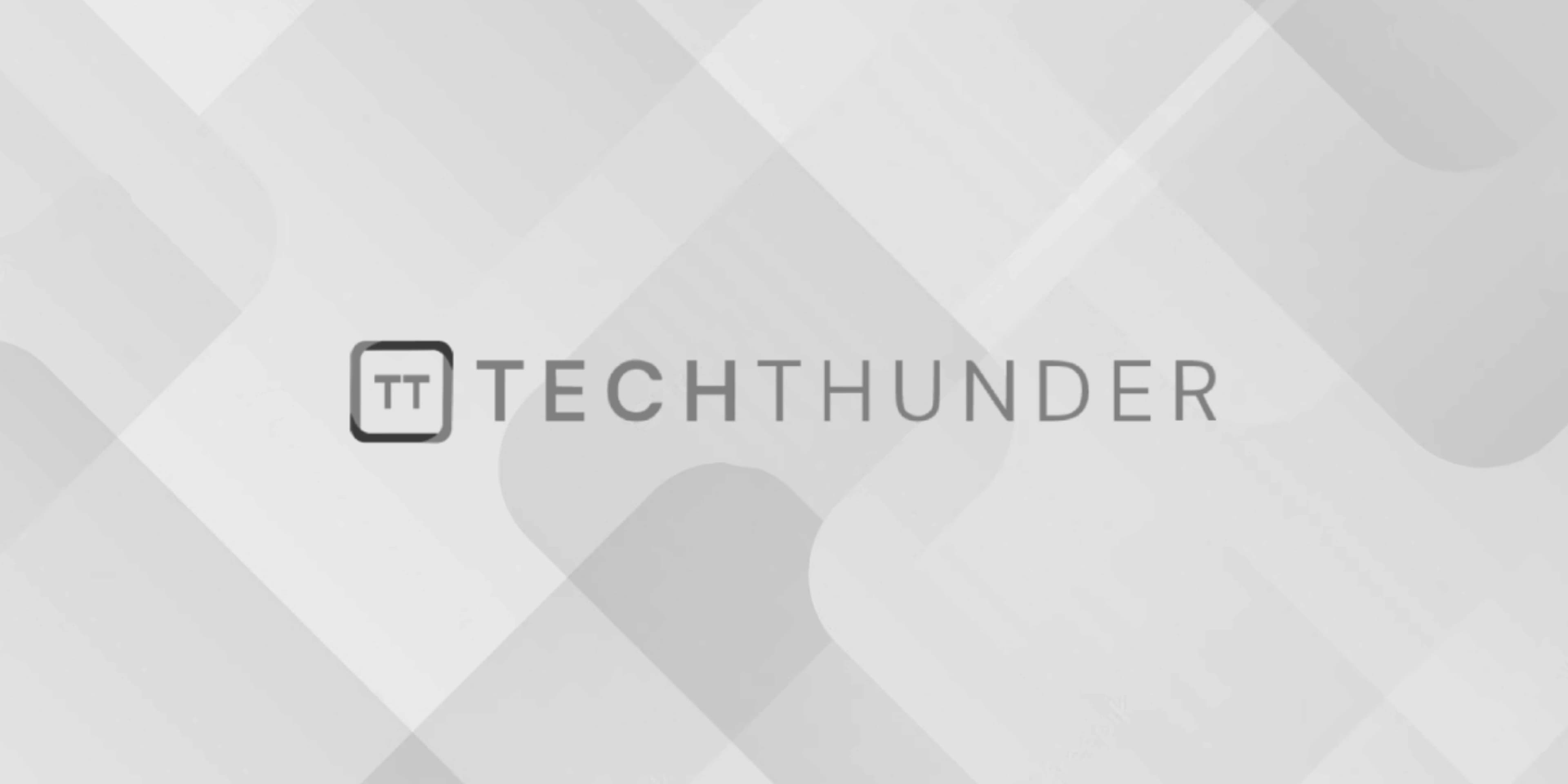
JQuery photo gallery with thumbnail
To create a simple jQuery photo gallery with thumbnails, you can follow these steps:
- Set up your HTML structure for the gallery.
- Use jQuery to handle interactions and display the selected photo.
- Add CSS to style the gallery and thumbnails.
Here’s an example of how to create a basic jQuery photo gallery with thumbnails:
Step 1: HTML structure
<!DOCTYPE html>
<html>
<head>
<title>jQuery Photo Gallery with Thumbnails</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div class="gallery">
<div class="main-photo">
<img src="images/photo1.jpg" alt="Photo 1">
</div>
<div class="thumbnails">
<img src="images/photo1.jpg" alt="Photo 1">
<img src="images/photo2.jpg" alt="Photo 2">
<img src="images/photo3.jpg" alt="Photo 3">
<!-- Add more thumbnails as needed -->
</div>
</div>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script src="script.js"></script>
</body>
</html>
Step 2: JavaScript (script.js)
$(document).ready(function() {
// Handle thumbnail click event
$(".thumbnails img").click(function() {
var selectedPhoto = $(this).attr("src");
$(".main-photo img").attr("src", selectedPhoto);
});
});
Step 3: CSS (styles.css)
body {
margin: 0;
padding: 0;
font-family: Arial, sans-serif;
}
.gallery {
display: flex;
justify-content: center;
align-items: center;
flex-wrap: wrap;
}
.main-photo img {
max-width: 600px;
max-height: 400px;
}
.thumbnails {
margin-top: 20px;
display: flex;
justify-content: center;
}
.thumbnails img {
width: 100px;
height: 100px;
margin: 5px;
cursor: pointer;
}
In this example, the photo gallery is created with two main sections: .main-photo
to display the selected photo and .thumbnails
to show the thumbnail images.
The JavaScript code uses jQuery to handle the click event on each thumbnail image. When a thumbnail is clicked, the selected photo’s source is extracted and set as the source of the main photo using .attr()
.
The CSS styles the gallery and thumbnail images. You can customize the CSS to achieve the desired appearance for your photo gallery.
Remember to replace the src
attributes of the image tags in the HTML with the paths to your actual images.
With these steps, you will have a simple jQuery photo gallery with thumbnails. You can enhance this gallery further by adding transition effects, lightbox functionality, or more advanced features as per your requirements.