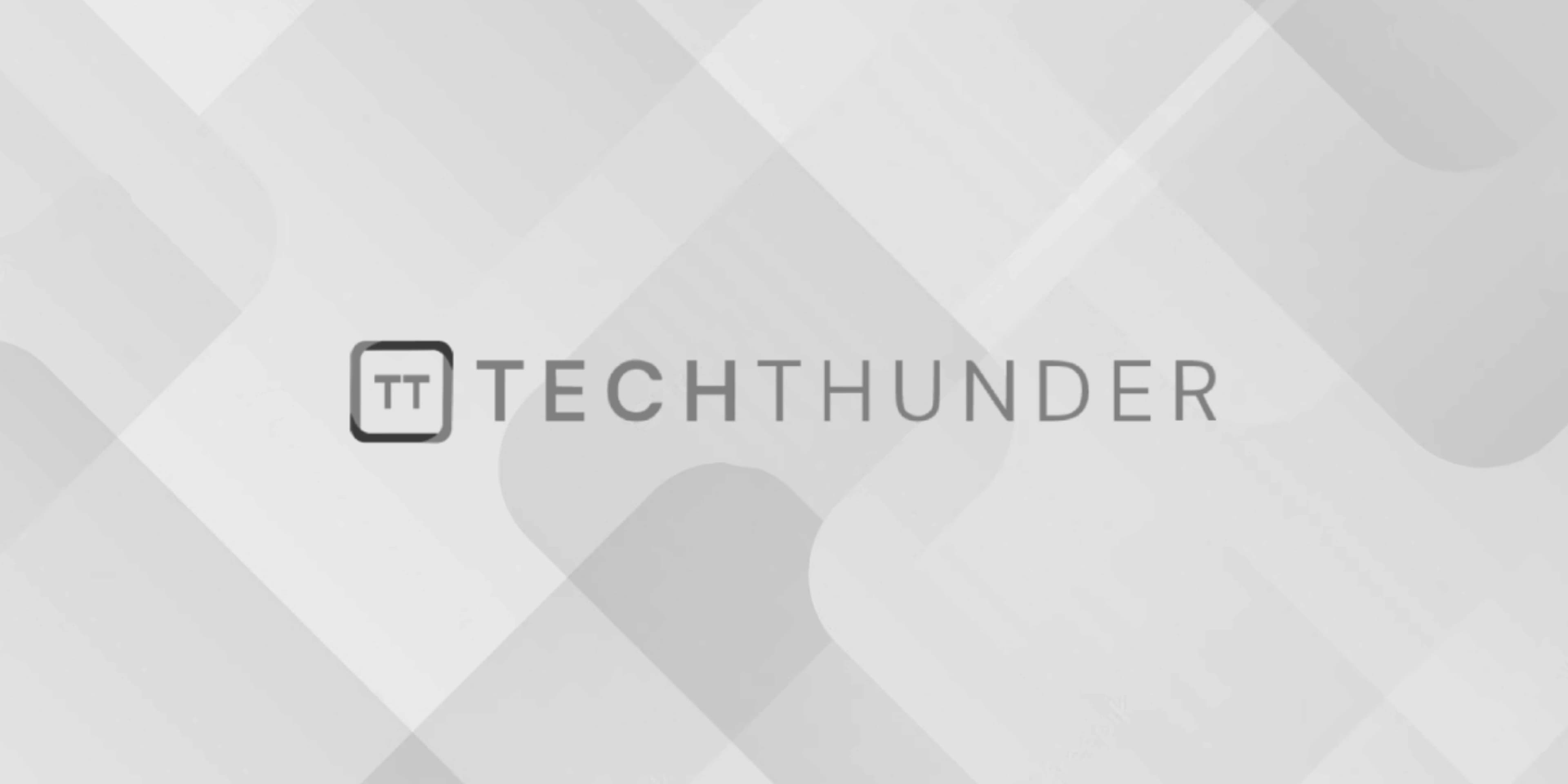
jQuery read more/read less
Implementing a “Read More” and “Read Less” functionality using jQuery allows you to display truncated text initially and show the full text when the user clicks on “Read More.” Clicking “Read Less” will then collapse the text back to its truncated form. Here’s how you can achieve this:
HTML:
<div class="content">
<p>
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Quisque et justo a nulla commodo hendrerit eu vel nunc. Suspendisse at nunc at purus mattis tincidunt in ut erat. Curabitur nec metus vel metus egestas scelerisque.
</p>
<a href="#" class="read-more">Read More</a>
</div>
CSS:
.content {
max-height: 80px; /* Set a maximum height for the content to show only a few lines initially */
overflow: hidden; /* Hide the overflowing content */
transition: max-height 0.3s ease; /* Add a smooth transition effect */
}
.read-less {
display: none; /* Hide the "Read Less" link initially */
}
JavaScript/jQuery:
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script>
$(document).ready(function() {
$('.read-more').click(function(e) {
e.preventDefault();
var content = $(this).parent('.content');
if (content.hasClass('expanded')) {
// Collapse the content
content.removeClass('expanded');
content.find('.read-less').hide();
content.find('.read-more').show().text('Read More');
} else {
// Expand the content
content.addClass('expanded');
content.find('.read-less').show();
content.find('.read-more').text('Read Less');
}
});
});
</script>
In this example, we have a div
element with class content
containing a paragraph of text. Initially, the text is truncated to a maximum height of 80px with overflow: hidden
. Clicking on the “Read More” link will expand the content to show the full text, and the “Read More” link will change to “Read Less.” Clicking on “Read Less” will collapse the content back to its truncated form.
By toggling the class expanded
on the content
div, we can control whether the content is expanded or collapsed. The transition
CSS property provides a smooth animation effect when the content expands and collapses.
With this setup, you can easily apply the “Read More” and “Read Less” functionality to multiple content sections on your website.