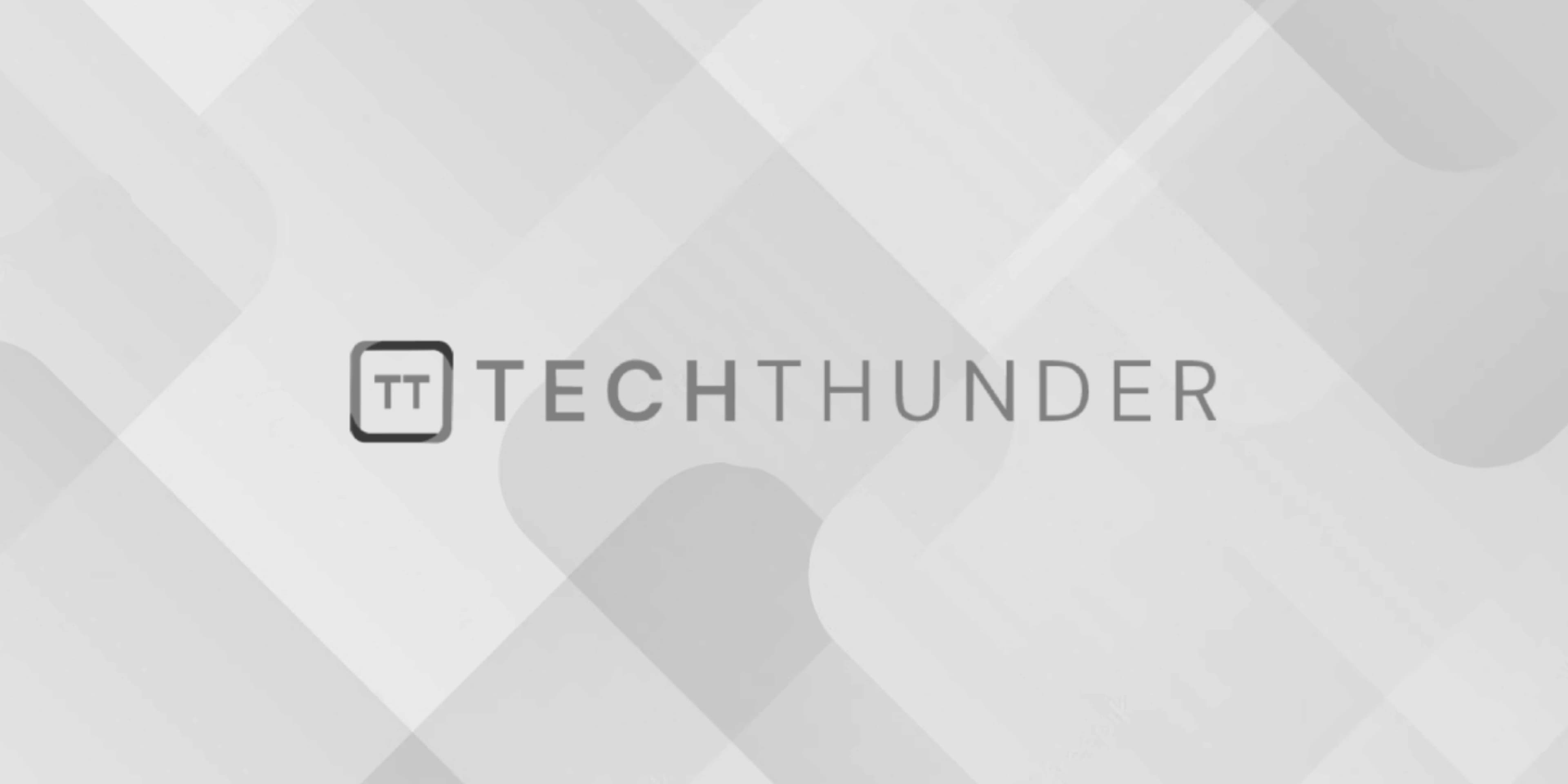
jQuery Page Flip
Creating a page flip effect using jQuery involves simulating the turning of a page in a book or magazine when a user interacts with a container element, such as clicking or dragging. While there is no built-in page flip functionality in jQuery, you can achieve this effect by using CSS3 transitions and transforms along with jQuery for handling user interactions.
Below is a basic example of how to create a page flip effect using jQuery:
HTML:
<!DOCTYPE html>
<html>
<head>
<title>Page Flip Effect</title>
<style>
body {
margin: 0;
padding: 0;
overflow: hidden;
font-family: Arial, sans-serif;
}
.book {
position: relative;
width: 400px;
height: 200px;
margin: 50px auto;
perspective: 800px;
}
.page {
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
background-color: #f0f0f0;
box-shadow: 0 4px 8px rgba(0, 0, 0, 0.1);
transform-origin: left center;
transform-style: preserve-3d;
backface-visibility: hidden;
transition: transform 0.5s ease;
}
.page:nth-child(2n) {
background-color: #e0e0e0;
}
.page-content {
padding: 20px;
box-sizing: border-box;
}
.page.flip {
transform: rotateY(-180deg);
z-index: -1;
}
</style>
</head>
<body>
<div class="book">
<div class="page">
<div class="page-content">
<!-- Content for page 1 goes here -->
<h1>Page 1</h1>
<p>This is the content of page 1.</p>
</div>
</div>
<div class="page">
<div class="page-content">
<!-- Content for page 2 goes here -->
<h1>Page 2</h1>
<p>This is the content of page 2.</p>
</div>
</div>
</div>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script src="script.js"></script>
</body>
</html>
JavaScript (script.js):
$(document).ready(function() {
$('.book').on('click', function() {
// Toggle the "flip" class on the pages to trigger the page flip effect
$('.page').toggleClass('flip');
});
});
In this example, we create a simple page flip effect using CSS3 transitions and transforms. Each page is represented by a .page
element inside a .book
container. The transform: rotateY(-180deg);
CSS property is used to create the flipping effect when the .flip
class is toggled.
When the user clicks anywhere inside the .book
container, the JavaScript code toggles the .flip
class on the pages, which triggers the flip animation.
Please note that this is a basic example, and you can enhance the page flip effect by adding more pages, using CSS animations, and implementing additional interactivity, such as flipping pages with a drag gesture or providing navigation buttons. For more complex and realistic page flip animations, you may consider using dedicated libraries and plugins specifically designed for creating book-like page flipping effects in web applications.