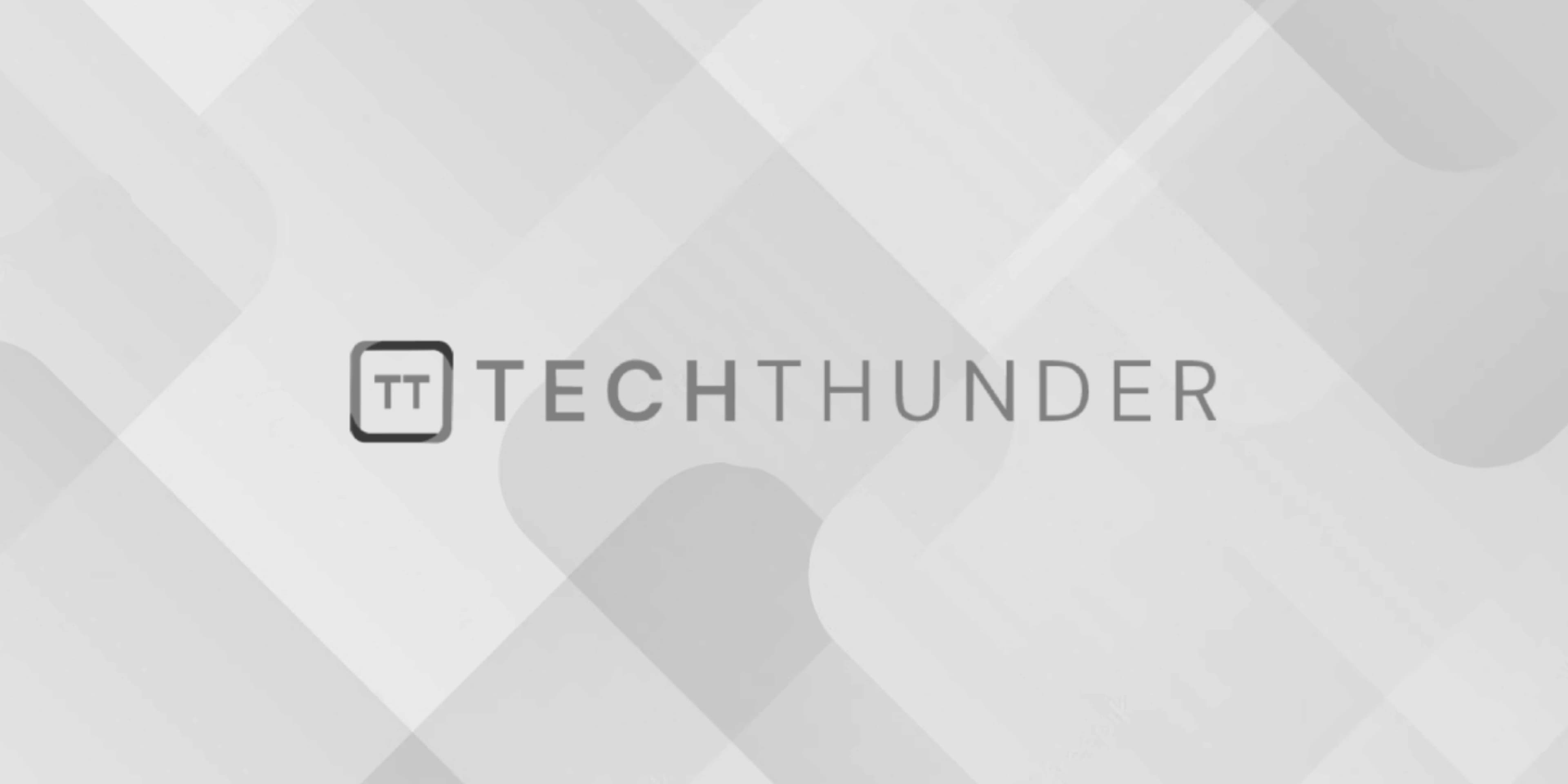
jQuery hasClass() method
The jQuery hasClass()
method is used to check if the selected elements have a specific CSS class or not. It returns true
if any of the matched elements have the given class, and false
otherwise.
The syntax for using the hasClass()
method is as follows:
$(selector).hasClass(className);
selector
: It is a string that specifies the elements to be selected.className
: It is a string representing the name of the CSS class you want to check for.
Here’s an example of how you can use the hasClass()
method:
HTML:
<div class="box highlight">This is a div element.</div>
JavaScript:
// Check if the div element has the class "highlight"
var hasHighlightClass = $('.box').hasClass('highlight');
console.log('Has "highlight" class: ' + hasHighlightClass);
In the above example, the hasClass()
method is used to check if the div
element with the class “box” has the class “highlight” or not. Since the div
element has both “box” and “highlight” classes, the result will be true
, and the message “Has ‘highlight’ class: true” will be logged to the console.
You can also use hasClass()
to check for multiple classes at once:
// Check if the element has either "class1" or "class2" or both
var hasClass1orClass2 = $('#myElement').hasClass('class1 class2');
The hasClass()
method is commonly used in conditional statements to apply certain actions or styles based on whether an element has a specific class. For example:
// Apply different styles based on the presence of the "highlight" class
if ($('#myElement').hasClass('highlight')) {
// Do something when the "highlight" class is present
$('#myElement').css('color', 'red');
} else {
// Do something when the "highlight" class is not present
$('#myElement').css('color', 'blue');
}
In this example, if the element with the ID “myElement” has the class “highlight,” the text color will be set to red. Otherwise, it will be set to blue.
The hasClass()
method is a handy way to conditionally apply logic based on the presence of classes in your HTML elements and is often used in combination with other jQuery methods and event handlers to create interactive and dynamic web pages.