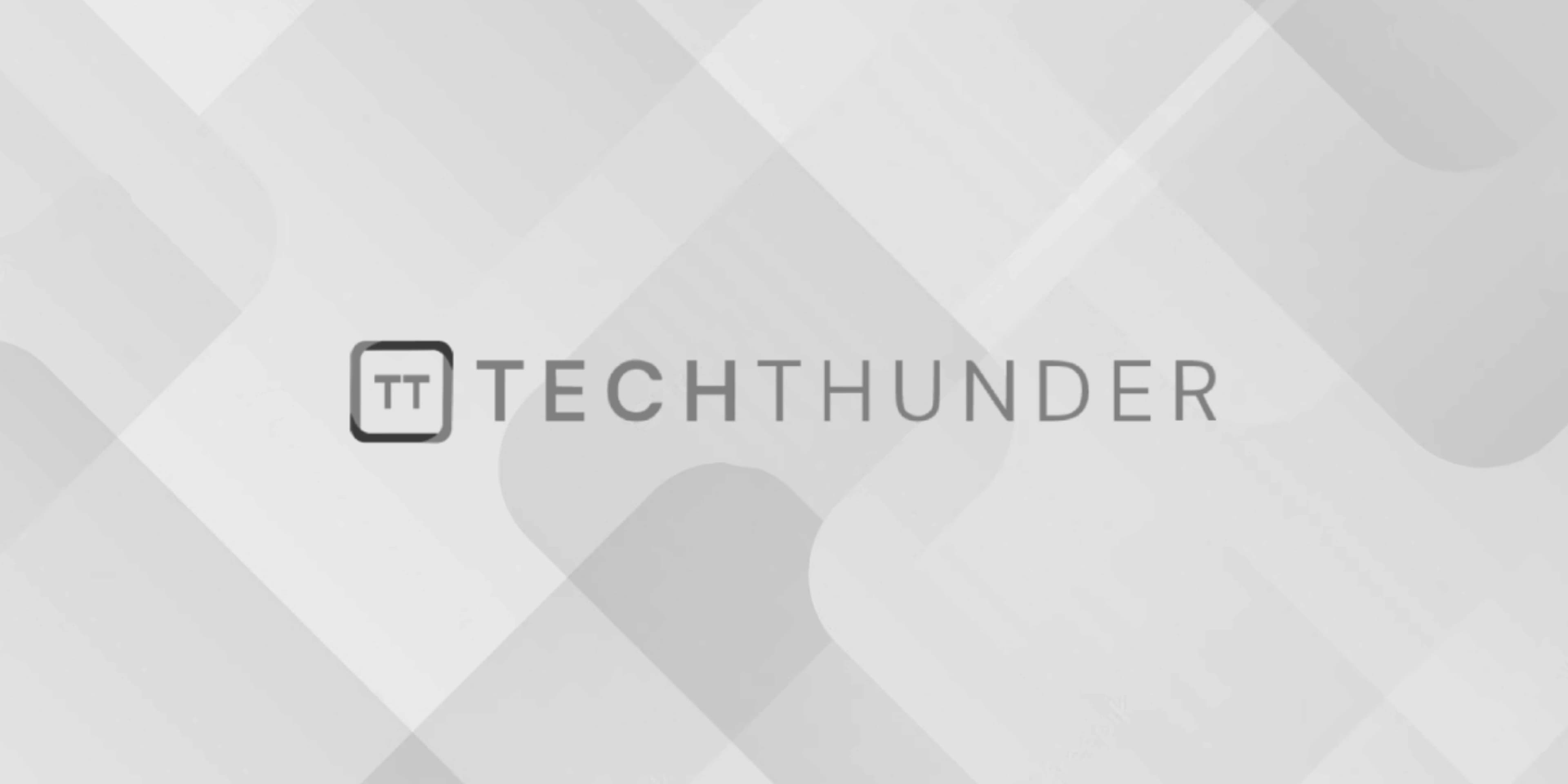
JQuery Input Mask Phone Number Validation
To implement input masking and phone number validation for a phone number input field using jQuery, you can use the “Inputmask” plugin along with regular expressions for validation. The “Inputmask” plugin helps create masked inputs that enforce a specific pattern while typing, and regular expressions are used for phone number validation.
Here’s a step-by-step guide to achieve this:
- Include the necessary CSS and JavaScript files for jQuery and the “Inputmask” plugin in your HTML file.
<!DOCTYPE html>
<html>
<head>
<title>Phone Number Validation with jQuery Inputmask</title>
<!-- Include the Inputmask CSS file (optional, for styling the input) -->
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/jquery.inputmask/5.0.6/css/inputmask.min.css">
</head>
<body>
<div>
<label for="phone">Phone Number:</label>
<input type="text" id="phone" data-inputmask="'mask': '(999) 999-9999'" placeholder="(123) 456-7890">
<span id="error-message" style="color: red; display: none;"></span>
</div>
<!-- Include jQuery and the Inputmask JavaScript files -->
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery.inputmask/5.0.6/jquery.inputmask.min.js"></script>
<script src="script.js"></script>
</body>
</html>
- Implement the JavaScript (script.js):
$(document).ready(function() {
// Initialize the Inputmask
$('#phone').inputmask('(999) 999-9999');
// Phone number validation on input change
$('#phone').on('input', function() {
const phoneInput = $(this);
const phoneNumber = phoneInput.val();
// Validate the phone number using a regular expression
const phoneRegex = /^\(\d{3}\) \d{3}-\d{4}$/;
if (!phoneRegex.test(phoneNumber)) {
// If the phone number is invalid, show an error message
phoneInput.addClass('error');
$('#error-message').text('Please enter a valid phone number.').show();
} else {
// If the phone number is valid, hide the error message
phoneInput.removeClass('error');
$('#error-message').hide();
}
});
});
- Create the CSS (styles.css) – Optional:
.error {
border: 1px solid red;
}
In this example, we use the “Inputmask” plugin to apply the phone number mask to the input field, which displays as () –__. The input is automatically formatted as the user types.
The phone number validation is performed on each input change using a regular expression. The regular expression ^\(\d{3}\) \d{3}-\d{4}$
checks if the phone number matches the pattern () –__, where _
represents a digit. If the phone number is valid, the error message is hidden; otherwise, an error message is displayed. When implementing this code, replace the form submission action (e.g., alert('Form submitted successfully!')
) with your desired action.
You will have a phone number input field with an input mask and validation to ensure that the phone number is in the correct format as the user types.