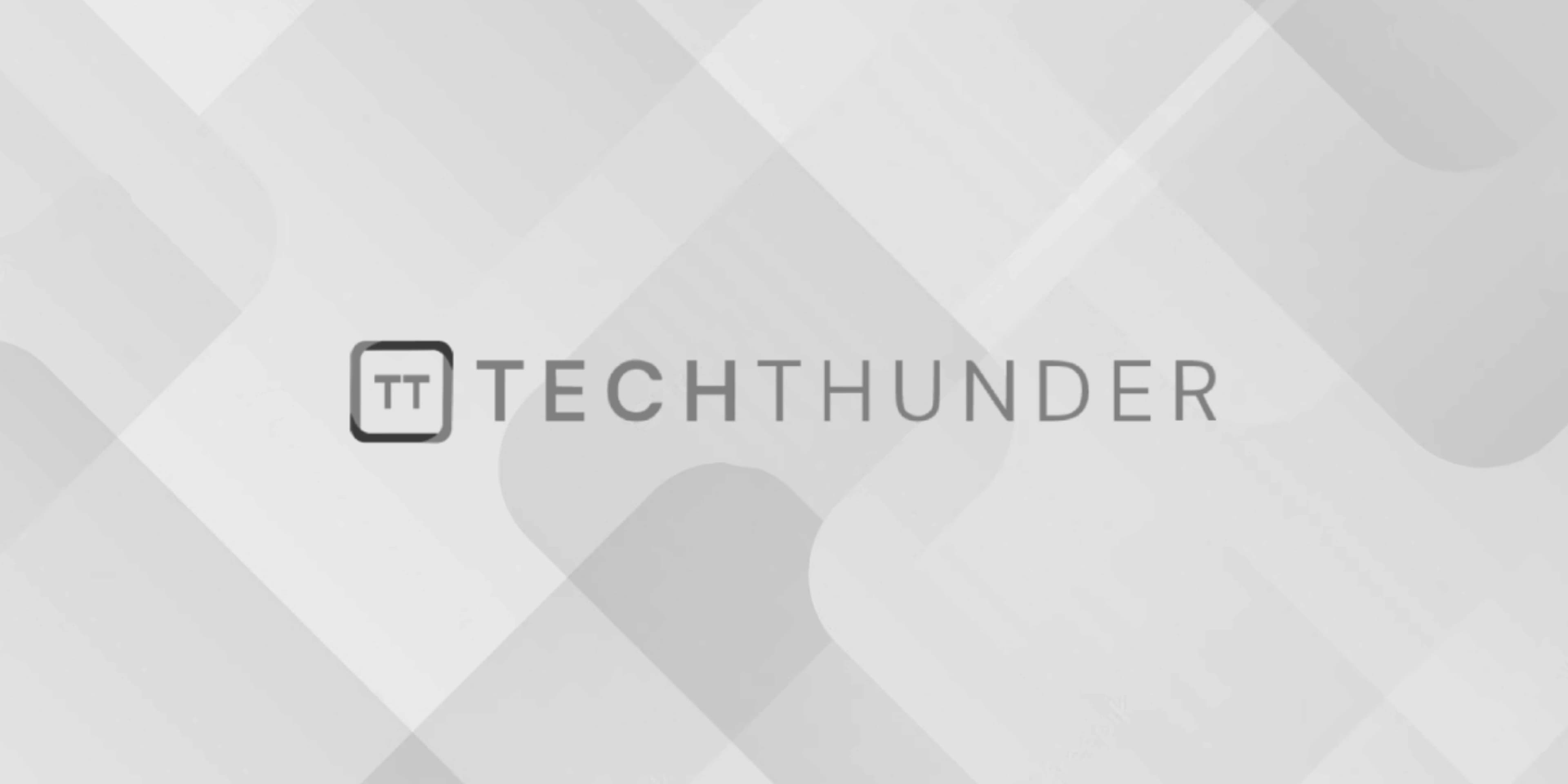
jQuery off() method
The jQuery off()
method is used to remove event handlers attached to elements using the on()
method. It allows you to unbind event handlers from specific elements or namespaces. When you use the off()
method to remove an event handler, the specified event will no longer trigger the associated function.
Here’s the basic syntax of the off()
method:
$(selector).off(eventType, handler)
Parameters:
selector
: A selector string or jQuery object representing the elements from which the event handlers will be removed.eventType
: (Optional) A string representing the type of event from which to remove the event handler (e.g., “click”, “change”, “mouseenter”, etc.). If not specified, all event handlers for the selected elements will be removed.handler
: (Optional) The specific event handler function to be removed. If not specified, all event handlers of the specified event type will be removed.
Example:
<!DOCTYPE html>
<html>
<head>
<title>jQuery off() Method Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<button id="myButton">Click Me</button>
<script>
// Define the click event handler function
function handleClick() {
alert("Button clicked!");
}
$(document).ready(function() {
// Attach the click event handler to the button
$("#myButton").on("click", handleClick);
// Remove the click event handler after 3 seconds
setTimeout(function() {
$("#myButton").off("click", handleClick);
}, 3000);
});
</script>
</body>
</html>
In this example, we have a button with the ID “myButton”. We attach a click event handler (handleClick
) to the button using the on()
method. After 3 seconds, we use the off()
method to remove the click event handler from the button. As a result, the click event will no longer trigger the handleClick
function after 3 seconds.
The off()
method allows you to manage event handlers dynamically and control when they should be active or inactive. It is particularly useful when you want to add and remove event handlers based on specific conditions or user interactions in your web application.