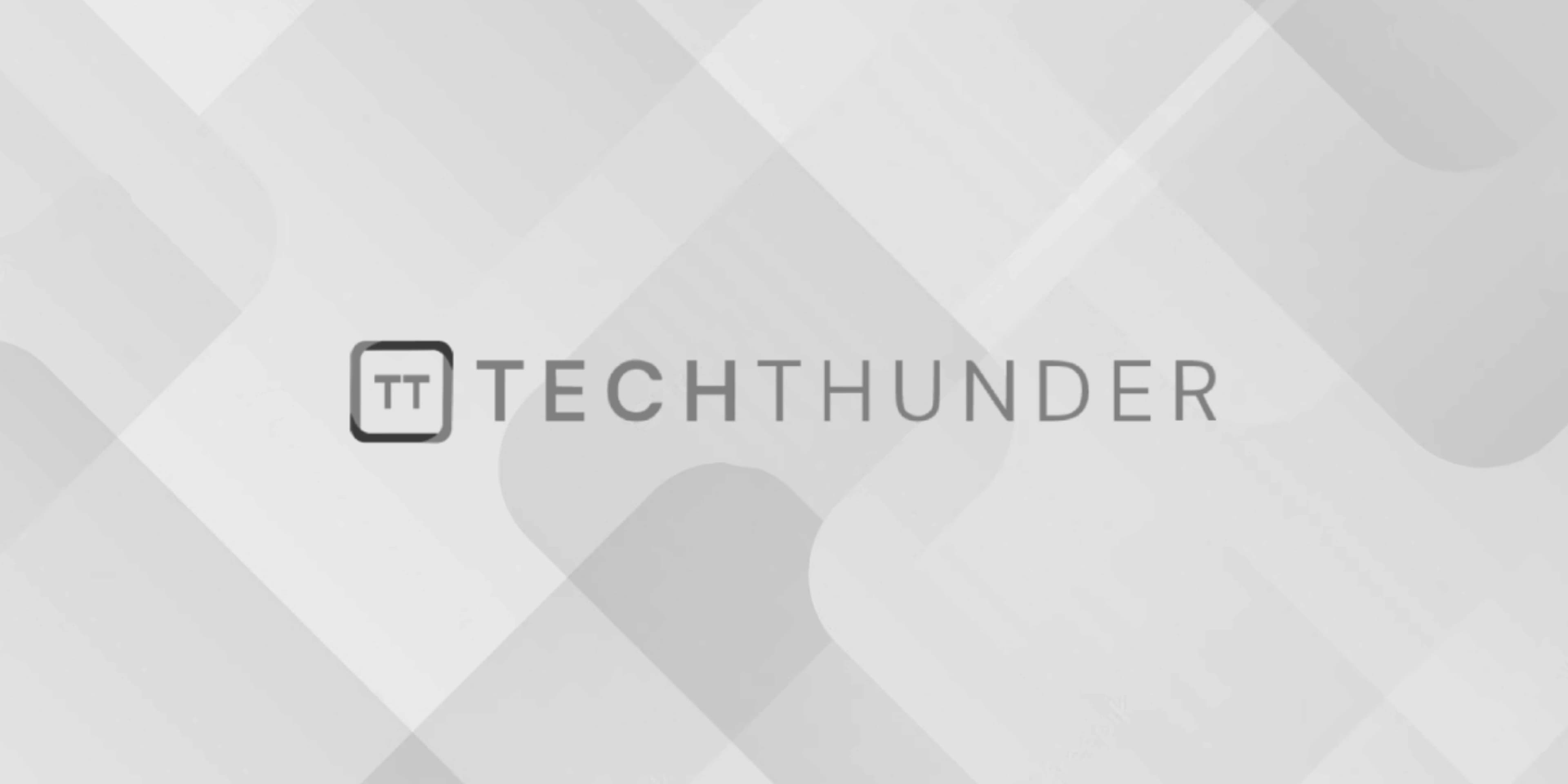
jQuery queue() method
The queue()
method in jQuery is used to manipulate the animation queue of elements selected by the jQuery object. The animation queue is a series of animations that are waiting to be executed on the selected elements.
Here’s the basic syntax of the queue()
method:
$(selector).queue([queueName], newQueue)
Parameters:
selector
: The selector for the elements to manipulate the animation queue.queueName
(optional): A string representing the name of the queue to manipulate. If not specified, the method will use the default “fx” queue, which is the standard animation queue.newQueue
(optional): An array of functions representing the new animation queue. If specified, it replaces the existing queue with the new one.
Return Value:
The queue()
method returns the selected jQuery object to support method chaining.
Example:
<!DOCTYPE html>
<html>
<head>
<title>jQuery queue() Method Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<style>
div {
width: 100px;
height: 100px;
background-color: red;
margin: 10px;
}
</style>
</head>
<body>
<div class="box"></div>
<button id="startAnimation">Start Animation</button>
<button id="clearQueue">Clear Queue</button>
<script>
$(document).ready(function() {
var $box = $(".box");
function startAnimation() {
// Add animations to the queue
$box.animate({ marginLeft: "200px" }, 2000);
$box.animate({ marginLeft: "0px" }, 2000);
}
function clearQueue() {
// Clear the animation queue
$box.queue("fx", []);
}
$("#startAnimation").click(function() {
startAnimation();
});
$("#clearQueue").click(function() {
clearQueue();
});
});
</script>
</body>
</html>
In this example, we have a red square (a div
element) and two buttons: “Start Animation” and “Clear Queue.” When the “Start Animation” button is clicked, the startAnimation()
function is called, which adds two animations to the animation queue using the animate()
method. The square moves to the right and then back to its original position.
When the “Clear Queue” button is clicked, the clearQueue()
function is called, which uses the queue()
method with the “fx” queue name and an empty array as the new queue. This effectively clears all the animations from the “fx” queue, stopping any ongoing animation and preventing future animations from executing.
The queue()
method can be helpful when you want to manage the animation flow, delay animations, or remove specific animations from the queue, providing you with more control over the animations applied to the selected elements.