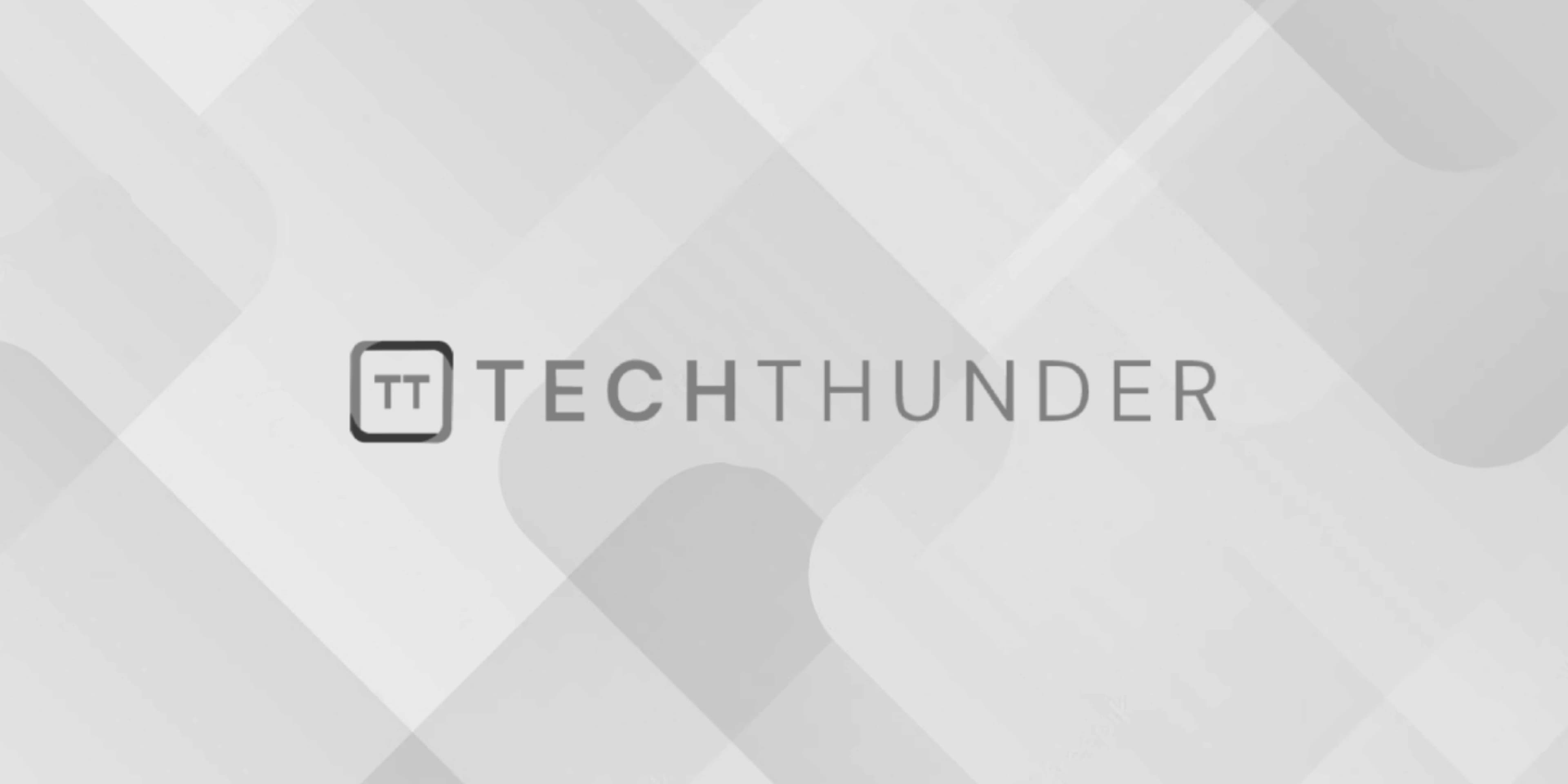
jQuery.uniqueSort() method
The jQuery.uniqueSort()
method is similar to jQuery.unique()
but additionally sorts the array in ascending order after removing duplicates.
Here’s the basic syntax of the jQuery.uniqueSort()
method:
jQuery.uniqueSort(array)
Parameters:
array
: The array from which duplicate elements should be removed and sorted.
Return Value:
The jQuery.uniqueSort()
method returns the original array with duplicate elements removed and the remaining elements sorted in ascending order.
Example:
<!DOCTYPE html>
<html>
<head>
<title>jQuery uniqueSort() Method Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<script>
$(document).ready(function() {
// Sample array with duplicate elements
var myArray = [3, 1, 2, 2, 4, 4, 5, 1, 6];
// Use jQuery.uniqueSort() to remove duplicate elements and sort the array
jQuery.uniqueSort(myArray);
// Display the result
console.log(myArray); // Output: [1, 2, 3, 4, 5, 6]
});
</script>
</body>
</html>
In this example, we have an array myArray
with some duplicate elements. We use the jQuery.uniqueSort()
method to remove the duplicate elements and sort the remaining elements in ascending order.
Please note that just like jQuery.unique()
, the jQuery.uniqueSort()
method is also provided by the jQuery Migrate plugin. As of jQuery version 3.0, these methods were removed from the core library because they were considered not performant for large arrays. Instead, you can use native JavaScript methods like Array.from(new Set(array)).sort()
to achieve the same result of removing duplicates and sorting the array without relying on the jQuery Migrate plugin.