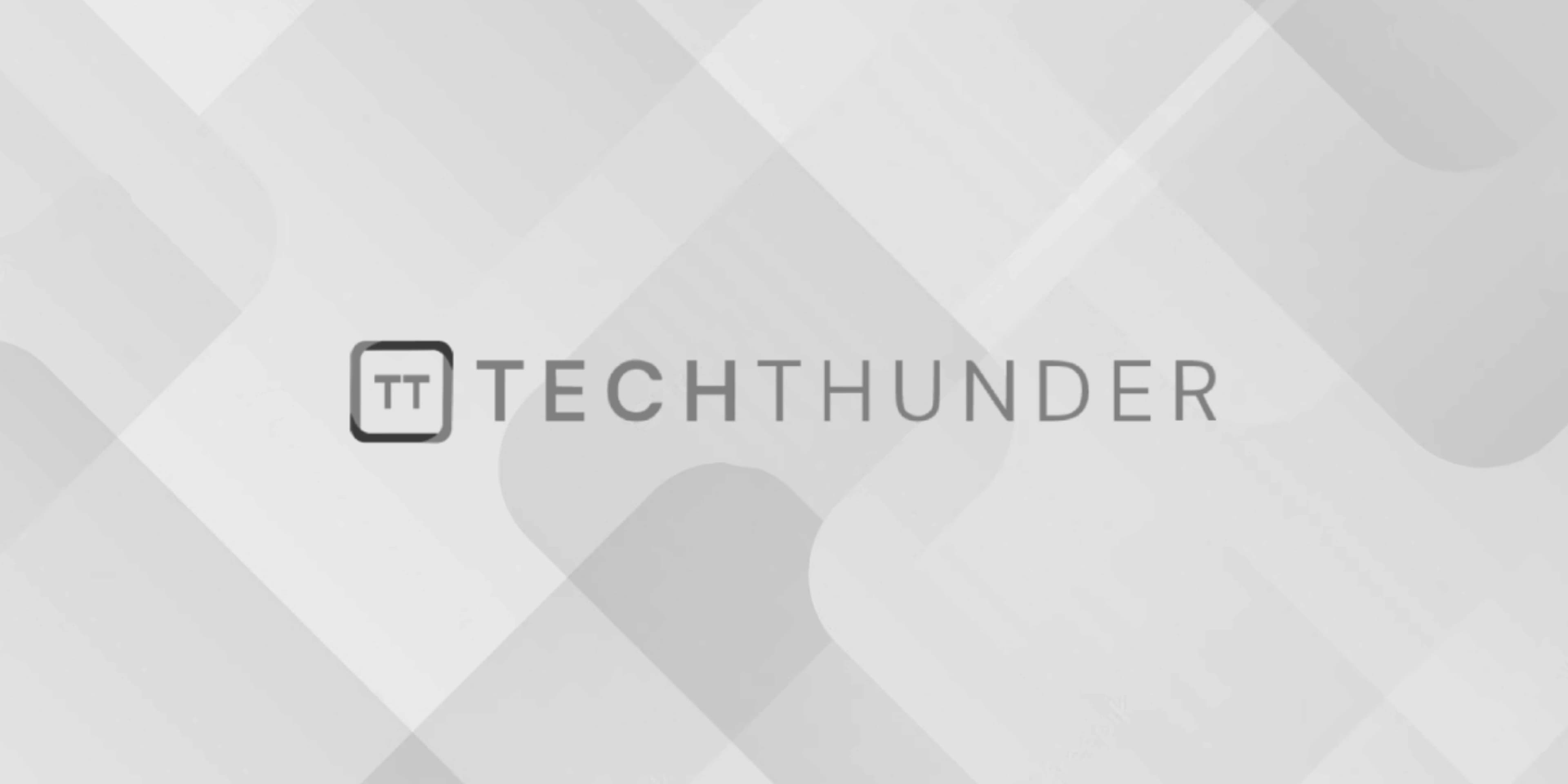
jQuery length property
The jQuery length
property is used to get the number of elements in a jQuery object or collection. It is a numeric property that indicates the count of elements that match the selector or criteria used to create the jQuery object.
Here’s an example of how to use the length
property:
<!DOCTYPE html>
<html>
<head>
<title>jQuery length Property Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<ul>
<li>Item 1</li>
<li>Item 2</li>
<li>Item 3</li>
</ul>
<script>
$(document).ready(function() {
// Select all li elements and check the length
var listItemCount = $('li').length;
console.log('Number of li elements:', listItemCount);
});
</script>
</body>
</html>
In this example, we have an unordered list (<ul>
) with three list items (<li>
). We use the $()
function to create a jQuery object that selects all the <li>
elements, and then we access the length
property to find out the number of list items. The result will be logged to the console, and it will show that there are three <li>
elements.
The length
property is useful for checking the size of a jQuery collection, which allows you to determine the number of elements that match a particular selector or filter. It is commonly used in conditional statements or for debugging purposes to see how many elements are being selected.