avoid potential errors or warnings.
Syntax:
The syntax for isset()
is as follows:
isset($variable);
Parameters:
$variable
: The variable you want to check.
Return Value:
The isset()
function returns a boolean value (true
or false
). It returns true
if the variable exists and is not null
, and false
if the variable is not set or is null
.
Example:
$name = "John";
$age = 30;
$city = null;
if (isset($name)) {
echo "Name is set: " . $name . "<br>";
}
if (isset($age)) {
echo "Age is set: " . $age . "<br>";
}
if (isset($city)) {
echo "City is set: " . $city . "<br>";
} else {
echo "City is not set.";
}
Output:
Name is set: John
Age is set: 30
City is not set.
In this example, the isset()
function is used to check the existence of the variables $name
, $age
, and $city
. The first two variables are set and have non-null values, so their respective isset()
conditions evaluate to true
, and their values are displayed. The $city
variable is null
, so its isset()
condition evaluates to false
, and the “City is not set.” message is displayed.
It’s important to use isset()
to avoid potential “Undefined variable” notices when trying to access variables that may not have been declared or initialized. Additionally, using isset()
can help you handle form data or user inputs more gracefully, as you can check if specific form fields have been submitted before using them in your PHP code.
Remember that isset()
is not the same as checking for a non-empty value. For example, isset($var)
will return false
if $var
is explicitly set to null
, while empty($var)
would return true
in that case. So, consider using isset()
and empty()
based on your specific use case and the behavior you want to achieve.