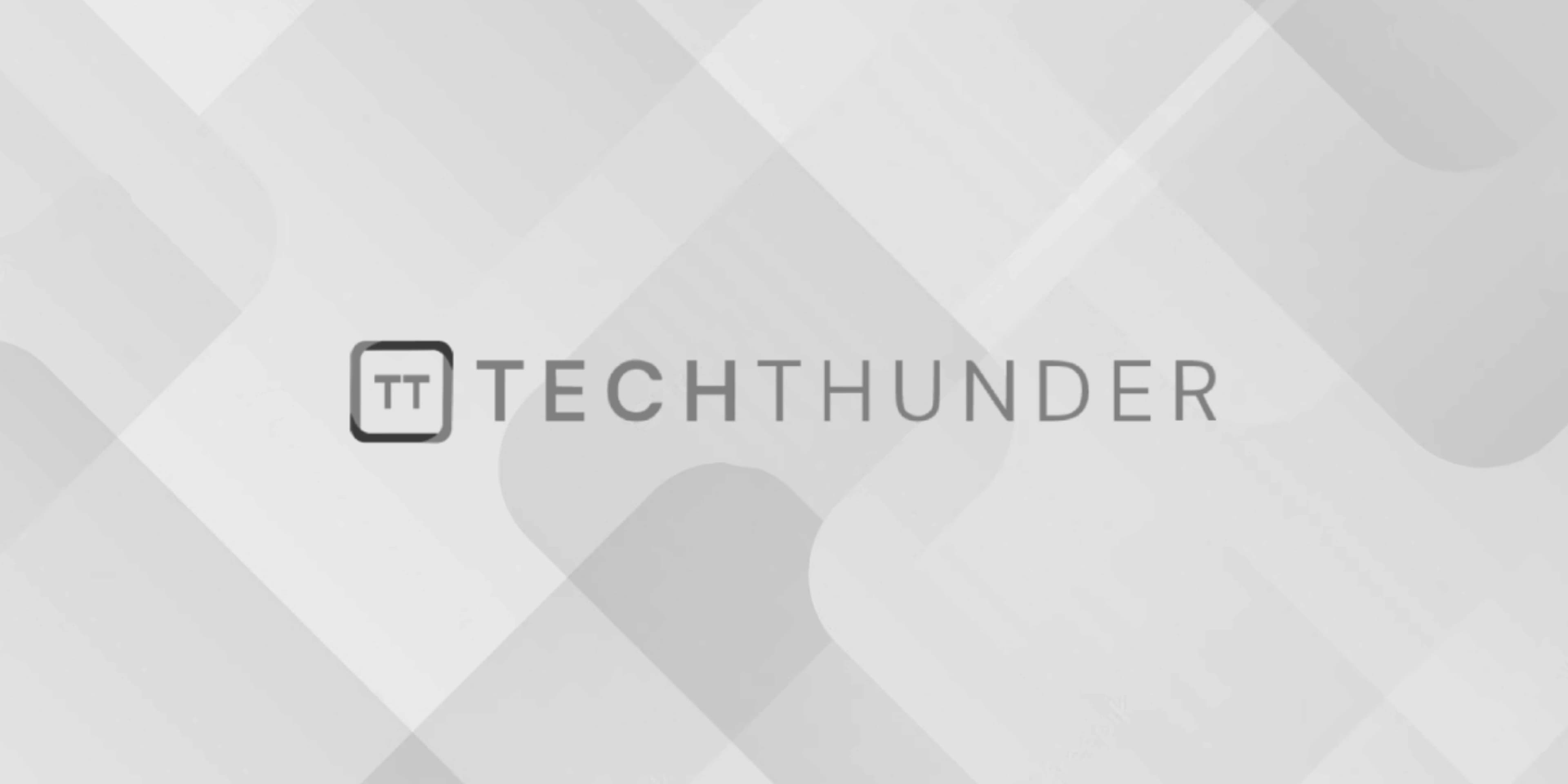
jQuery ajaxSend() method
The jQuery ajaxSend()
method is an event handler that is triggered just before an Ajax request is sent. It allows you to attach a function that will be executed whenever an Ajax request is about to be sent to the server. This event provides an opportunity to modify the request or perform any necessary setup before it is sent.
Here’s the basic syntax of the ajaxSend()
method:
$(document).ajaxSend(function(event, jqxhr, settings) {
// Code to be executed before the Ajax request is sent
});
Parameters:
event
: The jQuery event object.jqxhr
: The jQuery XHR (XMLHttpRequest) object representing the Ajax request.settings
: The settings object used for the Ajax request (e.g., URL, type, data, etc.).
Example:
<!DOCTYPE html>
<html>
<head>
<title>jQuery ajaxSend() Method Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<button id="sendRequest">Send Ajax Request</button>
<script>
$(document).ready(function() {
// Attach ajaxSend event handler
$(document).ajaxSend(function(event, jqxhr, settings) {
console.log("Ajax request is about to be sent.");
});
// Click event handler for the button
$("#sendRequest").on("click", function() {
// Simulate an Ajax request
$.ajax({
url: "https://api.example.com/data",
method: "GET",
success: function(data) {
console.log("Ajax request completed successfully.");
},
error: function() {
console.log("Ajax request failed.");
}
});
});
});
</script>
</body>
</html>
In this example, we have a button with the ID “sendRequest.” When the button is clicked, an Ajax request is made using $.ajax()
. Before the request is sent, the ajaxSend()
event handler is triggered, and the message “Ajax request is about to be sent.” is logged to the console.
Note: The ajaxSend()
event is triggered for every Ajax request made using jQuery. If you need to perform setup actions for specific Ajax requests, you can check the settings
object inside the event handler to determine the request’s URL, method, or other properties.
The ajaxSend()
method is useful when you want to perform global actions or setup tasks for all Ajax requests on a page. It is commonly used for tasks like showing loading spinners, modifying request headers, or performing other preparations before the actual request is sent to the server.