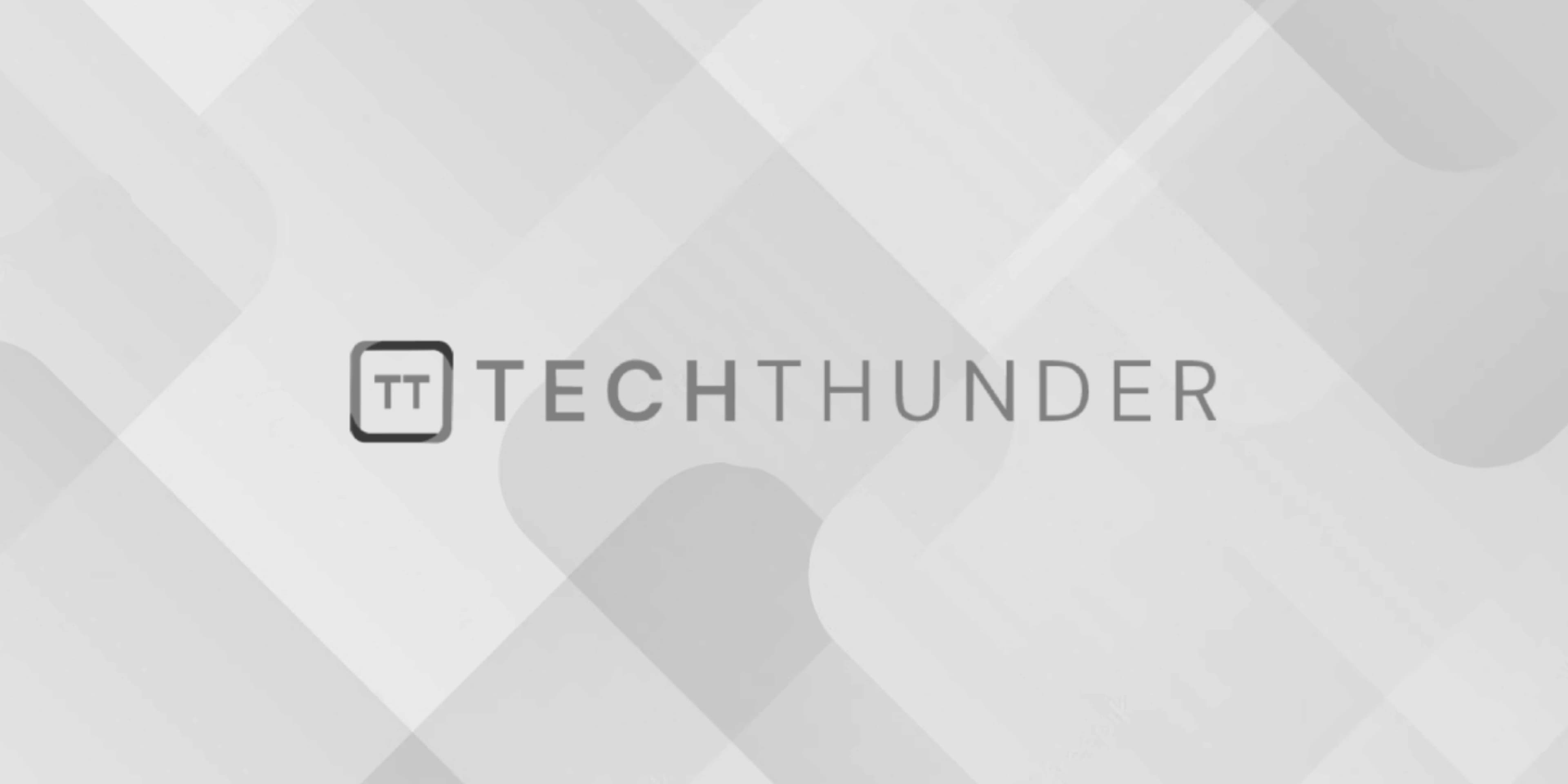
jQuery filter() method
The jQuery filter()
method is used to filter a set of matched elements based on a specified selector or a function. It reduces the set of matched elements to those that match the specified criteria.
Here’s the basic syntax of the filter()
method:
$(selector).filter(filterExpression)
Parameters:
selector
: A selector string representing the elements to be filtered.filterExpression
: A selector string, element, function, or another jQuery object representing the elements that should be included in the filtered set.
Example:
<!DOCTYPE html>
<html>
<head>
<title>jQuery filter() Method Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<ul>
<li>Apple</li>
<li>Orange</li>
<li>Banana</li>
<li>Cherry</li>
</ul>
<script>
$(document).ready(function() {
// Filter the list items to include only those containing the letter "a"
$('li').filter(':contains("a")').css('color', 'blue');
});
</script>
</body>
</html>
In this example, we have an unordered list (<ul>
) with four list items (<li>
). We use the filter()
method to select only the list items that contain the letter “a” and change their text color to blue using the css()
method.
The filter()
method is useful for refining a set of matched elements by selecting a subset that meets specific criteria. It allows you to create more targeted selections for manipulation, styling, or further processing. You can use various filter expressions, such as :contains()
, :even
, :odd
, :first
, :last
, etc., or even pass a custom function to perform more complex filtering based on your specific needs.