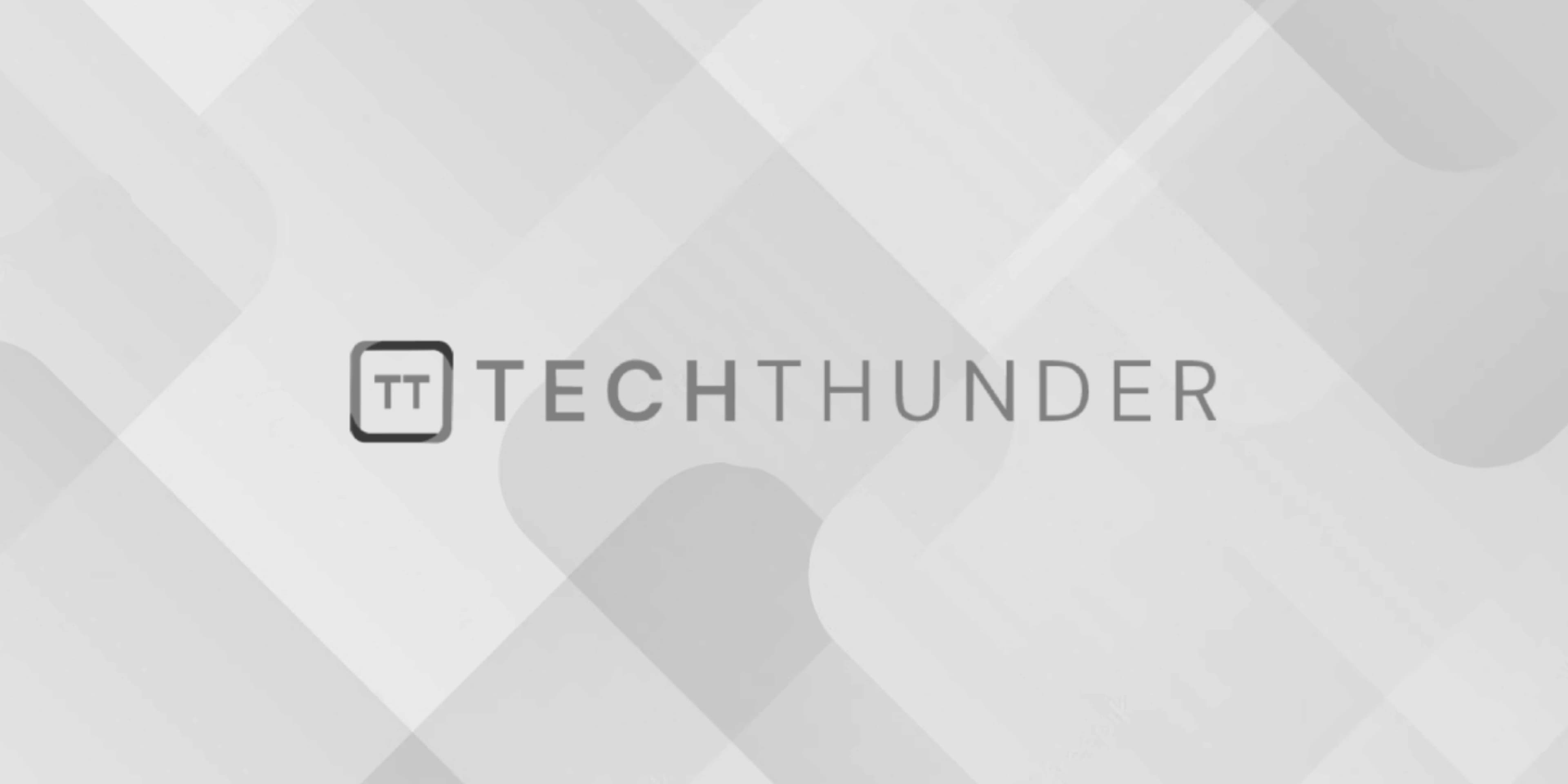
jQuery serializeArray() method
The serializeArray()
method in jQuery is used to create a JavaScript array of objects representing form field names and their values. It is typically used to serialize form data into a format that can be easily submitted to the server using AJAX or other similar methods.
The serializeArray()
method is often used in conjunction with AJAX requests when you need to send form data to the server without reloading the entire page. It allows you to capture the form input values and convert them into a format that can be sent in the request payload.
The syntax for using the serializeArray()
method is as follows:
$(selector).serializeArray();
selector
: It is a string that specifies the form element to be selected.
The method returns an array of objects, where each object represents a form field with two properties: name
and value
. The name
property contains the name attribute of the form field, and the value
property contains the user-entered value.
Here’s an example of how you can use the serializeArray()
method:
HTML:
<form id="myForm">
<input type="text" name="username" value="John">
<input type="email" name="email" value="[email protected]">
<input type="checkbox" name="subscribe" value="true" checked>
</form>
JavaScript:
// Serialize form data into an array of objects
var formData = $('#myForm').serializeArray();
// Log the serialized data to the console
console.log(formData);
In the above example, the serializeArray()
method will convert the form data into the following array:
[
{ name: 'username', value: 'John' },
{ name: 'email', value: '[email protected]' },
{ name: 'subscribe', value: 'true' }
]
You can then use this serialized array to send the data to the server using AJAX or other methods.
Keep in mind that the serializeArray()
method does not include disabled fields, file inputs, or form elements without a name attribute. If you need to include file inputs or disabled fields, you can use the serialize()
method instead, which includes all form fields in the serialized data as a URL-encoded string.