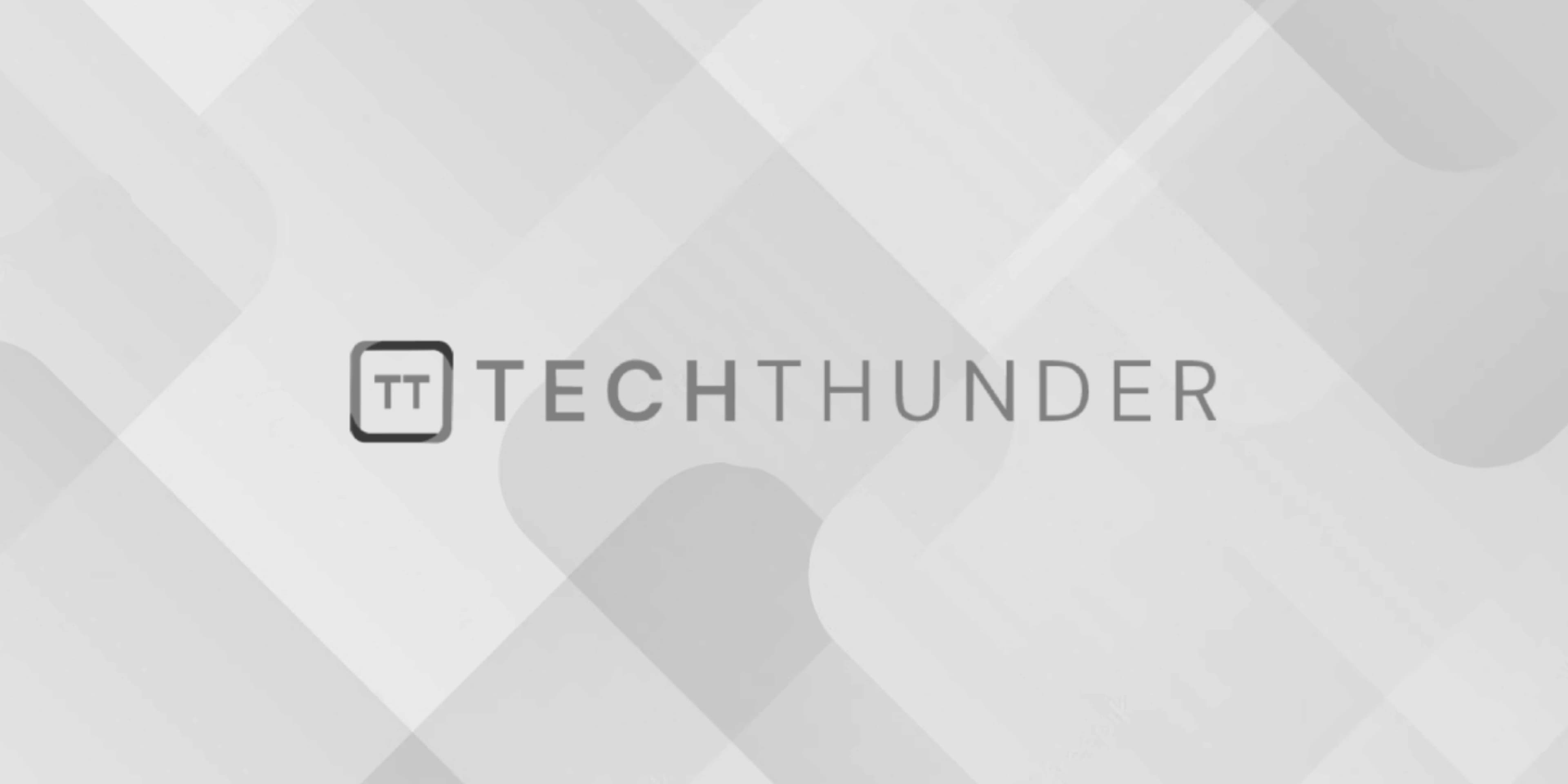
jQuery removeProp() method
The jQuery.removeProp()
method is used to remove a property from the selected elements. It is primarily designed to remove properties set with the jQuery.prop()
method.
Here’s the basic syntax of the removeProp()
method:
$(selector).removeProp(propertyName)
Parameters:
selector
: The selector for the elements from which to remove the property.propertyName
: The name of the property to remove.
Return Value:
The removeProp()
method returns the selected jQuery object to support method chaining.
Keep in mind that this method is mainly used to remove properties that were set using the prop()
method. It will not remove standard HTML attributes. For removing attributes, you should use the removeAttr()
method.
Example:
<!DOCTYPE html>
<html>
<head>
<title>jQuery removeProp() Method Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<input type="text" id="inputField" value="Hello, world!" readonly>
<script>
$(document).ready(function() {
// Disable the input field using prop()
$("#inputField").prop("disabled", true);
// Remove the "disabled" property
$("#inputField").removeProp("disabled");
});
</script>
</body>
</html>
In this example, we have an input field with the ID “inputField.” We initially disable the input field using the prop()
method to set the “disabled” property to true
. Later, we use the removeProp()
method to remove the “disabled” property, effectively re-enabling the input field.
However, please note that using removeProp()
to remove standard HTML attributes is not recommended. For example, if you use removeProp("disabled")
to remove the “disabled” attribute from an input element, it will not work as expected, because “disabled” is an attribute, not a property. In such cases, you should use the removeAttr()
method instead:
$("#inputField").removeAttr("disabled");
Using removeAttr()
will properly remove the “disabled” attribute from the input element.
In summary, use removeProp()
when you want to remove properties set using prop()
, and use removeAttr()
when you want to remove standard HTML attributes from elements.