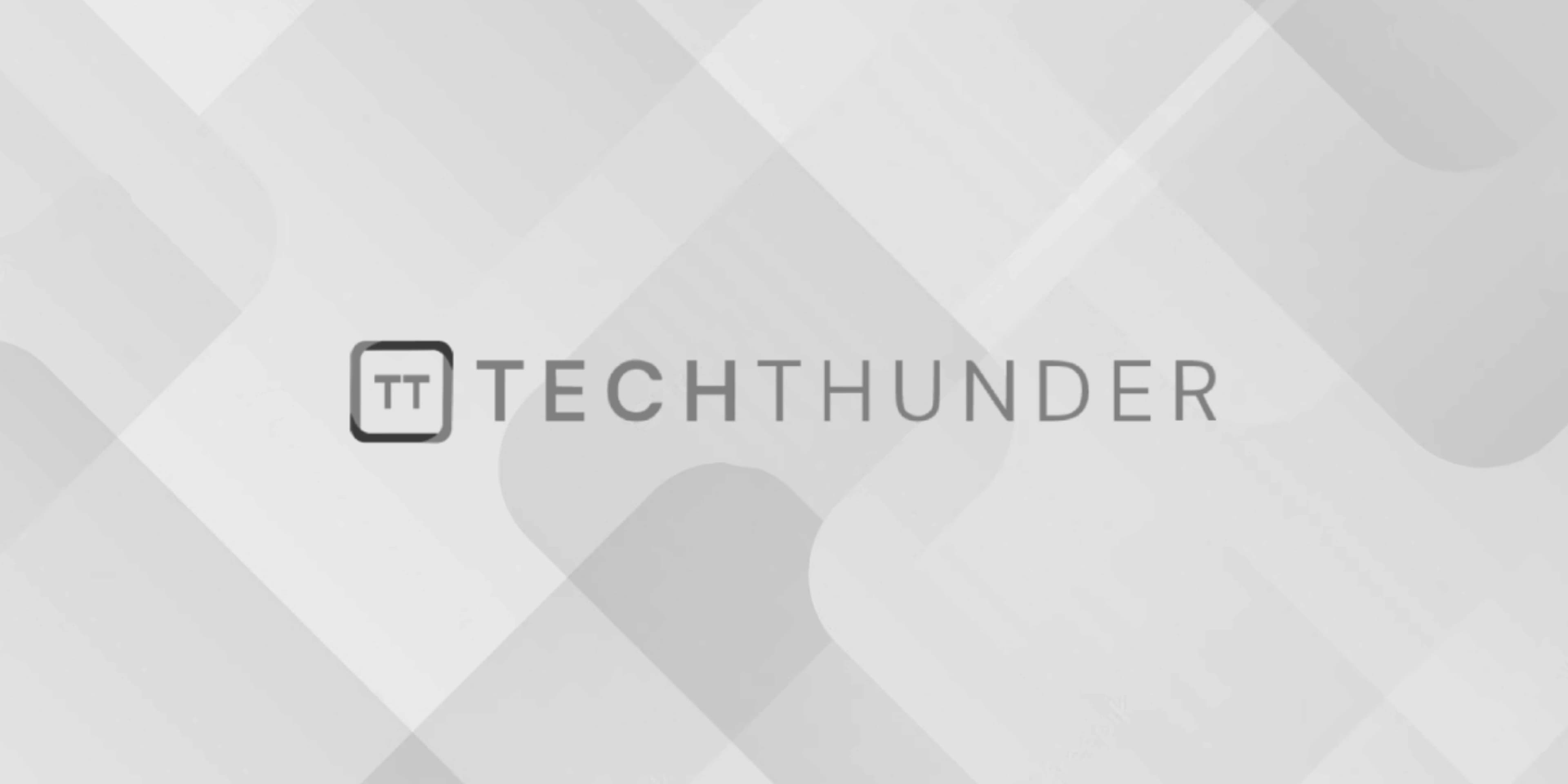
jQuery focusout() method
The focusout()
method in jQuery is used to attach an event handler function to the “focusout” event on one or more elements. The “focusout” event occurs when an element loses focus, typically triggered by moving focus to another element, clicking outside the element, or pressing the “Tab” key to navigate away from the element.
Here’s the basic syntax of the focusout()
method:
$(selector).focusout(handler)
Parameters:
selector
: The selector for the elements to attach the event handler to.handler
: The function to be executed when the “focusout” event is triggered on the selected elements.
Return Value:
The focusout()
method returns the selected jQuery object to support method chaining.
Example:
<!DOCTYPE html>
<html>
<head>
<title>jQuery focusout() Method Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<input type="text" id="inputField" placeholder="Enter your name">
<p id="message"></p>
<script>
$(document).ready(function() {
$("#inputField").focusout(function() {
var inputValue = $(this).val();
$("#message").text("You entered: " + inputValue);
});
});
</script>
</body>
</html>
In this example, we have an input field with the ID “inputField” and a paragraph element with the ID “message.” We use the focusout()
method to attach an event handler function to the “focusout” event of the input field. When the input field loses focus (i.e., the user clicks outside the input field or tabs away from it), the event handler function is executed. The function retrieves the value entered in the input field and displays it in the paragraph element with the ID “message.”
Note that the focusout()
method is similar to the blur()
method in jQuery. Both methods can be used to handle the “focusout” event, but there is a subtle difference between them. The focusout()
method bubbles the event, meaning that if an element loses focus, the “focusout” event will also be triggered for its ancestor elements. In contrast, the blur()
method does not bubble the event.
In most cases, you can use either focusout()
or blur()
depending on your specific needs. If you need the event to bubble, you can use focusout()
. If you don’t need bubbling and want to handle the event directly on the element that loses focus, you can use blur()
.