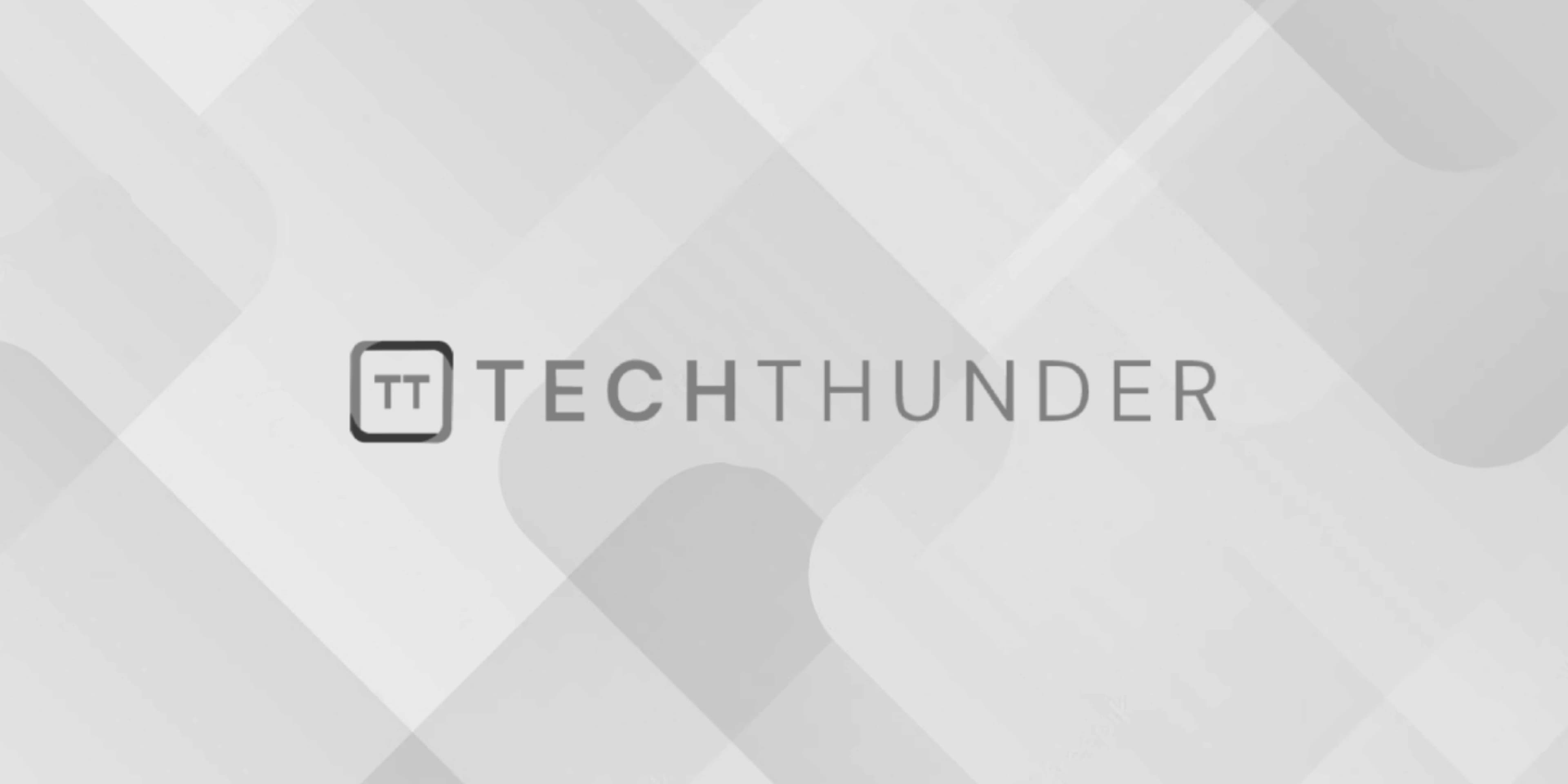
jQuery Excel Export Plugin Library
There are several third-party JavaScript libraries and plugins available that you can use to export data to Excel formats (e.g., .xls or .xlsx).
One popular library for exporting data to Excel is “SheetJS.” SheetJS is a client-side library that allows you to generate Excel files directly in the browser without the need for a server-side component.
To use SheetJS for Excel export, follow these steps:
Step 1: Include Dependencies
First, include the required JavaScript files for SheetJS in your HTML file:
<!DOCTYPE html>
<html>
<head>
<title>Excel Export</title>
<!-- Include jQuery library -->
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<!-- Include SheetJS library -->
<script src="https://cdnjs.cloudflare.com/ajax/libs/xlsx/0.17.3/xlsx.full.min.js"></script>
</head>
<body>
<!-- Your content goes here -->
<script src="script.js"></script>
</body>
</html>
Step 2: Export Data to Excel
In your JavaScript file (e.g., script.js
), you can use SheetJS to export data to Excel:
$(document).ready(function() {
$('#export-btn').on('click', function() {
// Sample data to export (replace with your actual data)
var data = [
['Name', 'Age', 'Email'],
['John Doe', 30, '[email protected]'],
['Jane Smith', 25, '[email protected]'],
// Add more rows as needed
];
// Create a new workbook and sheet
var workbook = XLSX.utils.book_new();
var sheet = XLSX.utils.aoa_to_sheet(data);
// Add the sheet to the workbook
XLSX.utils.book_append_sheet(workbook, sheet, 'Sheet1');
// Export the workbook as an Excel file
XLSX.writeFile(workbook, 'exported_data.xlsx');
});
});
In this example, we use SheetJS to create a new workbook, add a sheet to it, and populate the sheet with sample data. The XLSX.writeFile()
function is then used to export the workbook as an Excel file. When the user clicks the button with the ID export-btn
, the Excel file will be downloaded with the name exported_data.xlsx
.
Please note that the example above uses the aoa_to_sheet()
function to convert an array of arrays (2D array) into an Excel sheet. Depending on your data format, you may need to use different SheetJS functions to convert your data to the appropriate format for Excel.
Always ensure to use plugins and libraries from reputable sources, and check their documentation and support to ensure compatibility and reliability. Additionally, consider handling different data types and formats in your export process to ensure accurate representation in the Excel file.