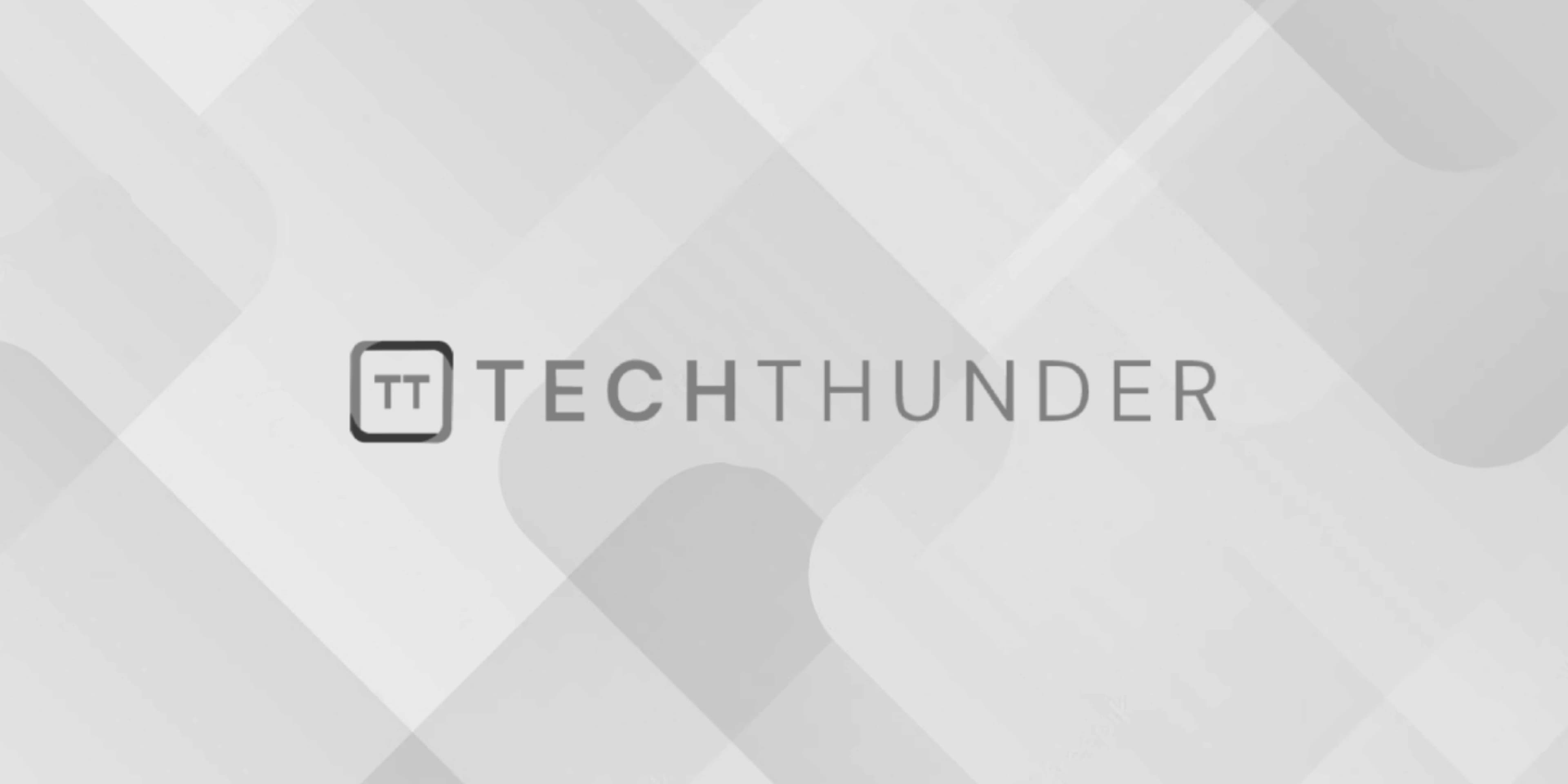
Gauge chart plugin using jQuery
The Gauge.js provides a simple and lightweight solution to create animated and customizable gauge charts to display data in a visually appealing way.
To use “Gauge.js” in your project, follow these steps:
Step 1: Include Dependencies
Download the “Gauge.js” library from the official website (https://github.com/bernii/gauge.js) or use a CDN link. Include the required CSS and JavaScript files in your HTML file:
<!DOCTYPE html>
<html>
<head>
<title>Gauge Chart using Gauge.js</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/gauge.js/1.3.6/gauge.min.js"></script>
</head>
<body>
<!-- Your content goes here -->
<canvas id="gaugeChart"></canvas>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script src="script.js"></script>
</body>
</html>
Step 2: Initialize Gauge Chart
In your JavaScript file or in a <script>
tag on your HTML page, use “Gauge.js” to create and customize the gauge chart:
$(document).ready(function() {
var opts = {
angle: -0.2, // The starting angle of the gauge (in radians)
lineWidth: 0.2, // The width of the gauge arc
radiusScale: 1, // Scale the size of the gauge
pointer: {
length: 0.6, // The length of the gauge needle (0-1)
strokeWidth: 0.035, // The width of the gauge needle
color: '#000000' // The color of the gauge needle
},
percentColors: [[0.0, "#ff0000"], [0.50, "#ffa500"], [1.0, "#00ff00"]], // Customize colors based on the gauge value
generateGradient: true, // Generate a gradient for the gauge arc
staticZones: [
{strokeStyle: "#f03e3e", min: 0, max: 30}, // Red from 0 to 30
{strokeStyle: "#ffad00", min: 30, max: 70}, // Yellow from 30 to 70
{strokeStyle: "#30b32d", min: 70, max: 100} // Green from 70 to 100
],
highDpiSupport: true // Enable high DPI support for better rendering on retina displays
};
var target = document.getElementById('gaugeChart'); // The canvas element to render the gauge
var gauge = new Gauge(target).setOptions(opts); // Create the gauge chart
// Set the initial value for the gauge (e.g., 50)
gauge.set(50);
});
In this example, we’ve used the “Gauge.js” library to create a gauge chart with a custom needle color and three color zones (red, yellow, green) based on the gauge value. The gauge chart starts at an angle of -0.2 radians and has a line width of 0.2. You can customize these options based on your requirements.
Please note that “Gauge.js” is not a jQuery-specific plugin, but it can be used with jQuery and other JavaScript libraries. Always ensure to use plugins from reputable sources and check their documentation for usage instructions and supported options. Additionally, consider browser compatibility and performance optimizations when using any third-party library in your project.