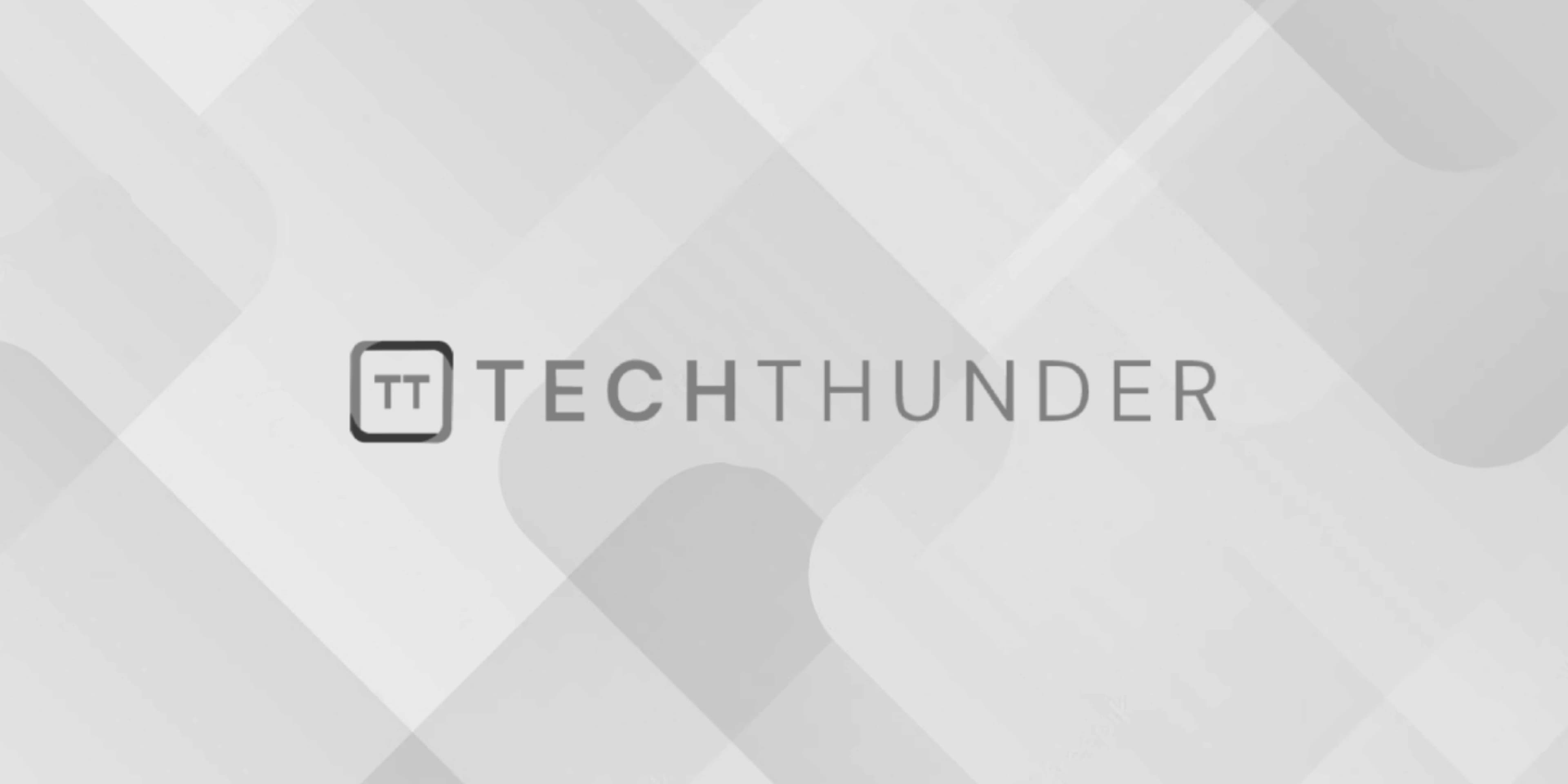
JQuery Validation
jQuery Validation is a powerful plugin that provides an easy-to-use and flexible way to validate user input in web forms. It is built on top of the jQuery library and allows you to define validation rules for form fields, display error messages, and handle form submission gracefully.
To use jQuery Validation, follow these steps:
Step 1: Include jQuery and jQuery Validation scripts in your HTML file.
<!DOCTYPE html>
<html>
<head>
<title>jQuery Validation</title>
<!-- Link to jQuery library -->
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<!-- Link to jQuery Validation plugin -->
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery-validate/1.19.3/jquery.validate.min.js"></script>
</head>
<body>
<!-- Your form goes here -->
<form id="myForm">
<input type="text" name="name" placeholder="Name" required>
<input type="email" name="email" placeholder="Email" required>
<button type="submit">Submit</button>
</form>
<script src="script.js"></script>
</body>
</html>
Step 2: Create a JavaScript file (script.js) to define the validation rules and error messages.
$(document).ready(function() {
$('#myForm').validate({
rules: {
name: {
required: true,
minlength: 3
},
email: {
required: true,
email: true
}
},
messages: {
name: {
required: 'Please enter your name',
minlength: 'Name must be at least 3 characters long'
},
email: {
required: 'Please enter your email',
email: 'Please enter a valid email address'
}
},
submitHandler: function(form) {
// Handle form submission here
form.submit();
}
});
});
In this example, we use jQuery Validation to validate the form with two fields: “name” and “email”. The validate()
method is called on the form element (#myForm) to initialize the validation.
The rules
option defines the validation rules for each form field. In this case, we specify that the “name” field is required and must have a minimum length of 3 characters, and the “email” field is required and must be a valid email address.
The messages
option provides custom error messages for each field when the validation fails.
The submitHandler
option specifies the function that will be executed when the form is successfully validated and submitted. In this example, we simply submit the form, but you can perform other actions, such as sending data to the server using Ajax.
With this implementation, the form will be automatically validated when the user submits it. If any field fails validation, an error message will be displayed, and the form will not be submitted until all validation rules are met. The user-friendly error messages will guide the user in providing the correct input.
You can customize the validation rules, error messages, and behavior to suit your specific form requirements. The jQuery Validation plugin offers a wide range of validation options, making it a valuable tool for enhancing the usability and reliability of web forms.