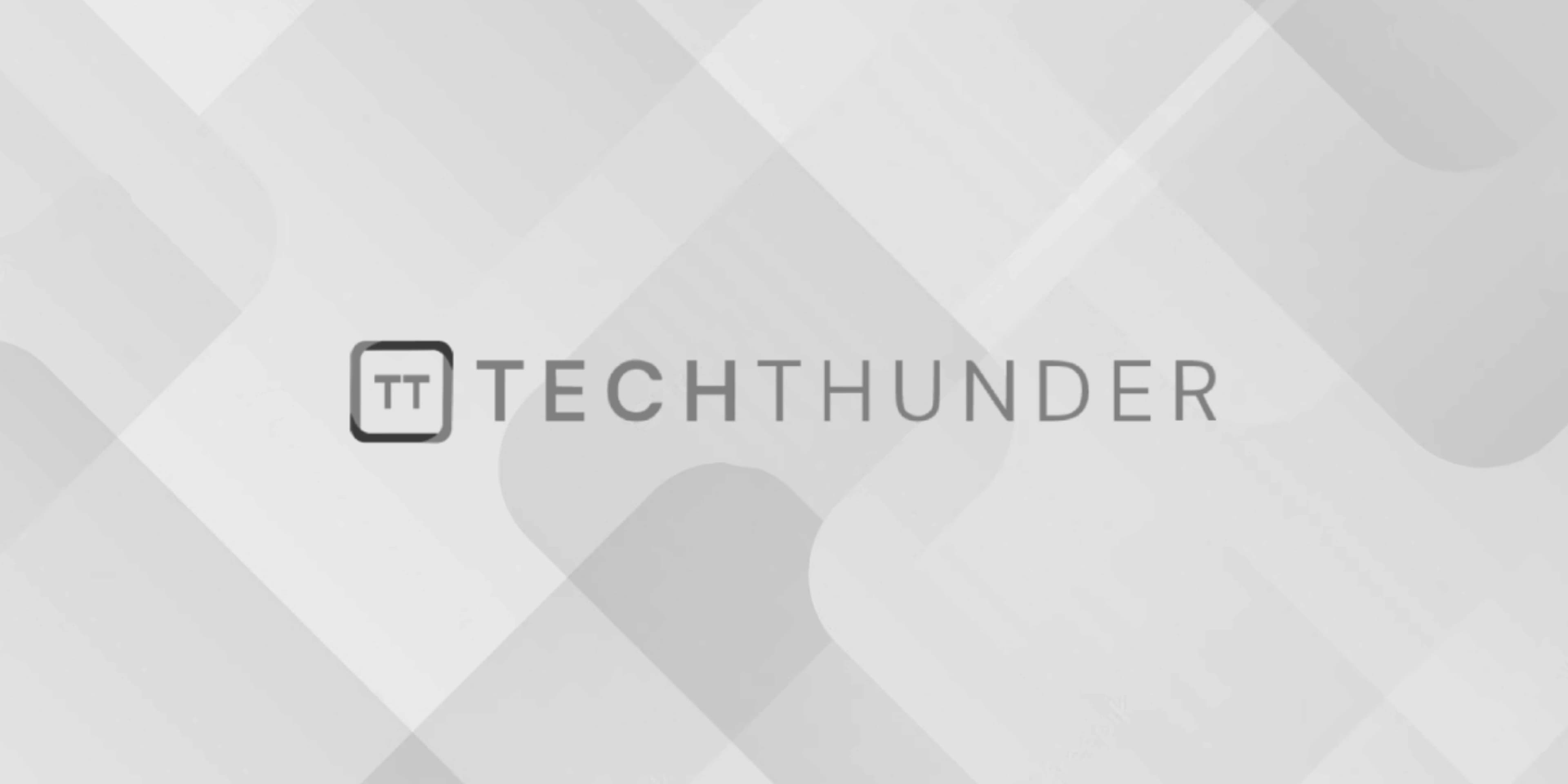
jQuery dequeue() method
The dequeue()
method in jQuery is used to remove and execute the next function from the animation queue of elements selected by the jQuery object. The animation queue is a series of animations that are waiting to be executed on the selected elements.
Here’s the basic syntax of the dequeue()
method:
$(selector).dequeue([queueName])
Parameters:
selector
: The selector for the elements to dequeue the next function.queueName
(optional): A string representing the name of the queue from which the next function should be dequeued. If not specified, the method dequeues the next function from the default “fx” queue, which is the standard animation queue.
Return Value:
The dequeue()
method returns the same jQuery object to support method chaining.
Example:
<!DOCTYPE html>
<html>
<head>
<title>jQuery dequeue() Method Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<style>
div {
width: 100px;
height: 100px;
background-color: red;
margin: 10px;
}
</style>
</head>
<body>
<div></div>
<button id="startAnimation">Start Animation</button>
<button id="dequeueAnimation">Dequeue Animation</button>
<script>
$(document).ready(function() {
var $box = $("div");
function startAnimation() {
$box.animate({ marginLeft: "200px" }, 2000);
$box.animate({ marginLeft: "0px" }, 2000);
}
function dequeueAnimation() {
// Dequeue the next animation from the queue when the "Dequeue Animation" button is clicked
$box.dequeue();
}
$("#startAnimation").click(function() {
startAnimation();
});
$("#dequeueAnimation").click(function() {
dequeueAnimation();
});
});
</script>
</body>
</html>
In this example, we have a red square (a div
element) and two buttons: “Start Animation” and “Dequeue Animation.” When the “Start Animation” button is clicked, the startAnimation()
function is called, which animates the square to move right and then back to its original position. When the “Dequeue Animation” button is clicked, the dequeueAnimation()
function is called, which uses the dequeue()
method to remove and execute the next animation from the animation queue, effectively stopping any ongoing animation and immediately jumping to the final position.
Please note that the dequeue()
method is useful when you want to control the execution of animations and remove specific animations from the queue. It allows you to control the flow of animations and manage animations dynamically based on certain conditions.
Keep in mind that if you want to remove all animations from the queue, you can use the clearQueue()
method as shown in a previous answer.