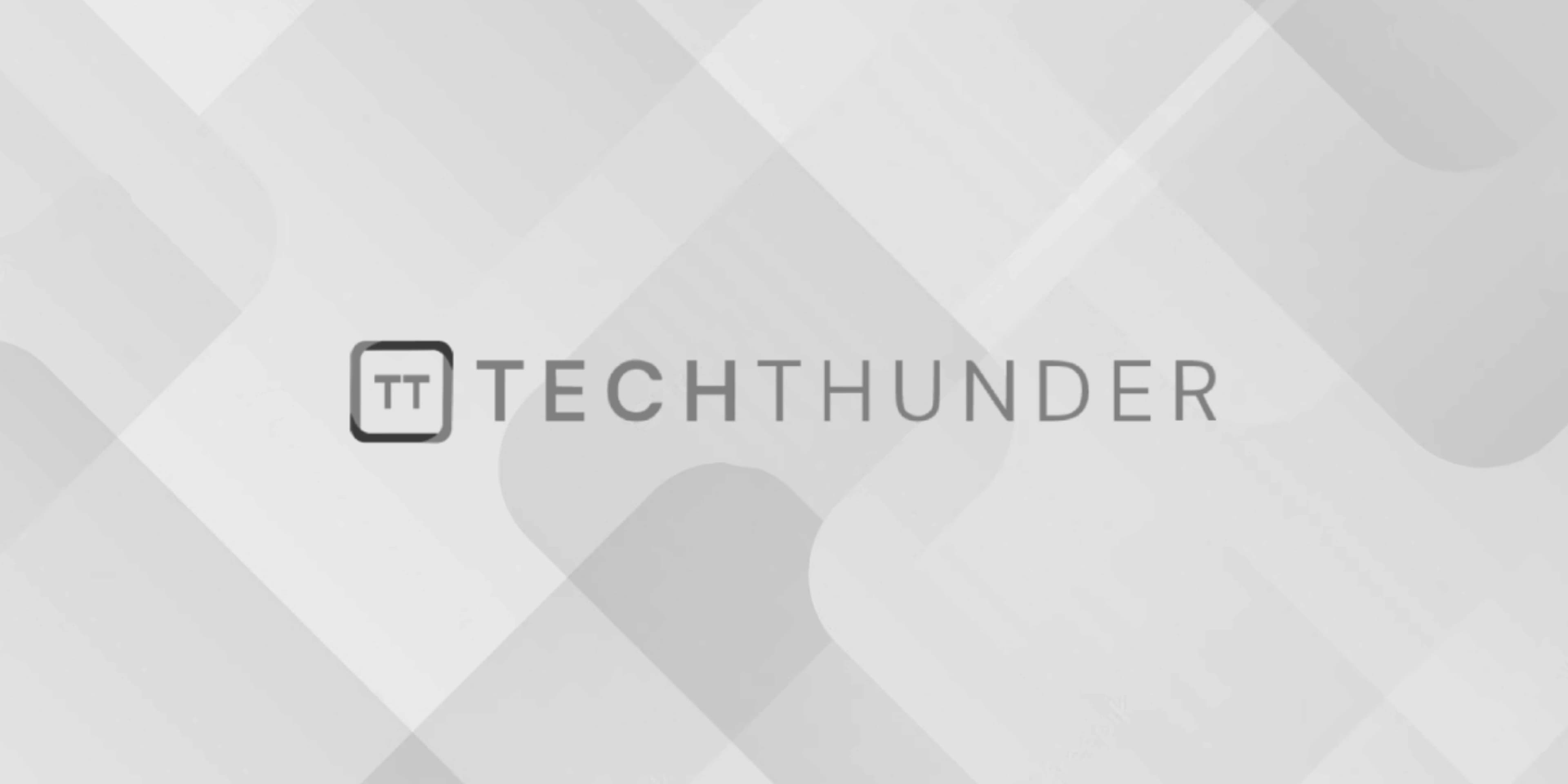
Add Edit Delete Table Row in JQuery
To implement Add, Edit, and Delete functionality for table rows using jQuery, you can follow these steps:
Step 1: Set up your HTML table structure.
Step 2: Implement Add Row functionality using jQuery.
Step 3: Implement Edit Row functionality using jQuery.
Step 4: Implement Delete Row functionality using jQuery.
Here’s an example of how to achieve this:
Step 1: HTML structure
<!DOCTYPE html>
<html>
<head>
<title>Add Edit Delete Table Row using jQuery</title>
</head>
<body>
<table id="myTable">
<thead>
<tr>
<th>Name</th>
<th>Email</th>
<th>Actions</th>
</tr>
</thead>
<tbody>
<!-- Table rows will be dynamically added here -->
</tbody>
</table>
<button id="addButton">Add Row</button>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script src="script.js"></script>
</body>
</html>
Step 2: JavaScript (script.js)
$(document).ready(function() {
// Add Row functionality
$('#addButton').click(function() {
var newRow = '<tr><td contenteditable="true"></td><td contenteditable="true"></td><td><button class="editButton">Edit</button><button class="deleteButton">Delete</button></td></tr>';
$('#myTable tbody').append(newRow);
});
// Edit Row functionality
$(document).on('click', '.editButton', function() {
var row = $(this).closest('tr');
var name = row.find('td:eq(0)').text();
var email = row.find('td:eq(1)').text();
row.find('td:eq(0)').html('<input type="text" value="' + name + '">');
row.find('td:eq(1)').html('<input type="text" value="' + email + '">');
$(this).toggleClass('editButton updateButton').text('Update');
});
$(document).on('click', '.updateButton', function() {
var row = $(this).closest('tr');
var name = row.find('input:eq(0)').val();
var email = row.find('input:eq(1)').val();
row.find('td:eq(0)').text(name);
row.find('td:eq(1)').text(email);
$(this).toggleClass('editButton updateButton').text('Edit');
});
// Delete Row functionality
$(document).on('click', '.deleteButton', function() {
$(this).closest('tr').remove();
});
});
In this example, the JavaScript code handles the Add Row, Edit Row, and Delete Row functionalities using jQuery event delegation. The Add Row functionality appends a new row to the table when the “Add Row” button is clicked. The Edit Row functionality allows users to edit the table cells by converting them into editable input fields when the “Edit” button is clicked. The “Edit” button changes to an “Update” button to save the changes. The Delete Row functionality removes the row when the “Delete” button is clicked.
This example uses contenteditable attribute to make the table cells editable. If you prefer using modal dialogs or other methods for editing data, you can customize the code accordingly.
With this implementation, you have an Add Edit Delete table row functionality using jQuery. You can further enhance this functionality by adding data validation, confirmation dialogs, or saving data to the server using Ajax.