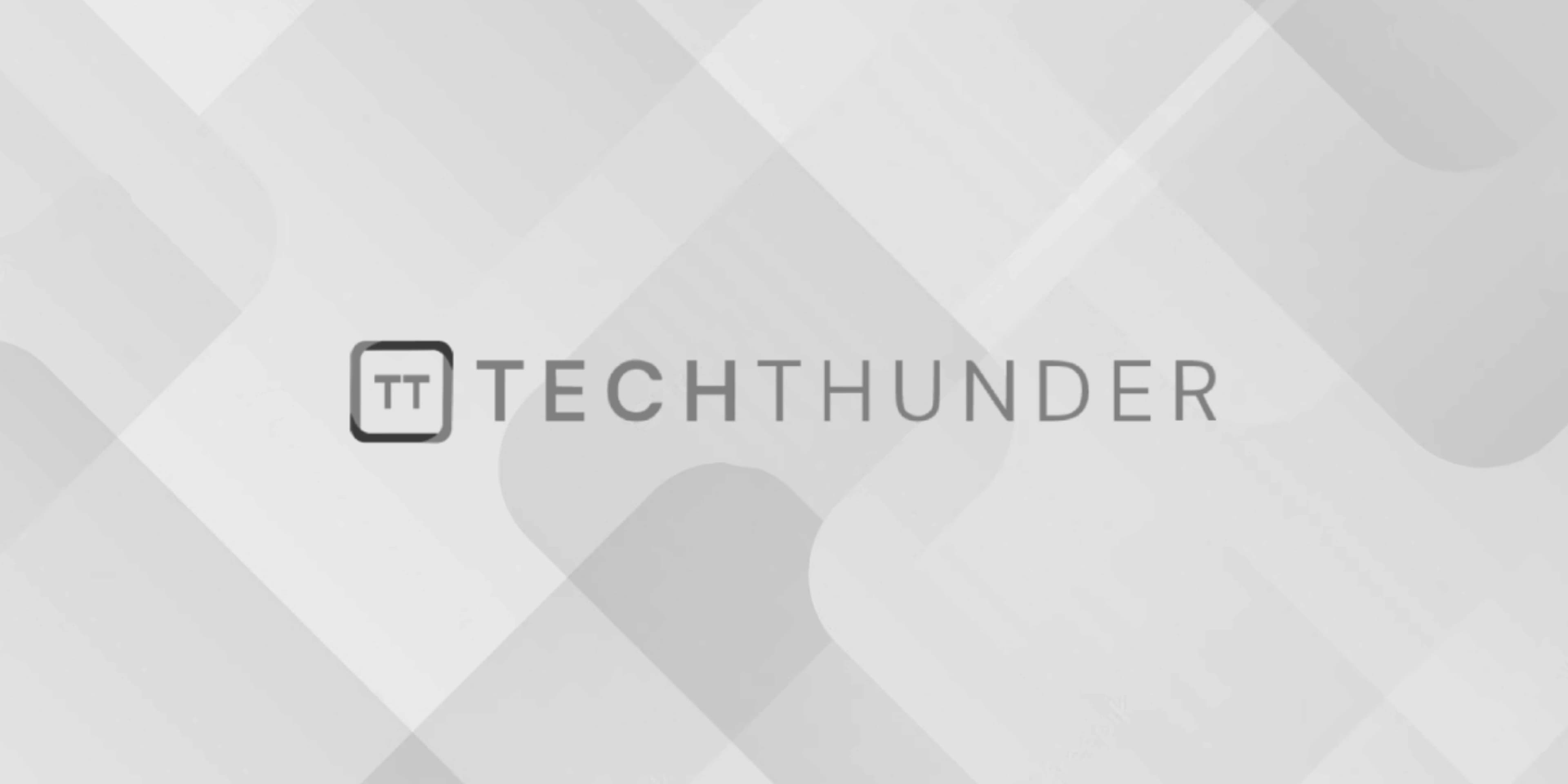
JQuery Typewriter
Creating a typewriter effect using jQuery involves animating the appearance of text one character at a time, simulating the effect of typing. Here’s a simple example of how to implement a typewriter effect using jQuery:
HTML:
<!DOCTYPE html>
<html>
<head>
<title>Typewriter Effect using jQuery</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div id="typewriter">
<h1 id="text-container"></h1>
</div>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script src="script.js"></script>
</body>
</html>
CSS (styles.css):
body {
font-family: Arial, sans-serif;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
margin: 0;
}
#typewriter {
background-color: #f2f2f2;
padding: 20px;
border-radius: 5px;
}
#text-container {
white-space: pre;
}
JavaScript (script.js):
$(document).ready(function() {
var text = "Welcome to Typewriter Effect using jQuery!";
var index = 0;
var speed = 50; // Speed of typewriter effect (in milliseconds)
function typeWriter() {
if (index < text.length) {
var char = text.charAt(index);
$("#text-container").append(char);
index++;
setTimeout(typeWriter, speed);
}
}
// Start the typewriter effect when the page loads
typeWriter();
});
In this example, we have a <div>
with the ID typewriter
, and within it, an <h1>
element with the ID text-container
. The text that we want to display with the typewriter effect is stored in the text
variable.
In the JavaScript file (script.js
), we create a function called typeWriter
to implement the typewriter effect. The function uses jQuery to append one character at a time to the text-container
element with a certain time delay specified by the speed
variable.
When the page loads, we call the typeWriter
function to start the typewriter effect and display the text “Welcome to Typewriter Effect using jQuery!” with the typewriter animation.
You can customize the text
variable to display any text you want and adjust the speed
variable to control the speed of the typewriter effect.
Remember to adjust the CSS (styles.css) to style the typewriter container and text as per your design requirements.
That’s it! With this code, you should have a basic typewriter effect using jQuery.