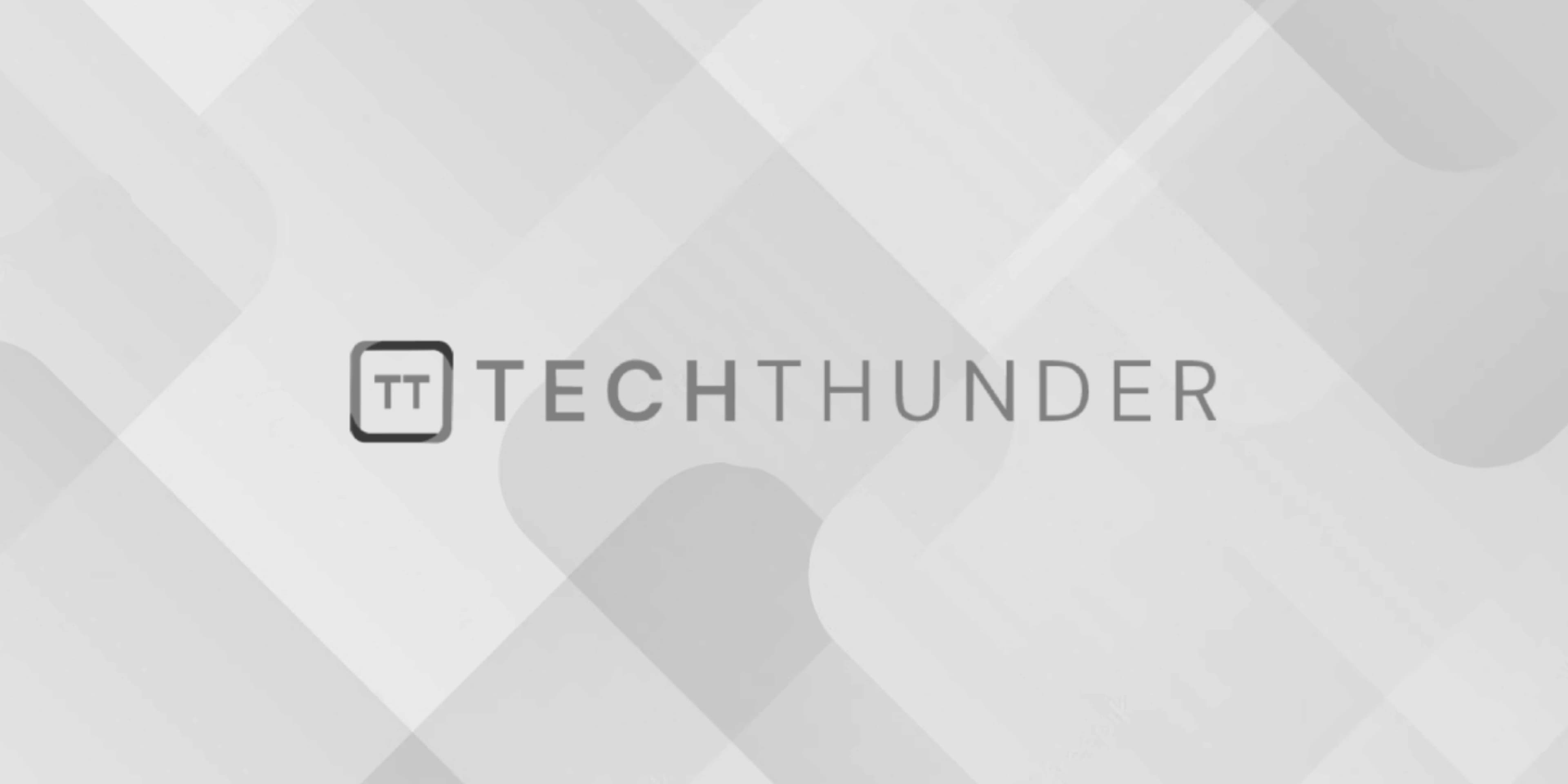
Jquery Search Slide out Plugin
The plugins allow you to create a search bar that can slide out from the top, bottom, left, or right of the page when triggered by a button or icon. The search bar typically overlays the content and provides a smooth sliding animation for a better user experience.
Please note that plugin availability and features may change over time, so it’s recommended to check the latest versions and documentation of the plugins before usage. Below is an example using the “SlideOutSearch” plugin, which provides a slide-out search bar from the top of the page:
HTML:
<!DOCTYPE html>
<html>
<head>
<title>Slide-Out Search Plugin</title>
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/5.15.4/css/all.min.css">
<link rel="stylesheet" href="slideoutsearch.css">
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script src="slideoutsearch.js"></script>
</head>
<body>
<!-- Your page content goes here -->
<!-- Search Icon -->
<div class="search-icon">
<i class="fas fa-search"></i>
</div>
<!-- Search Slide-Out -->
<div class="search-slideout">
<input type="text" placeholder="Search...">
<i class="fas fa-times close-btn"></i>
</div>
</body>
</html>
CSS (slideoutsearch.css):
/* Styles for Search Icon */
.search-icon {
position: fixed;
top: 20px;
right: 20px;
font-size: 24px;
cursor: pointer;
}
/* Styles for Search Slide-Out */
.search-slideout {
position: fixed;
top: 0;
left: 0;
width: 100%;
height: 60px;
background-color: #f1f1f1;
padding: 10px;
box-sizing: border-box;
display: none;
}
.search-slideout input[type="text"] {
width: 100%;
height: 40px;
border: none;
padding: 5px 10px;
font-size: 16px;
}
.search-slideout .close-btn {
float: right;
font-size: 20px;
cursor: pointer;
}
JavaScript (slideoutsearch.js):
$(document).ready(function() {
// Slide out search functionality
$('.search-icon').click(function() {
$('.search-slideout').slideDown();
});
$('.close-btn').click(function() {
$('.search-slideout').slideUp();
});
});
In this example, we have a search icon (Font Awesome search icon) at the top right corner of the page. When the user clicks on the search icon, the search bar slides down from the top of the page, allowing the user to input their search query. The search bar includes a close button (Font Awesome times icon) that can be used to slide the search bar back up when clicked.
Please ensure that you have the jQuery library, Font Awesome CSS, and the plugin CSS and JavaScript files correctly included in your project for this example to work. Additionally, there are many other jQuery plugins available that offer similar functionality, so feel free to explore and choose the one that best fits your requirements.