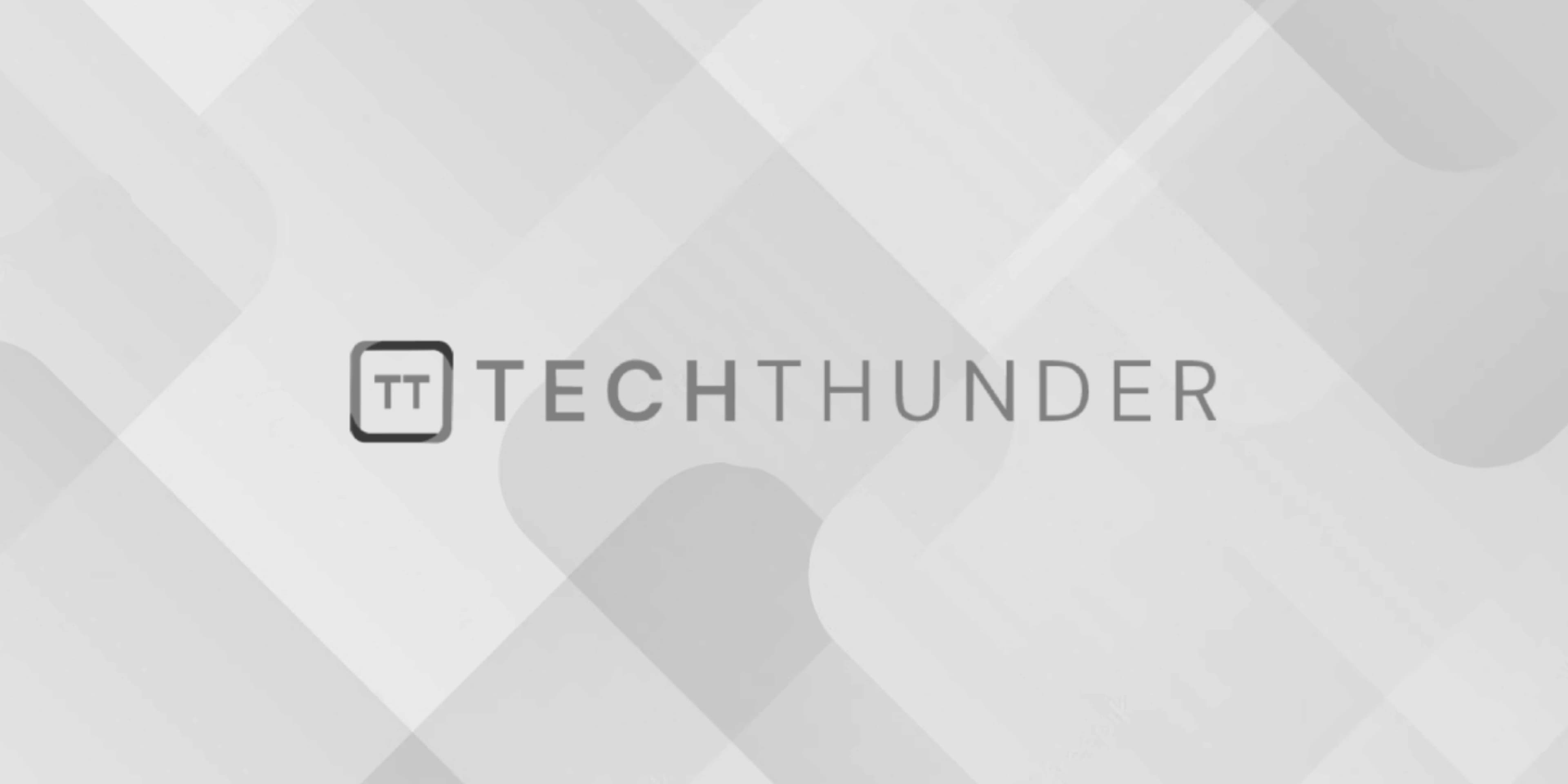
jQuery map() function
The jQuery map()
function is used to transform elements of an array or a jQuery object into a new array by applying a callback function to each element. It allows you to modify the values or properties of the elements and create a new array based on the results.
Here’s the basic syntax of the map()
function:
jQuery.map(array, callback)
Parameters:
array
: The array or jQuery object to be mapped.callback
: The function that will be called for each element of the array. The function takes two arguments: the current element value and the index of the element. The function should return the new value for the element, which will be included in the new mapped array.
Return Value:
The map()
function returns a new array containing the results of applying the callback function to each element of the original array.
Example:
<!DOCTYPE html>
<html>
<head>
<title>jQuery map() Function Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<ul id="originalList">
<li>Apple</li>
<li>Banana</li>
<li>Orange</li>
</ul>
<script>
$(document).ready(function() {
// Get the text of each list item and create a new array with the lengths of the text
var originalListItems = $("#originalList li");
var textLengths = originalListItems.map(function(index, element) {
return $(element).text().length;
}).get();
// Display the new array containing the lengths of the text
console.log(textLengths); // Output: [5, 6, 6]
});
</script>
</body>
</html>
In this example, we have an unordered list (<ul>
) with three list items (<li>
), each containing a fruit name. We use the map()
function to create a new array called textLengths
, which contains the lengths of the text of each list item. The callback function takes the index and element as arguments, accesses the text of each list item using $(element).text()
, calculates its length, and returns the length to be included in the new array.
When you run the code, you will see the new array [5, 6, 6]
displayed in the console. This array represents the lengths of the text in each list item.
The map()
function is useful when you want to transform elements in an array or jQuery object into a new array based on some specific logic or operation. It is often used to simplify data manipulation and transformation tasks when working with collections of elements.