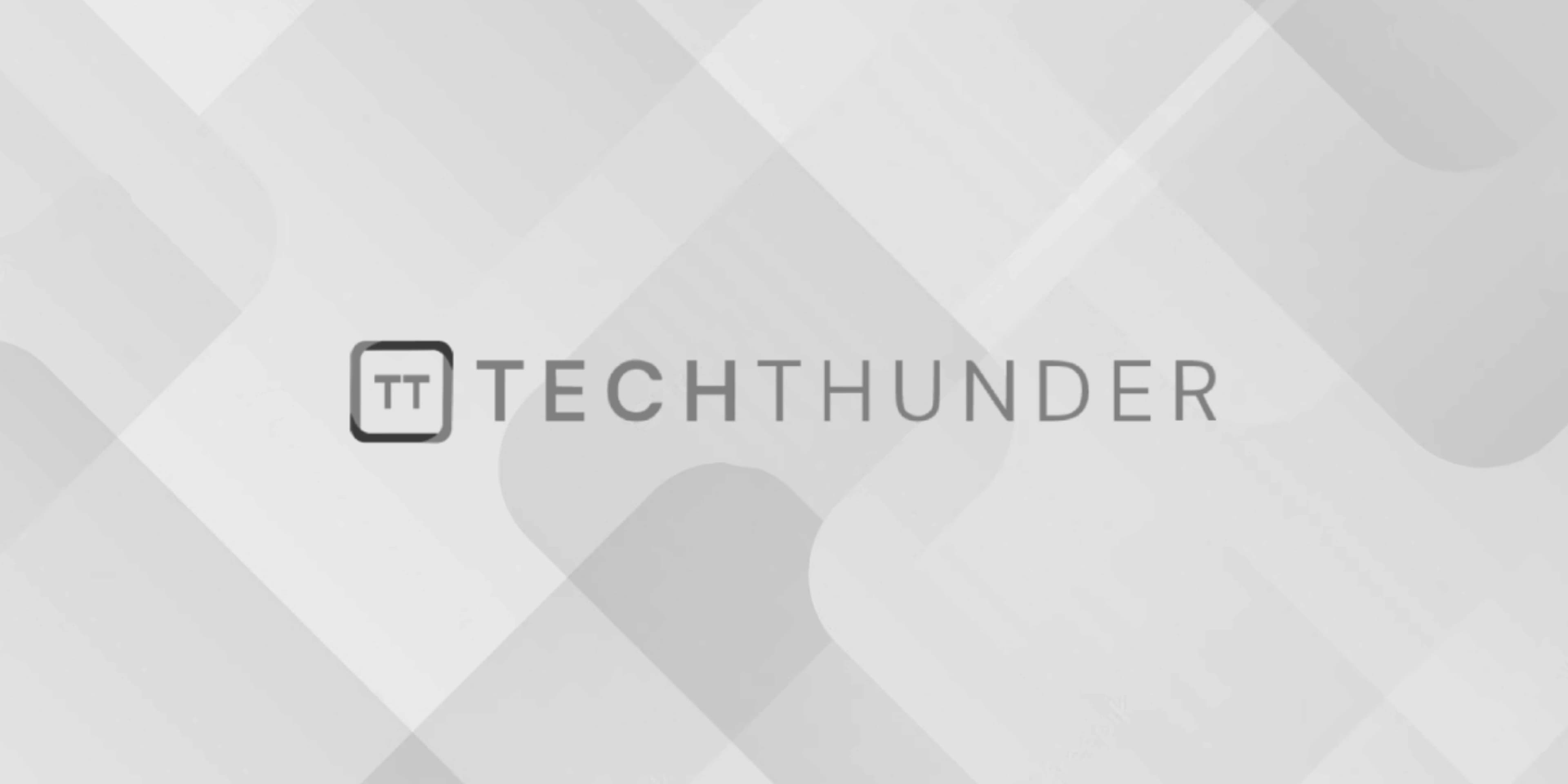
jQuery resize() method
The jQuery resize()
method is an event handler that is used to bind a function to the “resize” event of the window or an HTML element. The “resize” event is triggered when the size of the browser window or the specified element is changed, either by resizing the window or changing its dimensions through CSS or JavaScript.
Here’s the basic syntax of the resize()
method:
$(window).resize(function() {
// Code to be executed when the window is resized
});
// or for a specific HTML element
$(element).resize(function() {
// Code to be executed when the element is resized
});
Parameters:
- The
resize()
method takes a function as its argument, which will be executed when the “resize” event occurs.
Example:
<!DOCTYPE html>
<html>
<head>
<title>jQuery resize() Method Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<div id="box"></div>
<script>
$(document).ready(function() {
// Resize event handler for the window
$(window).resize(function() {
console.log("Window is resized.");
});
// Resize event handler for the specific element
$("#box").resize(function() {
console.log("Box is resized.");
});
});
</script>
<style>
#box {
width: 200px;
height: 200px;
background-color: red;
}
</style>
</body>
</html>
In this example, we have two resize event handlers. The first one is bound to the “resize” event of the window, and the second one is bound to the “resize” event of a <div>
element with the ID “box.” When the browser window is resized, the message “Window is resized.” will be logged to the console. When the “box” element is resized (by changing its width or height), the message “Box is resized.” will be logged to the console.
Note: The “resize” event for elements other than the window is not supported in jQuery by default. However, you can trigger the “resize” event manually using JavaScript when you want to detect changes to the size of a specific element.
The resize()
method is useful when you want to perform actions or update the layout of your web page in response to changes in the size of the window or specific elements. It is commonly used for responsive design or for adjusting the layout of elements based on the available screen space.