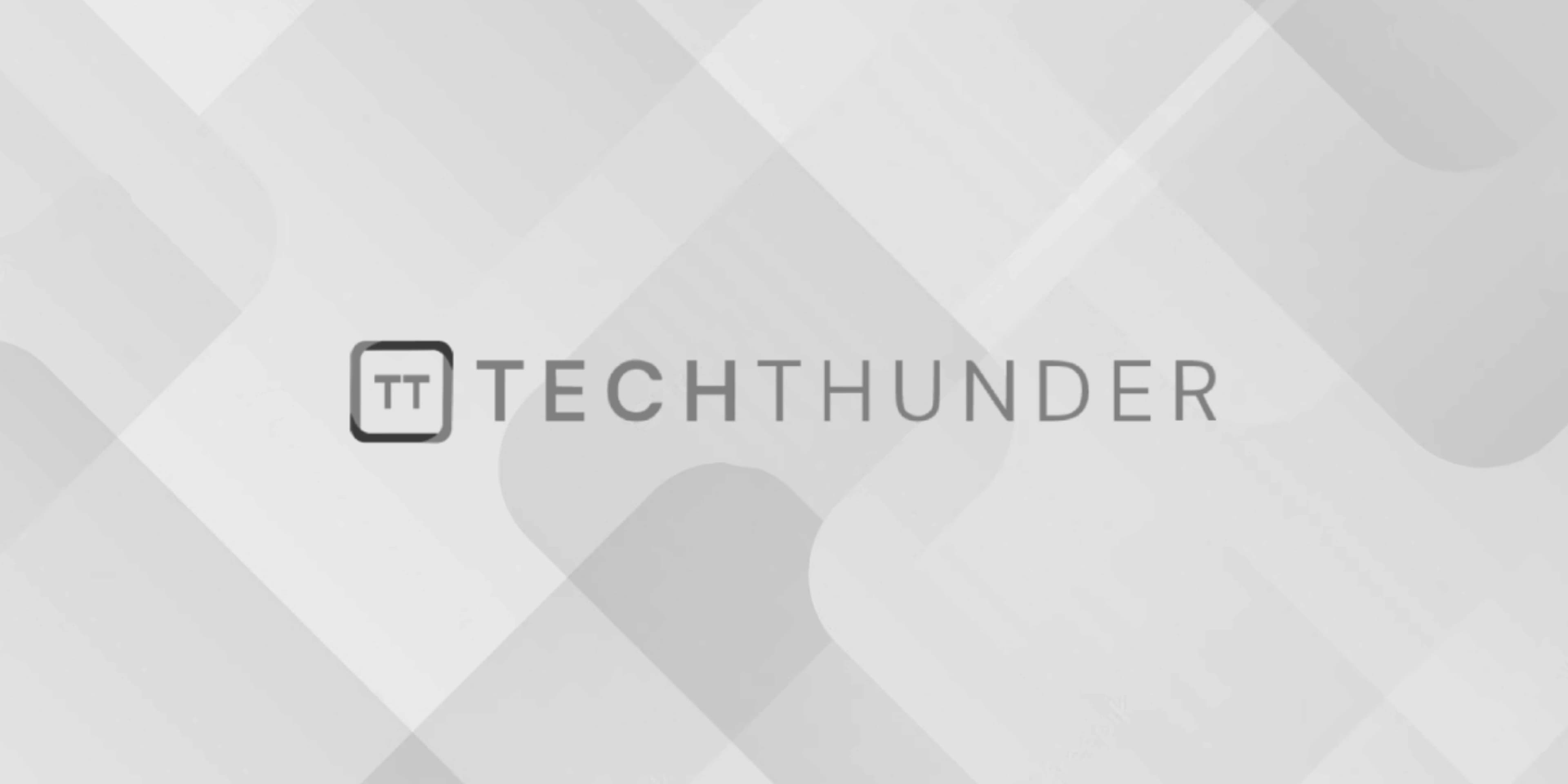
jQuery not() method
The jQuery not()
method is used to filter out elements from a set of matched elements based on a specified condition. It allows you to exclude elements that match a particular selector or filter function.
Here’s the basic syntax of the not()
method:
$(selector).not(filter)
Parameters:
selector
: A selector string or jQuery object representing the elements to be filtered.filter
: A selector string, element, function, or another jQuery object representing the elements that should be excluded from the matched set.
Example:
<!DOCTYPE html>
<html>
<head>
<title>jQuery not() Method Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<ul>
<li class="item">Item 1</li>
<li class="item">Item 2</li>
<li class="item special">Item 3</li>
<li class="item">Item 4</li>
<li class="item">Item 5</li>
</ul>
<script>
$(document).ready(function() {
// Select all <li> elements with class "item", but not the one with class "special"
$('ul li.item').not('.special').addClass('highlight');
});
</script>
<style>
.highlight {
background-color: yellow;
}
</style>
</body>
</html>
In this example, we have an unordered list (<ul>
) with five list items (<li>
). We use the not()
method to select all list items with class “item” but exclude the one with class “special”. We then apply the highlight
class to the selected list items. As a result, all list items with class “item” except the one with class “special” will have a yellow background.
The not()
method is useful for refining a set of matched elements by removing specific elements that do not meet certain criteria. It allows you to create more targeted selections for manipulation or styling.