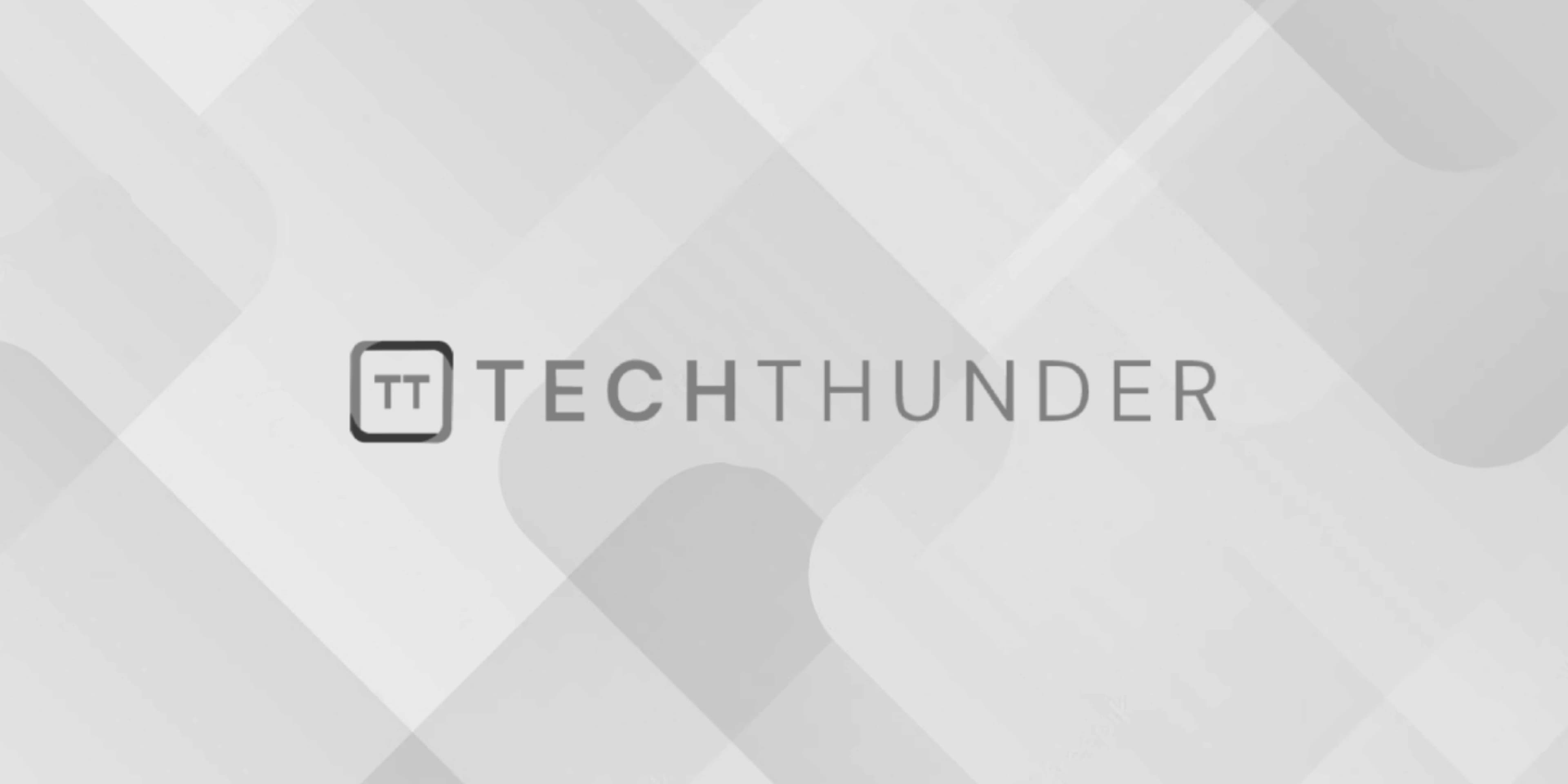
jQuery click() method
The click()
method in jQuery is an event handler that allows you to attach a function to be executed when a specified element is clicked or activated by the user. It is one of the most commonly used event handlers in jQuery and is used to perform actions in response to user clicks.
The syntax for using the click()
method is as follows:
$(selector).click(function(event) {
// Function body
});
selector
: It is a string that specifies the elements to be selected.function(event)
: The callback function that will be executed when theclick
event occurs. It takes one argument:event
: The event object containing information about theclick
event. It provides details about the event, such as the mouse position, the target element, and other related information.
Here’s an example of how you can use the click()
method:
HTML:
<button id="myButton">Click me</button>
JavaScript:
// Attach a click event handler to the button element
$('#myButton').click(function(event) {
console.log('Button clicked!');
});
In the above example, when you click the button with the ID “myButton,” the click()
event handler will be triggered, and it will log “Button clicked!” to the console.
The click()
event handler is commonly used for various interactive elements such as buttons, links, and other clickable elements. It allows you to define actions or behaviors that should occur when the user interacts with these elements.
Keep in mind that you can use the on()
method with the click
event as well if you want to attach the event handler to dynamically created or removed elements, or if you need more flexibility in event handling. For example:
// Using on() method for click event
$(document).on('click', '#myButton', function(event) {
console.log('Button clicked!');
});
This version of the event binding will work for elements that are present at the time of binding and future elements added to the DOM that match the selector ‘#myButton’.