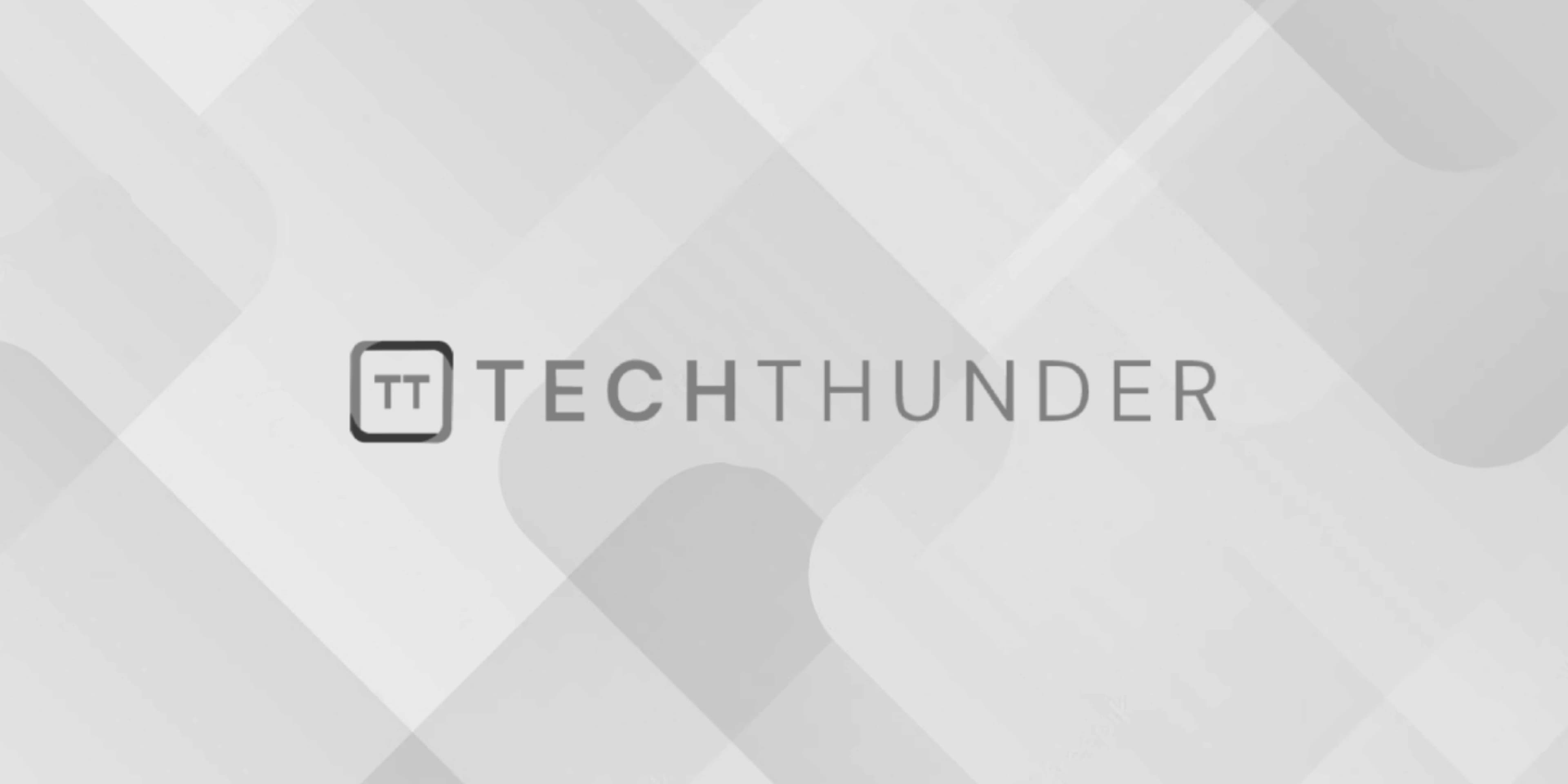
jQuery Effects
The jQuery provides a variety of built-in effects that allow you to animate and enhance the visual appearance of elements on a web page. These effects can be used to create smooth transitions, show or hide elements with animation, and add dynamic interactivity to the user interface. Here are some of the most commonly used jQuery effects:
- fadeIn() / fadeOut() / fadeToggle(): These methods allow you to smoothly fade elements in or out, adjusting their opacity over a specified duration.
fadeIn()
makes an element visible by gradually increasing its opacity,fadeOut()
hides an element by decreasing its opacity, andfadeToggle()
toggles the visibility with a fade effect. - slideUp() / slideDown() / slideToggle(): These methods animate the height of elements, creating sliding effects.
slideUp()
hides an element by sliding it up,slideDown()
makes an element visible by sliding it down, andslideToggle()
toggles the visibility with a sliding effect. - animate(): This method provides a way to create custom animations on selected elements. You can animate any numeric CSS property over a specified duration, such as changing the width, height, position, opacity, or color of an element.
- show() / hide() / toggle(): These methods provide simple ways to show or hide elements with optional animation.
show()
makes an element visible,hide()
hides an element, andtoggle()
toggles the visibility of elements with an optional animation. - delay(): The
delay()
method is used to introduce a time delay before the execution of subsequent functions in the effects queue. It is useful for creating timed effects or synchronizing animations. - fadeIn() / fadeOut() / fadeTo(): These methods provide variations of the fading effect.
fadeTo()
allows you to set a specific target opacity when fading an element in or out.
Here’s an example of using some jQuery effects:
HTML:
<!DOCTYPE html>
<html>
<head>
<title>jQuery Effects</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<style>
.box {
width: 100px;
height: 100px;
background-color: red;
margin: 10px;
}
</style>
</head>
<body>
<button id="fadeInButton">Fade In</button>
<button id="slideUpButton">Slide Up</button>
<button id="animateButton">Animate</button>
<div class="box" id="myBox"></div>
<script>
$(document).ready(function() {
$('#fadeInButton').click(function() {
$('#myBox').fadeIn(1000); // Fade in over 1 second
});
$('#slideUpButton').click(function() {
$('#myBox').slideUp(1000); // Slide up over 1 second
});
$('#animateButton').click(function() {
// Custom animation: change width and background color
$('#myBox').animate({
width: '200px',
backgroundColor: 'blue'
}, 1000); // Animation duration is 1 second
});
});
</script>
</body>
</html>
In this example, we have three buttons: “Fade In,” “Slide Up,” and “Animate.” Each button triggers a different jQuery effect on the red box (<div>
element) with the ID “myBox.”
- Clicking the “Fade In” button will fade in the box over 1 second.
- Clicking the “Slide Up” button will slide up the box over 1 second.
- Clicking the “Animate” button will animate the box by changing its width and background color over 1 second.
These are just a few examples of jQuery effects, and there are many more possibilities to create engaging and interactive user experiences on web pages. jQuery’s effects make it easier to add dynamic visual effects without writing complex CSS transitions or animations.