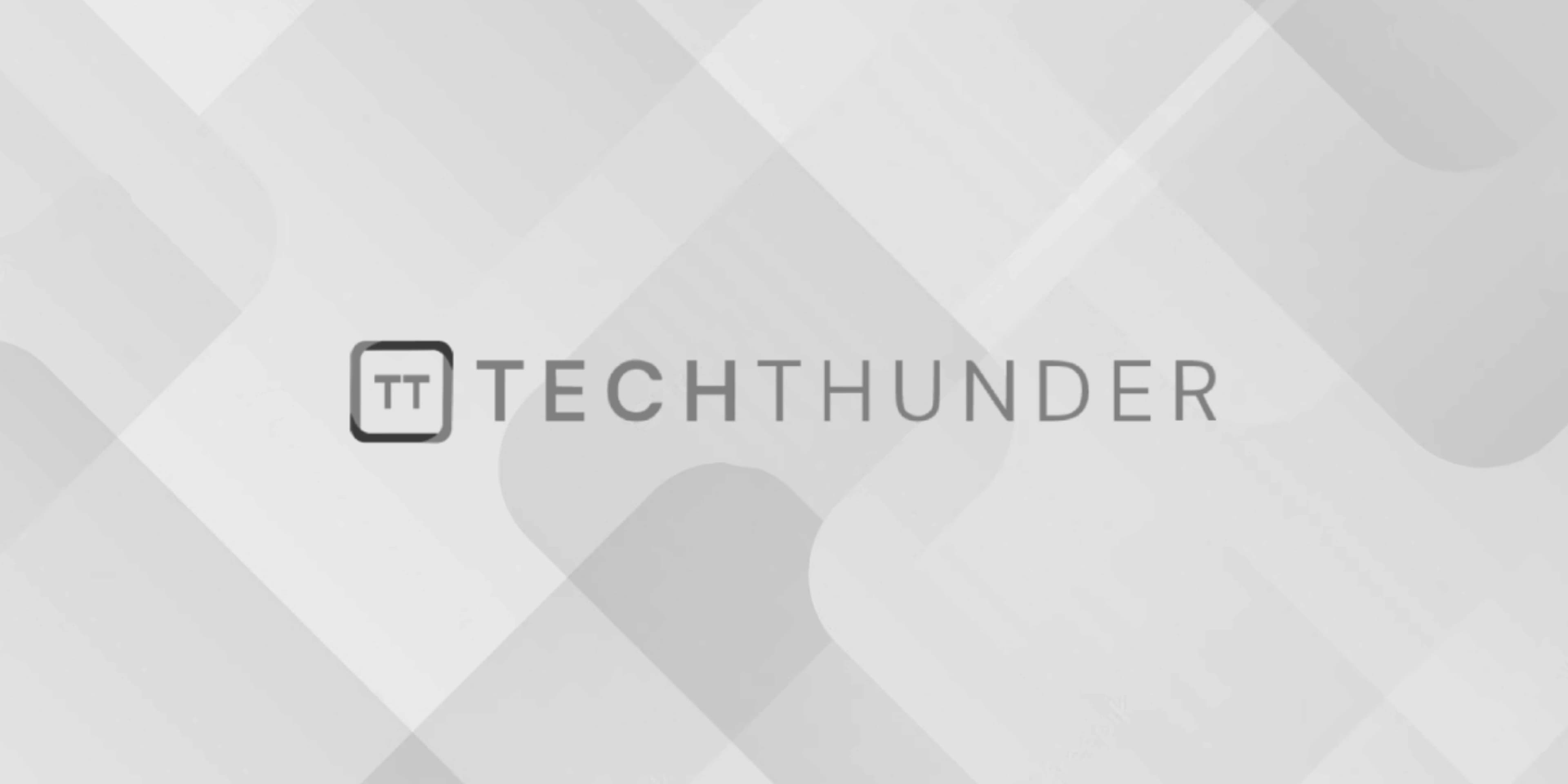
Jquery Countdown Timer
Creating a countdown timer using jQuery is a common task for various web applications, such as event countdowns or limited-time offers. Below is a simple example of how to create a countdown timer using jQuery:
HTML:
<!DOCTYPE html>
<html>
<head>
<title>Countdown Timer</title>
<!-- Include jQuery library -->
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<div id="countdown"></div>
<script src="script.js"></script>
</body>
</html>
JavaScript (script.js):
$(document).ready(function() {
// Set the target date and time for the countdown (YYYY-MM-DD HH:mm:ss format)
var targetDate = '2023-12-31 23:59:59';
// Update the countdown every second
var timer = setInterval(function() {
// Get the current date and time
var now = new Date().getTime();
// Get the target date and time
var target = new Date(targetDate).getTime();
// Calculate the remaining time
var remainingTime = target - now;
// Calculate days, hours, minutes, and seconds
var days = Math.floor(remainingTime / (1000 * 60 * 60 * 24));
var hours = Math.floor((remainingTime % (1000 * 60 * 60 * 24)) / (1000 * 60 * 60));
var minutes = Math.floor((remainingTime % (1000 * 60 * 60)) / (1000 * 60));
var seconds = Math.floor((remainingTime % (1000 * 60)) / 1000);
// Display the countdown
document.getElementById('countdown').innerHTML =
'Countdown: ' +
days + ' days, ' +
hours + ' hours, ' +
minutes + ' minutes, ' +
seconds + ' seconds';
// If the countdown is over, display a message
if (remainingTime <= 0) {
clearInterval(timer);
document.getElementById('countdown').innerHTML = 'Countdown is over!';
}
}, 1000); // Update the countdown every second
});
In this example, we use jQuery to set up the countdown timer. We specify the target date and time in the targetDate
variable, and the countdown will calculate the remaining time and update the display every second using setInterval
.
Replace the targetDate
variable with the specific date and time you want to countdown to in the format 'YYYY-MM-DD HH:mm:ss'
.
This is a basic example, and you can customize the countdown timer’s appearance and behavior as per your project requirements, such as displaying the countdown in a different format or adding additional styling. Also, consider handling time zone differences if your countdown is intended for a specific location or event.