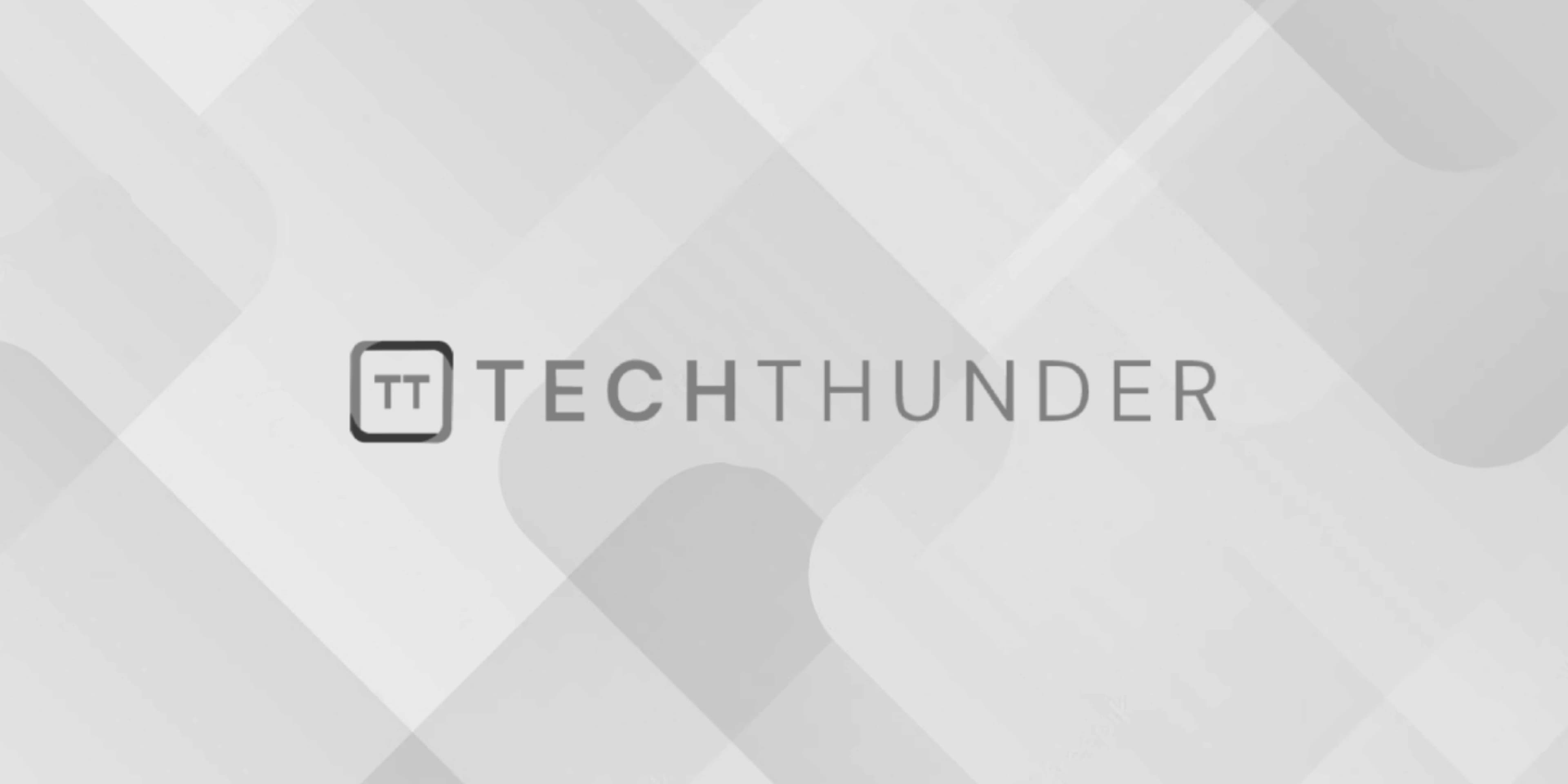
jQuery Example
To create a simple example using jQuery to toggle the visibility of a div element when a button is clicked.
HTML:
<!DOCTYPE html>
<html>
<head>
<title>jQuery Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<style>
#myDiv {
display: none;
background-color: lightblue;
padding: 10px;
margin-top: 10px;
}
</style>
</head>
<body>
<button id="toggleButton">Toggle Content</button>
<div id="myDiv">
<p>This is some hidden content.</p>
</div>
<script>
$(document).ready(function() {
// Attach a click event handler to the button
$('#toggleButton').click(function() {
// Toggle the visibility of the div with a fading animation
$('#myDiv').fadeToggle();
});
});
</script>
</body>
</html>
In this example, we have a button with the ID “toggleButton” and a div with the ID “myDiv” containing some hidden content. The CSS sets the initial display of the “myDiv” to “none”, making it hidden when the page loads.
When the “toggleButton” is clicked, the jQuery code inside the <script>
tag executes. The $(document).ready()
function ensures that the code inside it runs after the page has finished loading.
The $('#toggleButton').click()
function attaches a click event handler to the button. When the button is clicked, the $('#myDiv').fadeToggle()
method is called. This method toggles the visibility of “myDiv” with a fading animation. If the div is hidden, it will fade in and become visible. If the div is visible, it will fade out and become hidden.
The result is that clicking the “Toggle Content” button will toggle the visibility of the “myDiv” element, revealing or hiding the hidden content with a smooth fade-in and fade-out effect.
This is just a simple example to demonstrate the basic usage of jQuery. In real-world applications, jQuery can be used for more complex interactions, animations, and dynamic content manipulation on web pages.