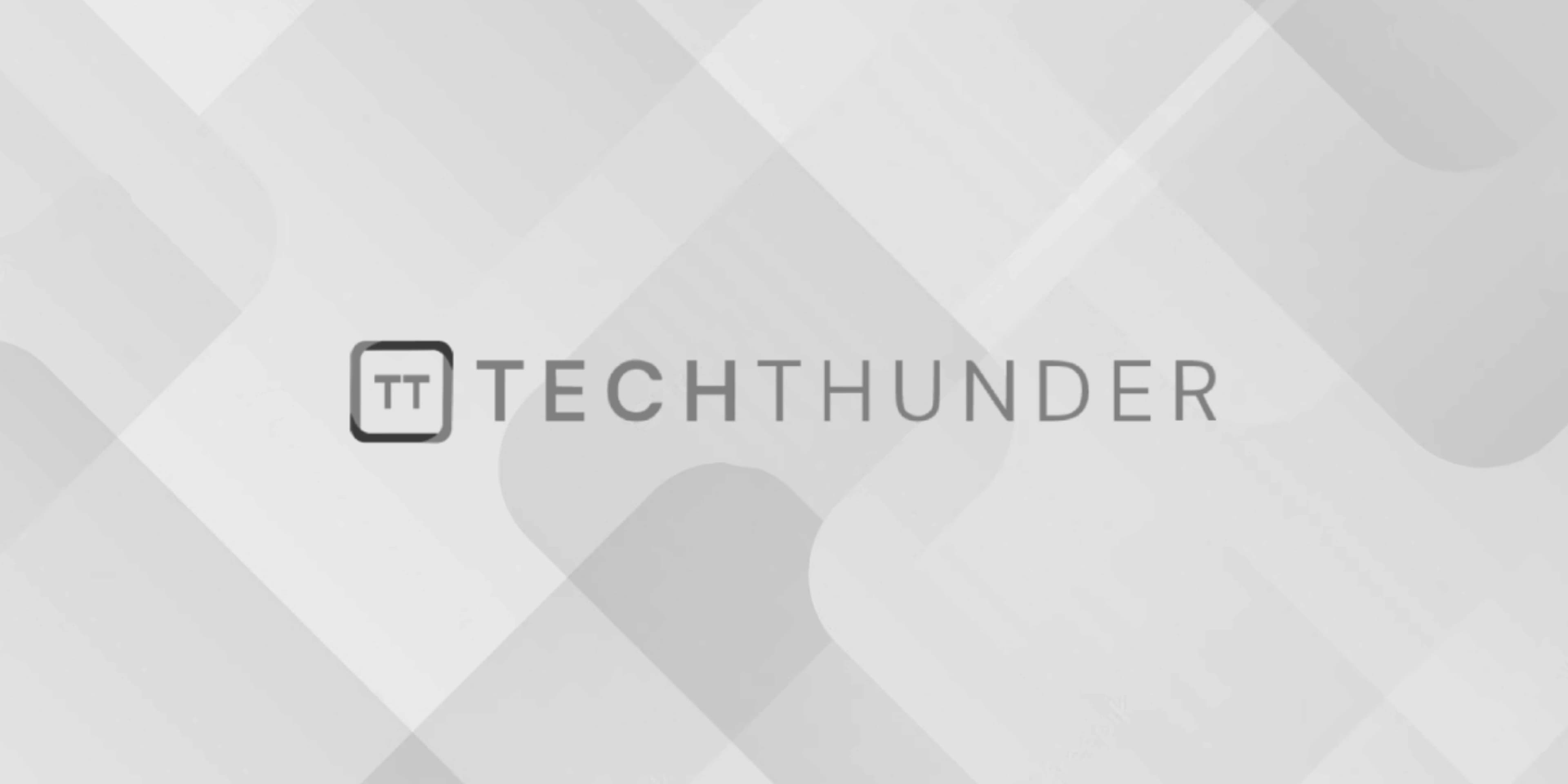
184 views
Create 3D Text Effect using HTML and CSS and jQuery
Creating a 3D text effect involves using CSS transformations and animations. While the effect won’t be true 3D, we can achieve a visually convincing 3D-like effect using HTML, CSS, and jQuery. Here’s a step-by-step guide to create a simple 3D text effect:
HTML:
<!DOCTYPE html>
<html>
<head>
<title>3D Text Effect</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div class="container">
<h1 class="text">3D Text Effect</h1>
</div>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script src="script.js"></script>
</body>
</html>
CSS (styles.css):
body {
font-family: Arial, sans-serif;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
margin: 0;
}
.container {
perspective: 500px; /* Adjust this value to control the depth of the 3D effect */
}
.text {
font-size: 3rem;
color: #fff;
background: linear-gradient(45deg, #ff3d00, #ffba00);
padding: 10px 20px;
border-radius: 5px;
text-shadow: 2px 2px 4px rgba(0, 0, 0, 0.4);
transform-origin: center;
transition: transform 0.5s ease-in-out;
}
.text:hover {
transform: translateZ(50px) rotateX(20deg) scale(1.2);
}
JavaScript (script.js):
// You can add jQuery code here if needed, though it's not required for the 3D text effect.
Explanation:
- In the HTML file, we have a
div
with the classcontainer
that will provide the 3D perspective. Inside the container, there’s anh1
element with the classtext
, which represents the 3D text we want to animate. - In the CSS file, we apply the 3D effect to the
.container
using theperspective
property. Theperspective
property defines the distance between the viewer and the z=0 plane. Increasing the value will create a stronger 3D effect. - The
.text
class represents the 3D text. We set thetransform-origin
property tocenter
, which is the default, but it’s essential to specify it for 3D transformations to work correctly. - On hover, we apply a 3D-like transformation to the text using the
:hover
pseudo-class. We use thetransform
property to applytranslateZ
,rotateX
, andscale
transformations.translateZ
moves the element along the z-axis (depth),rotateX
rotates the element around the x-axis, andscale
increases the size of the text. - The
transition
property is used to make the animation smooth and gradual when the mouse hovers over the text.
Note: This example uses CSS to create the 3D effect, and jQuery is not required for this particular effect. However, you can use jQuery for other interactive features or to manipulate the 3D text effect based on user interactions, if needed.