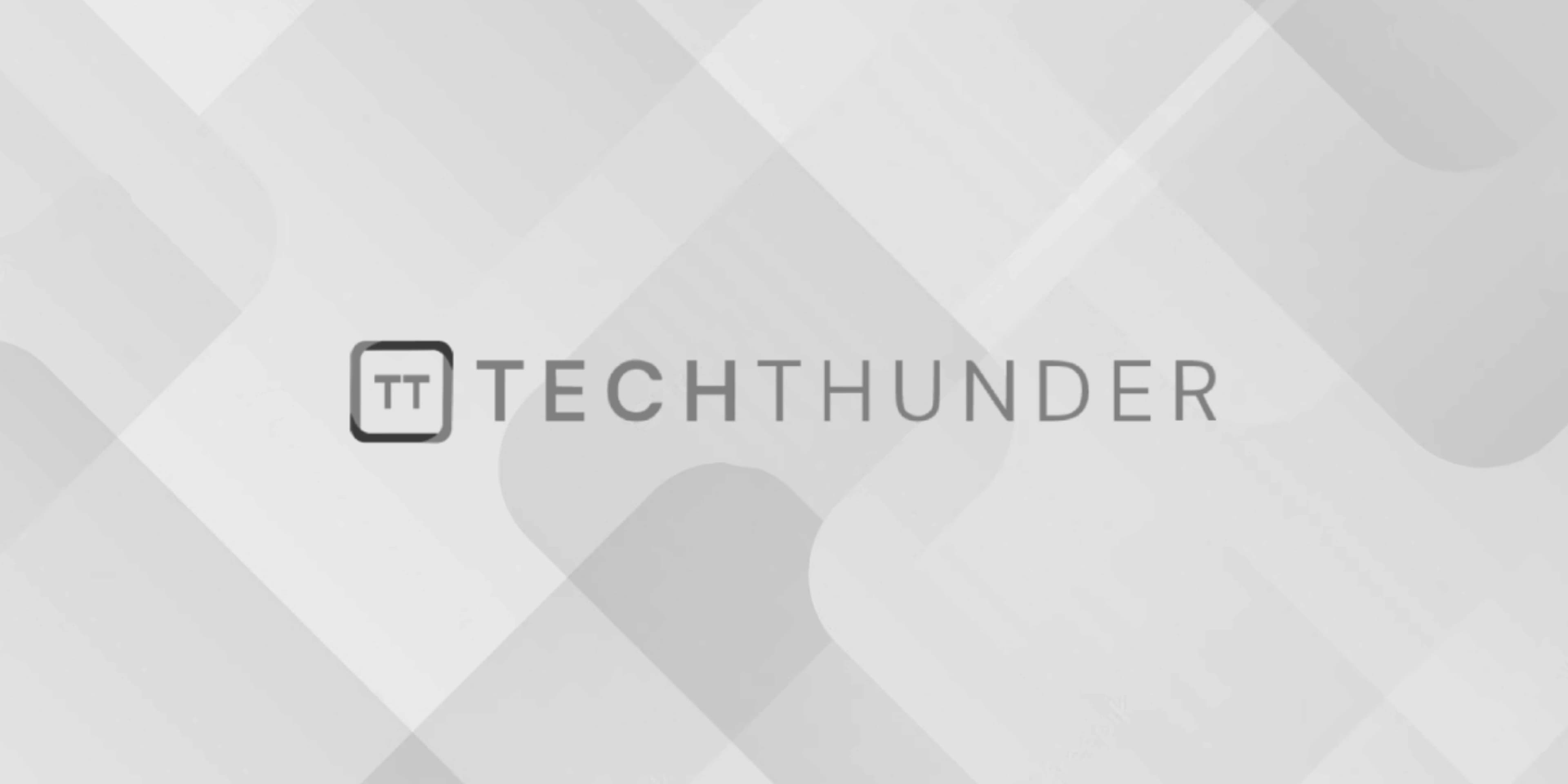
jQuery prependTo() method
The prependTo()
method in jQuery is used to insert the selected elements as the first child of the target elements. It allows you to move or copy elements to the beginning of the target elements’ content. The selected elements will be inserted inside each target element, becoming the new first child of each target.
Here’s the basic syntax of the prependTo()
method:
$(selectedElements).prependTo(targetElements)
Parameters:
selectedElements
: The elements to be inserted as the first child of the target elements. This can be a selector, an HTML string, or a jQuery object containing elements.targetElements
: The target elements where the selected elements will be inserted. This can be a selector, an HTML string, or a jQuery object containing elements.
Return Value:
The prependTo()
method returns the original jQuery object containing the selected elements.
Example:
<!DOCTYPE html>
<html>
<head>
<title>jQuery prependTo() Method Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<div id="target">
<p>This is the existing content.</p>
</div>
<button id="prependButton">Prepend Text</button>
<script>
$(document).ready(function() {
$("#prependButton").click(function() {
// Create a new paragraph element with some text
var newParagraph = $("<p>Hello, I am a new paragraph.</p>");
// Prepend the new paragraph to the target element
newParagraph.prependTo("#target");
});
});
</script>
</body>
</html>
In this example, we have a div
element with the ID “target” containing an existing paragraph element. When the “Prepend Text” button is clicked, the click
event handler function is executed. Inside the function, we create a new paragraph element with the text “Hello, I am a new paragraph.” Then, we use the prependTo()
method to insert the new paragraph as the first child of the “target” div
. As a result, the new paragraph will appear above the existing paragraph, making it the first child of the “target” div
.
Keep in mind that the prependTo()
method can also be used with multiple target elements, in which case the selected elements will be inserted as the first child of each target element. Additionally, it can be used to move elements from one part of the document to another or to create and insert new elements dynamically into the DOM.