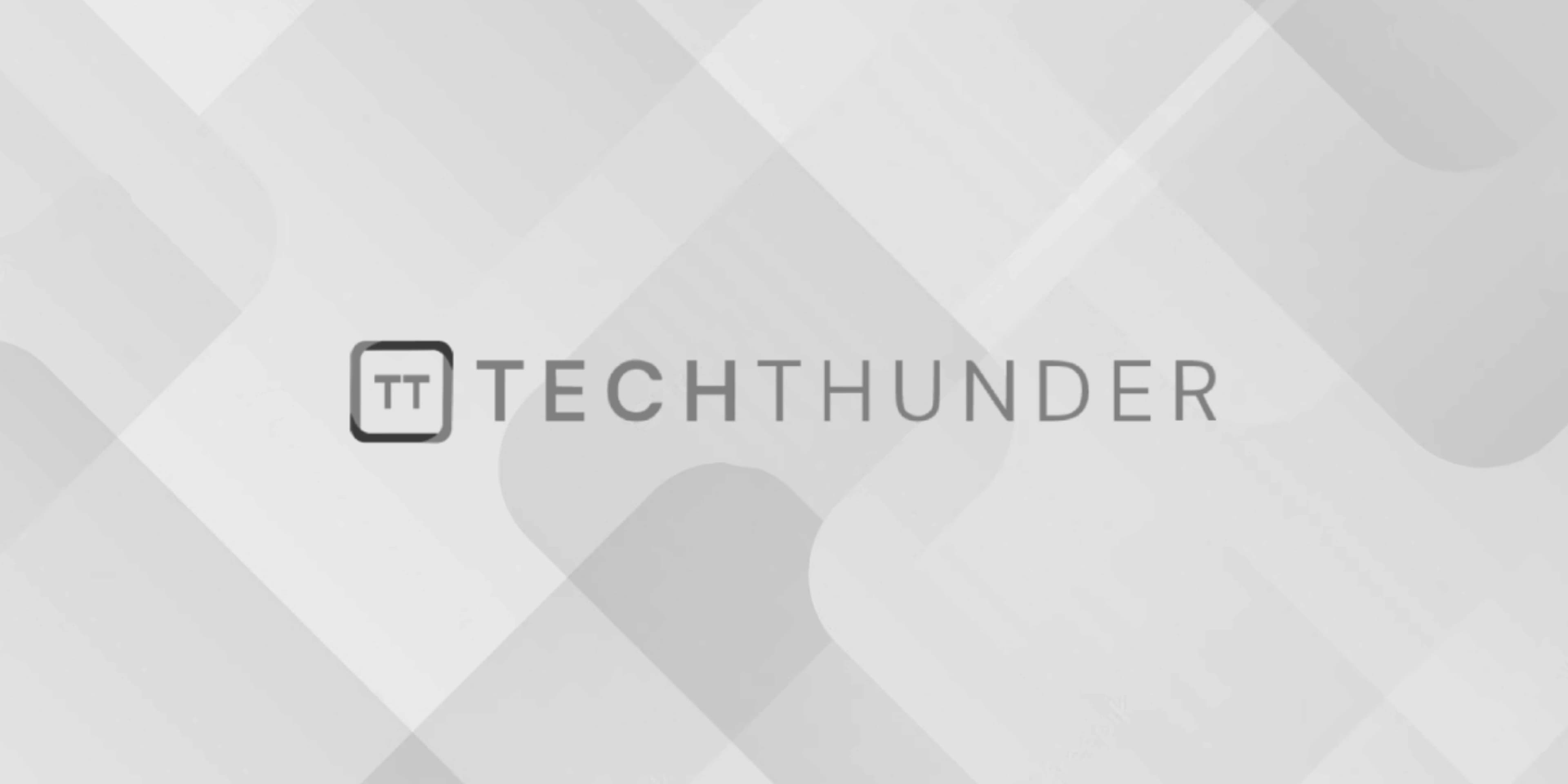
jQuery makeArray() method
As of my last update in September 2021, the jQuery.makeArray()
method in jQuery is used to convert an array-like or iterable object into a true JavaScript array. It allows you to work with the data as an array and use array-specific methods and properties on the converted result.
Here’s the basic syntax of the jQuery.makeArray()
method:
jQuery.makeArray(obj)
Parameters:
obj
: The array-like or iterable object to be converted into a JavaScript array.
Return Value:
The jQuery.makeArray()
method returns a new JavaScript array that contains the elements of the original object.
Example:
<!DOCTYPE html>
<html>
<head>
<title>jQuery makeArray() Method Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<div class="item">Item 1</div>
<div class="item">Item 2</div>
<div class="item">Item 3</div>
<button id="convertToArrayButton">Convert to Array</button>
<script>
$(document).ready(function() {
$("#convertToArrayButton").click(function() {
// Convert the jQuery object containing the elements with class "item" into a JavaScript array
var itemArray = $.makeArray($(".item"));
console.log(itemArray);
});
});
</script>
</body>
</html>
In this example, we have three div
elements with the class “item.” When the “Convert to Array” button is clicked, the click
event handler function is executed. Inside the function, we use the $.makeArray()
method to convert the jQuery object containing the elements with class “item” into a JavaScript array called itemArray
. We then log the resulting array to the console.
The output in the console will be an array containing the div
elements with class “item”:
[<div class="item">Item 1</div>, <div class="item">Item 2</div>, <div class="item">Item 3</div>]
By converting the jQuery object into a JavaScript array, you can access and manipulate the elements using array methods like .forEach()
, .filter()
, .map()
, and so on.
Keep in mind that starting from jQuery version 3.0, the makeArray()
method is deprecated, and you can use the standard JavaScript Array.from()
method to achieve the same result:
var itemArray = Array.from($(".item"));
Or even use the spread syntax for converting a jQuery object to an array:
var itemArray = [...$(".item")];
Using Array.from()
or the spread syntax is preferred over makeArray()
for compatibility with modern JavaScript and to follow best practices.