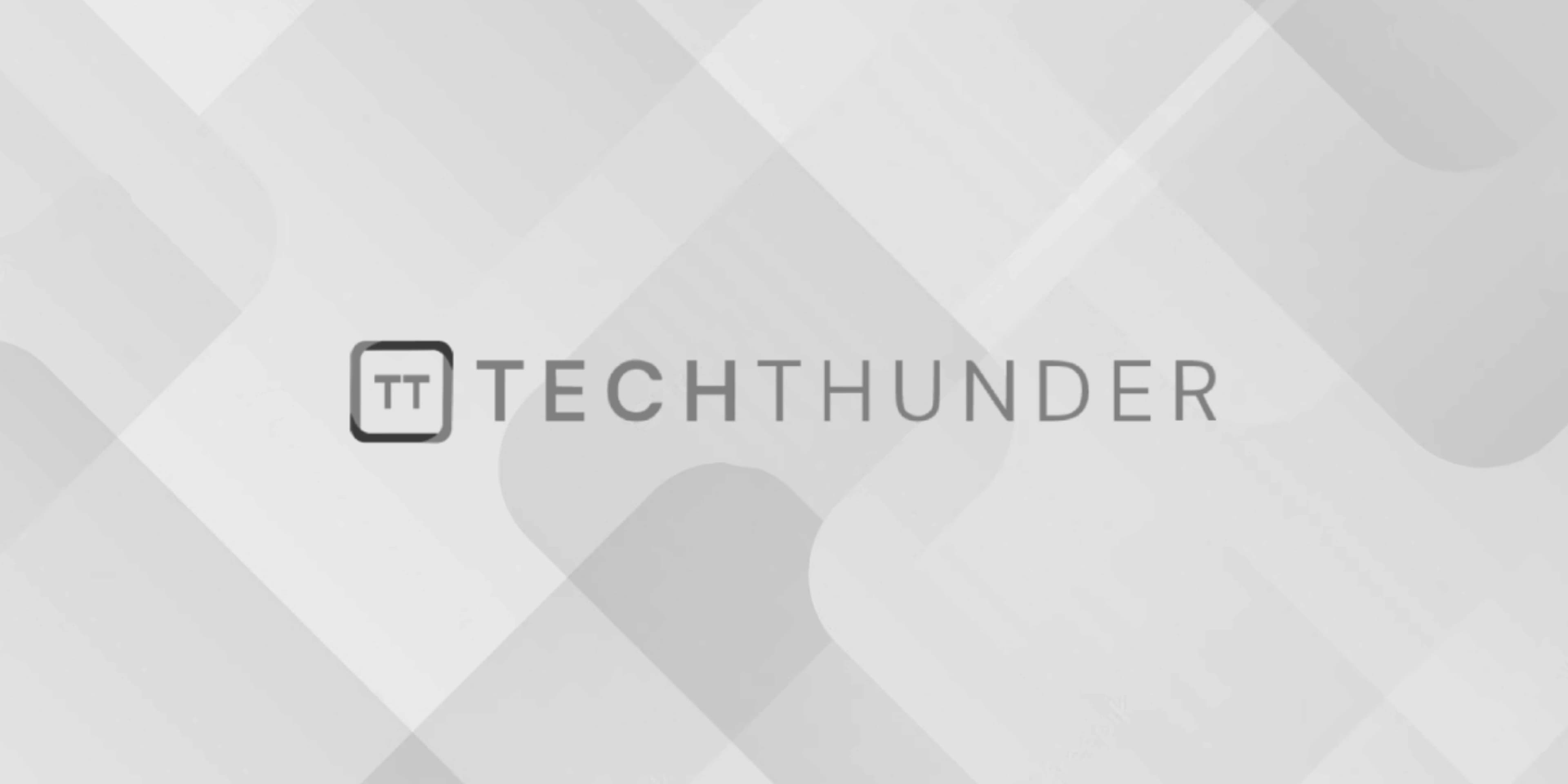
Jquery Flickerplate Plugin
The Flickerplate is a jQuery plugin that provides a simple and customizable image slider or carousel. It allows you to create image galleries with smooth transitions and various options for customization.
Please note that there might have been updates or changes to the Flickerplate plugin since my last update, so I recommend checking the official documentation and repository for the most recent information.
To use the Flickerplate plugin, follow these steps:
Step 1: Include Dependencies
Include the required CSS and JavaScript files for Flickerplate in your HTML file:
<!DOCTYPE html>
<html>
<head>
<title>Flickerplate Example</title>
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/flickity/2.2.2/flickity.min.css">
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/flickity/2.2.2/flickity.pkgd.min.js"></script>
</head>
<body>
<!-- Your content goes here -->
<div class="flicker-slider">
<div class="slide"> <!-- Slide 1 content --> </div>
<div class="slide"> <!-- Slide 2 content --> </div>
<!-- Add more slides as needed -->
</div>
<script src="script.js"></script>
</body>
</html>
Step 2: Initialize the Flickerplate Slider
In your JavaScript file (e.g., script.js
), initialize the Flickerplate slider on the .flicker-slider
container:
$(document).ready(function() {
$('.flicker-slider').flicker();
});
Step 3: Customize the Flickerplate Options (Optional)
Flickerplate provides various options for customization, such as auto-play, navigation buttons, animation speed, and more. You can pass an options object to the flicker()
function to configure the slider according to your needs. Below is an example of customizing some options:
$(document).ready(function() {
$('.flicker-slider').flicker({
arrows: true, // Show navigation arrows
auto_flick: true, // Auto-play the slider
auto_flick_delay: 5000, // Auto-play delay in milliseconds
flick_animation: 'transform-slide', // Animation style ('transform-slide', 'transition-slide', 'jquery-slide', etc.)
flick_position: 0, // Starting slide index
flick_duration: 750, // Animation duration in milliseconds
flick_direction: 'forward', // Direction of auto-flick ('forward' or 'backward')
});
});
These are just a few of the available options. You can explore the Flickerplate documentation for a complete list of options and their descriptions.
Step 4: Style the Slider (Optional)
You can add custom CSS to style the slider and its slides according to your design preferences.
Please note that Flickerplate is just one of many jQuery plugins available for creating image sliders and carousels. There are other alternatives with different features and styles that you can explore based on your specific requirements.
Always ensure to use plugins from reputable sources and check their documentation for usage instructions and supported options. Additionally, consider browser compatibility and performance optimizations when using any image slider or carousel plugin on your website.