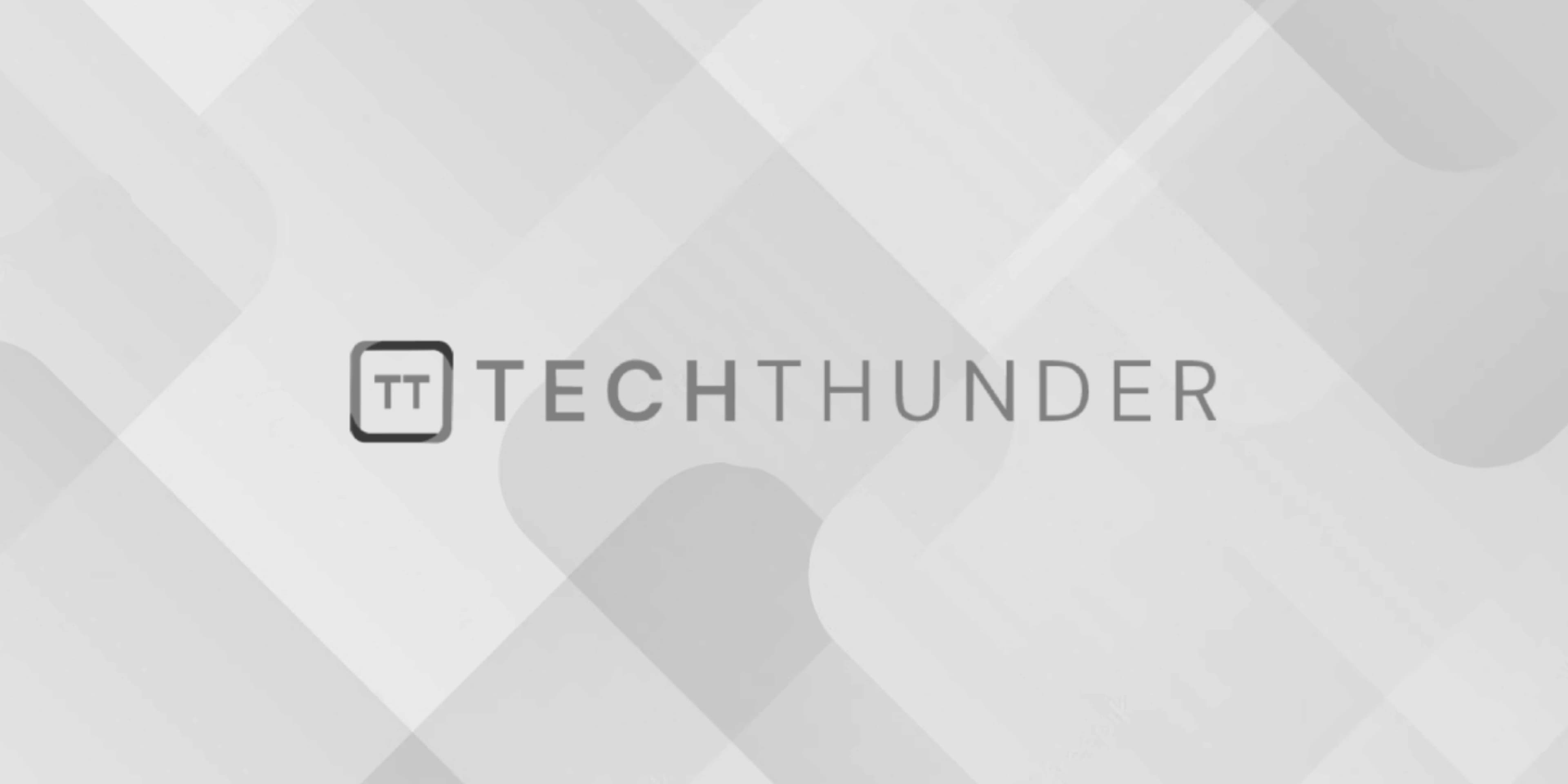
Use of moment JS to change date format in jquery
Moment.js is a popular JavaScript library used for parsing, manipulating, and formatting dates and times. To change the date format using Moment.js in jQuery, you need to include the Moment.js library and then use its functions to format the date according to your desired format.
Here’s a simple example to demonstrate how to change the date format using Moment.js in jQuery:
HTML:
<!DOCTYPE html>
<html>
<head>
<title>Change Date Format using Moment.js</title>
</head>
<body>
<p>Original date: <span id="originalDate">2023-07-27T12:34:56</span></p>
<p>Formatted date: <span id="formattedDate"></span></p>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<!-- Link to Moment.js library -->
<script src="https://cdnjs.cloudflare.com/ajax/libs/moment.js/2.29.1/moment.min.js"></script>
<script src="script.js"></script>
</body>
</html>
JavaScript (script.js):
$(document).ready(function() {
// Get the original date from the HTML
var originalDate = $('#originalDate').text();
// Use Moment.js to format the date
var formattedDate = moment(originalDate).format('YYYY-MM-DD');
// Display the formatted date
$('#formattedDate').text(formattedDate);
});
In this example, the moment()
function from the Moment.js library is used to parse the original date from the HTML (2023-07-27T12:34:56
). The format()
function is then used to format the date according to the desired format (YYYY-MM-DD
). The formatted date is then displayed in the #formattedDate
element.
The result will be:
Original date: 2023-07-27T12:34:56
Formatted date: 2023-07-27
You can change the format string in the format()
function to customize the date format according to your requirements. Moment.js provides a wide range of formatting options, allowing you to display dates in various formats like “MM/DD/YYYY”, “DD/MM/YYYY”, “YYYY-MM-DD HH:mm:ss”, and so on. Refer to the Moment.js documentation for more information on date formatting options.