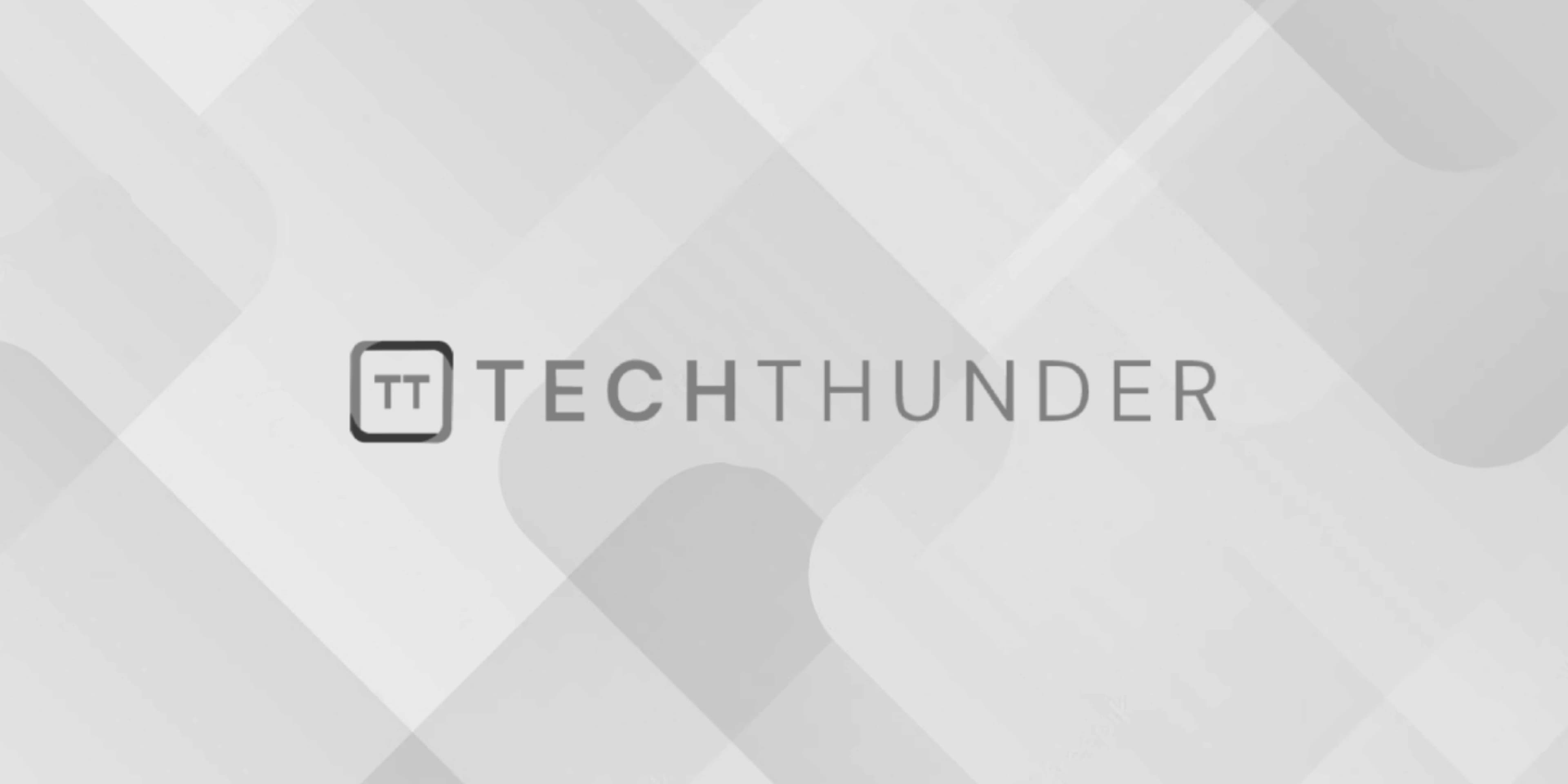
Dynamic Drag and Drop table rows using JQuery Ajax
To create a dynamic drag and drop functionality for table rows using jQuery and Ajax, you can follow these steps:
- Set up your HTML table with the necessary rows and columns.
- Use jQuery UI to make the table rows draggable and droppable.
- Implement Ajax to update the server with the new order of rows after dragging and dropping.
Here’s a step-by-step guide:
Step 1: HTML structure
<!DOCTYPE html>
<html>
<head>
<title>Dynamic Drag and Drop Table Rows</title>
<link rel="stylesheet" href="https://code.jquery.com/ui/1.12.1/themes/base/jquery-ui.css">
</head>
<body>
<table id="sortableTable">
<thead>
<tr>
<th>ID</th>
<th>Name</th>
<th>Age</th>
</tr>
</thead>
<tbody>
<tr data-id="1">
<td>1</td>
<td>John</td>
<td>30</td>
</tr>
<tr data-id="2">
<td>2</td>
<td>Jane</td>
<td>25</td>
</tr>
<!-- Add more table rows as needed -->
</tbody>
</table>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script src="https://code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
<script src="script.js"></script>
</body>
</html>
Step 2: JavaScript (script.js)
$(document).ready(function() {
$("#sortableTable tbody").sortable({
update: function(event, ui) {
saveNewOrder();
}
});
});
function saveNewOrder() {
var data = [];
$("#sortableTable tbody tr").each(function() {
var id = $(this).data("id");
data.push(id);
});
// Send the new order to the server using Ajax
$.ajax({
url: "updateOrder.php", // Replace with your server-side script to handle the new order
type: "POST",
data: { order: data },
success: function(response) {
// Handle the server response if needed
},
error: function(xhr, status, error) {
// Handle error if the request fails
}
});
}
Step 3: PHP (updateOrder.php)
<?php
// Handle the new order received from Ajax
if (isset($_POST['order'])) {
$newOrder = $_POST['order'];
// Update your database table with the new order
// Replace the code below with the code to update your database table
// Example code (for demonstration purposes only)
// Connect to the database and update the order field in the table
$host = 'localhost';
$username = 'your_username';
$password = 'your_password';
$database = 'your_database';
$connection = new mysqli($host, $username, $password, $database);
if ($connection->connect_error) {
die("Connection failed: " . $connection->connect_error);
}
foreach ($newOrder as $index => $id) {
$query = "UPDATE your_table_name SET order_column = $index WHERE id = $id";
$connection->query($query);
}
$connection->close();
}
?>
In this example, we use jQuery UI’s sortable()
function to make the table rows draggable and droppable. The update
event is triggered when the user finishes dragging and dropping a row, and it calls the saveNewOrder()
function to save the new order to the server using Ajax.
The saveNewOrder()
function collects the row IDs in the new order and sends them to the server-side PHP script using Ajax. The PHP script (updateOrder.php
) then updates the database table with the new order based on the received data.
Replace “your_table_name” with the name of your database table and “order_column” with the name of the column that stores the order of the rows in your table.
With this implementation, you can achieve a dynamic drag and drop functionality for table rows, and the new order will be saved to the server using Ajax and PHP.