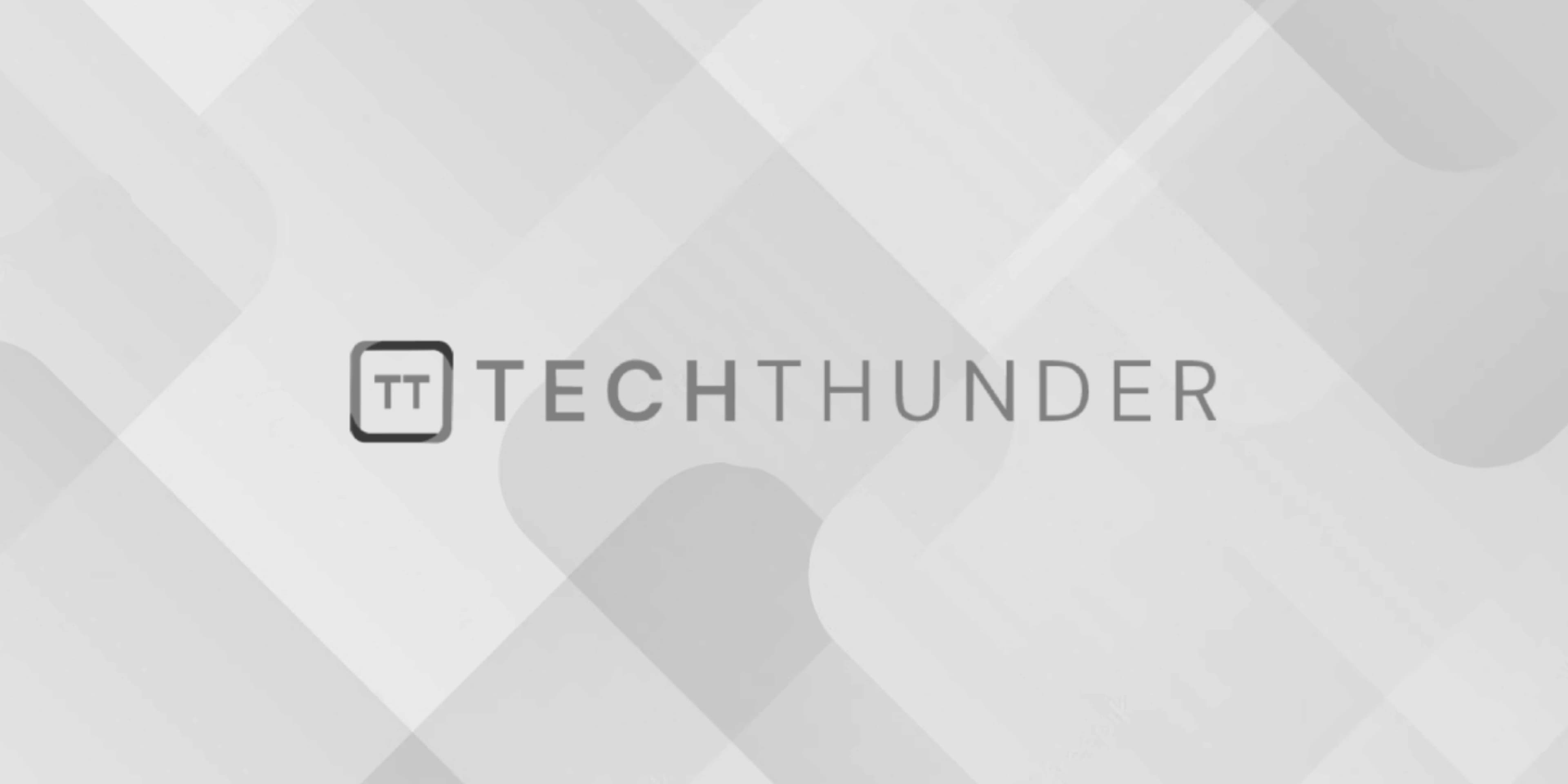
156 views
How to Detect Mobile Device
You can detect a mobile device using JavaScript by checking the user agent string, screen width, or media queries. Here are three common methods to achieve this:
- User Agent String:
You can check the user agent string of the browser to identify whether the device is a mobile device. The user agent string provides information about the browser and the device being used. Here’s a simple example:
if (/Mobi|Android/i.test(navigator.userAgent)) {
// Code for mobile devices
} else {
// Code for non-mobile devices (e.g., desktop)
}
This code checks if the user agent string contains “Mobi” or “Android,” which are often present in the user agent string of mobile devices.
- Screen Width:
You can use JavaScript to check the screen width and determine if the device’s width is below a certain threshold, typically associated with mobile devices.
if (window.innerWidth <= 768) {
// Code for mobile devices (adjust the threshold as needed)
} else {
// Code for non-mobile devices
}
In this example, a screen width of 768 pixels or less is considered a mobile device.
- Media Queries (CSS):
Another approach is to use CSS media queries to apply different styles or execute JavaScript code based on the screen size. You can define CSS rules for mobile and non-mobile devices:
/* Mobile styles (applies to devices with a max width of 768px) */
@media (max-width: 768px) {
/* CSS styles for mobile devices */
}
/* Non-mobile styles (applies to devices with a width greater than 768px) */
@media (min-width: 769px) {
/* CSS styles for non-mobile devices */
}
You can also use JavaScript to check if a specific CSS rule is applied, which would indicate the device type.
Keep in mind that the user agent string can be manipulated, and using media queries is generally a more reliable method for handling responsive design. However, depending on your use case, any of these methods may be appropriate.