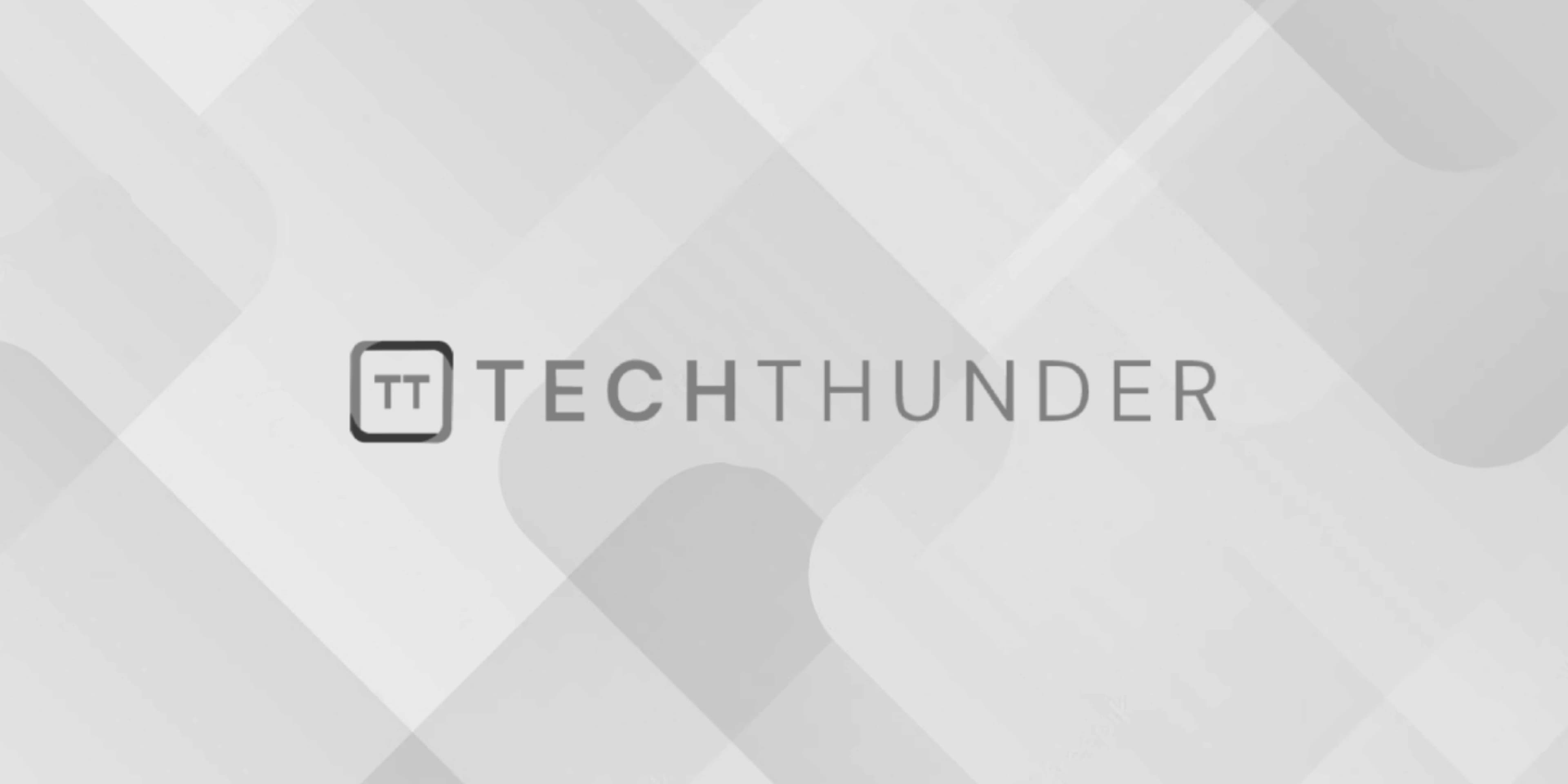
jQuery last() method
The jQuery last()
method is used to select the last element in a set of matched elements. It is similar to the :last
selector in jQuery, but it can be used on jQuery objects as well.
Here’s the basic syntax of the last()
method:
$(selector).last()
Return Value:
The last()
method returns a jQuery object containing the last element from the set of matched elements.
Example:
<!DOCTYPE html>
<html>
<head>
<title>jQuery last() Method Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<ul>
<li>Item 1</li>
<li>Item 2</li>
<li>Item 3</li>
<li>Item 4</li>
</ul>
<script>
$(document).ready(function() {
// Select the last <li> element in the <ul>
var lastItem = $("ul li").last();
// Add a class to the last <li> element for styling
lastItem.addClass("last-item");
});
</script>
<style>
.last-item {
font-weight: bold;
}
</style>
</body>
</html>
In this example, we have an unordered list (<ul>
) with four list items (<li>
). We use the last()
method to select the last <li>
element in the list. We then add a class called “last-item” to the last <li>
element to apply bold font weight to it.
When you run the code, you will see that the last list item “Item 4” is displayed in bold font weight due to the “last-item” class being applied to it.
The last()
method is useful when you want to target the last element in a selection or perform specific operations on the last element in a set of matched elements. It is commonly used in combination with other jQuery methods to manipulate or style the last element in a group.