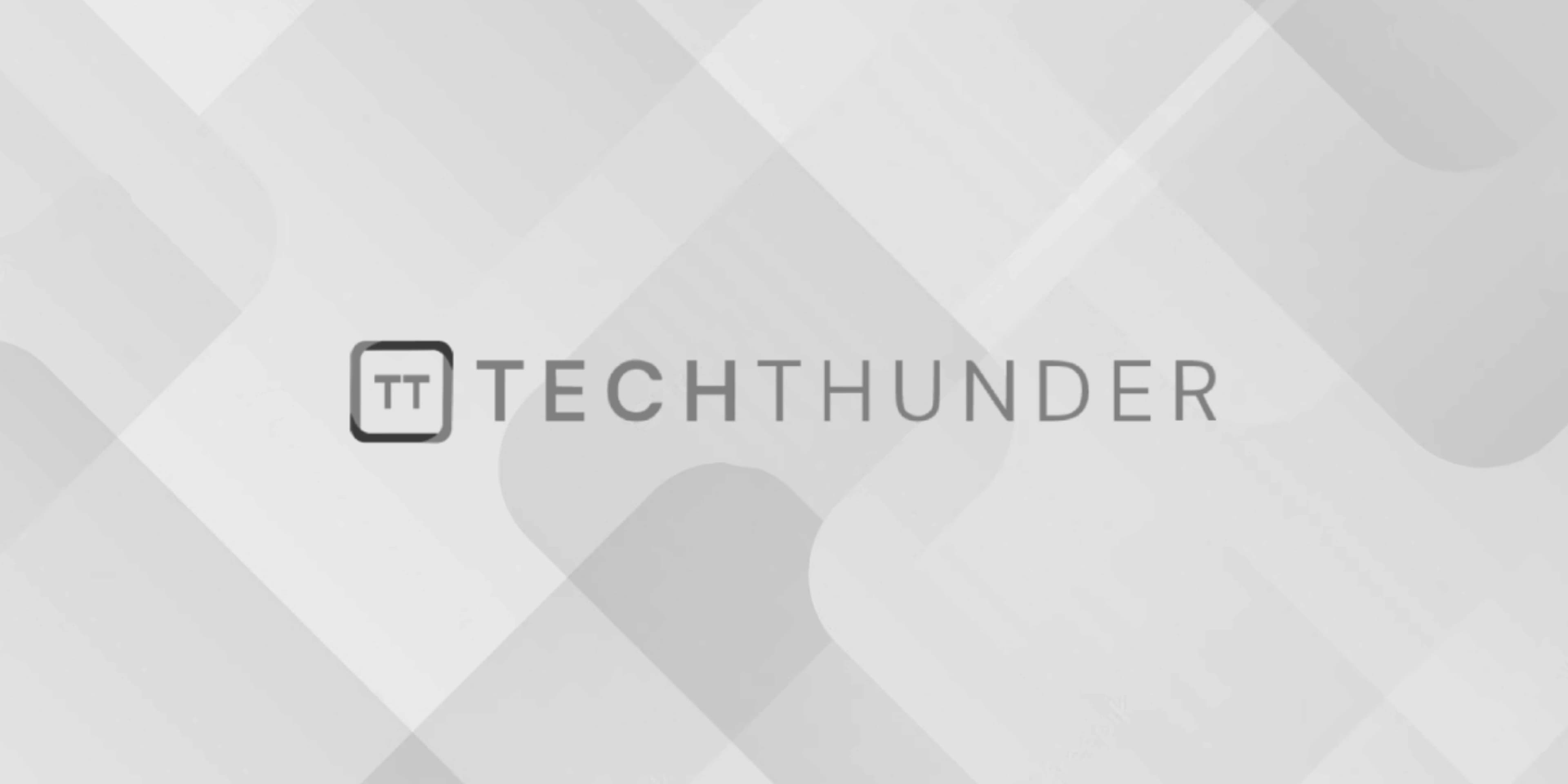
jQuery slice() method
The jQuery.slice()
method is used to extract a subset of elements from a jQuery object and return a new jQuery object containing the selected elements. It allows you to specify a range of elements based on their index positions within the original jQuery object.
Here’s the basic syntax of the slice()
method:
$(selector).slice(startIndex [, endIndex])
Parameters:
selector
: The selector expression used to select the elements.startIndex
: The index position at which to begin extraction. It is a zero-based index, where 0 represents the first element, 1 represents the second element, and so on.endIndex
: Optional. The index position at which to stop extraction. The element at this index will not be included in the result. If not specified, all elements from thestartIndex
to the end of the jQuery object will be included.
Return Value:
The slice()
method returns a new jQuery object containing the selected subset of elements.
Example:
<!DOCTYPE html>
<html>
<head>
<title>jQuery slice() Method Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<div class="item">Item 1</div>
<div class="item">Item 2</div>
<div class="item">Item 3</div>
<div class="item">Item 4</div>
<div class="item">Item 5</div>
<script>
$(document).ready(function() {
// Select all elements with class "item" and slice a subset
var selectedItems = $(".item").slice(1, 4);
// Log the number of selected elements and their content
console.log("Number of selected elements:", selectedItems.length);
selectedItems.each(function(index) {
console.log("Selected Item " + index + ":", $(this).html());
});
});
</script>
</body>
</html>
In this example, we use the slice()
method to select a subset of elements from the jQuery object containing elements with the class “item.” The slice(1, 4)
call will extract elements starting from the second element (index 1) up to, but not including, the fifth element (index 4).
The output of the above example will be:
Number of selected elements: 3
Selected Item 0: Item 2
Selected Item 1: Item 3
Selected Item 2: Item 4
In this output, we see that the slice()
method successfully extracted a subset of elements from the original jQuery object, and the new jQuery object selectedItems
contains the selected elements.
The slice()
method is useful when you need to work with a specific range of elements in a jQuery object. It allows you to select a continuous subset of elements based on their index positions, making it easier to manipulate or perform operations on specific sections of the jQuery object.