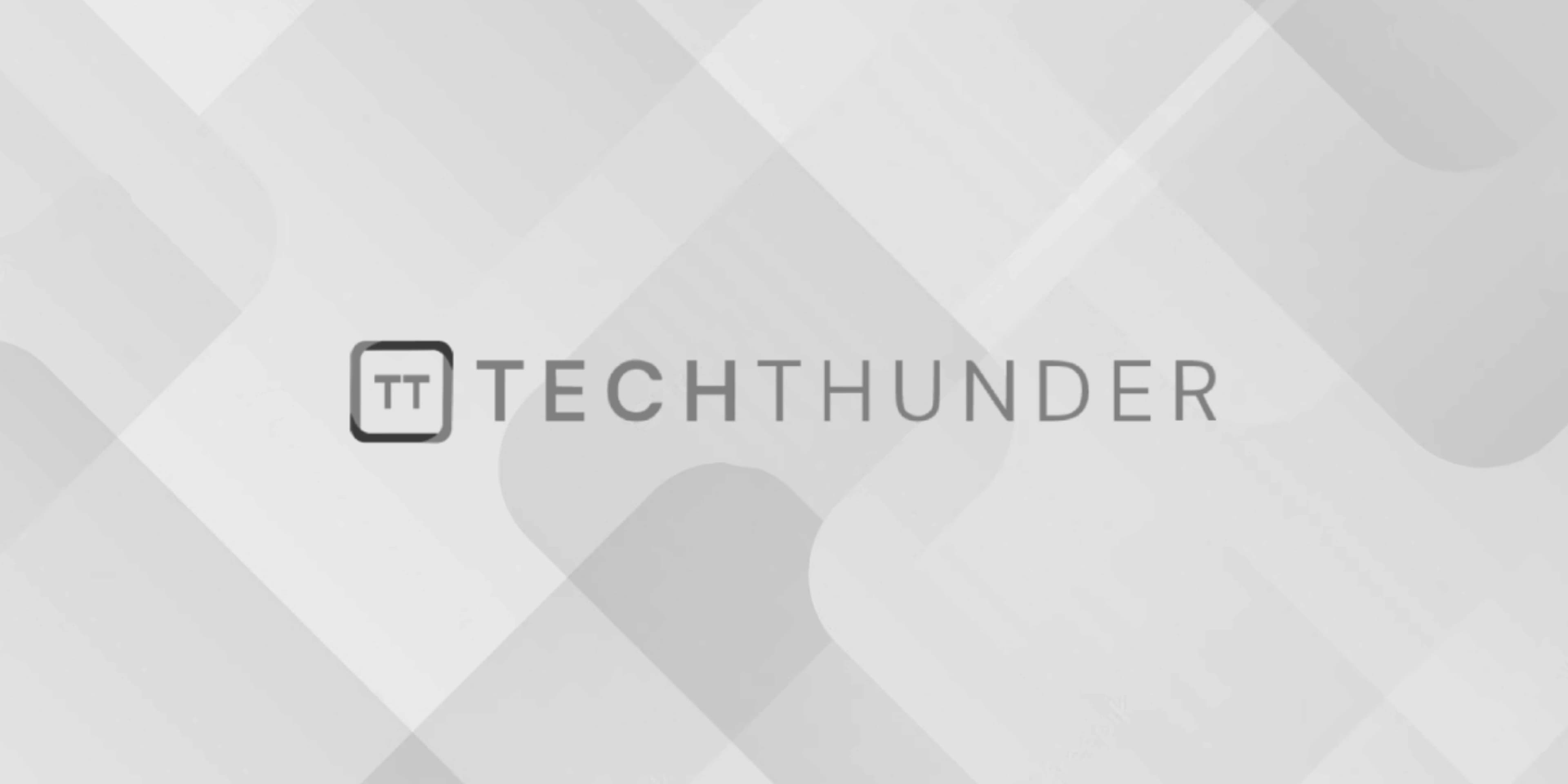
Create Tic TAC Toe Game using jQuery
Creating a Tic Tac Toe game using jQuery is a fun project that involves handling user clicks, checking for a winner, and managing the game state. Here’s a step-by-step guide to building a simple Tic Tac Toe game:
HTML:
<!DOCTYPE html>
<html>
<head>
<title>Tic Tac Toe</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div class="board">
<div class="cell" data-id="0"></div>
<div class="cell" data-id="1"></div>
<div class="cell" data-id="2"></div>
<div class="cell" data-id="3"></div>
<div class="cell" data-id="4"></div>
<div class="cell" data-id="5"></div>
<div class="cell" data-id="6"></div>
<div class="cell" data-id="7"></div>
<div class="cell" data-id="8"></div>
</div>
<div id="result"></div>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script src="script.js"></script>
</body>
</html>
CSS (styles.css):
body {
font-family: Arial, sans-serif;
text-align: center;
}
.board {
display: grid;
grid-template-columns: repeat(3, 100px);
grid-gap: 2px;
margin: 50px auto;
}
.cell {
width: 100px;
height: 100px;
display: flex;
justify-content: center;
align-items: center;
font-size: 2rem;
background-color: #f2f2f2;
cursor: pointer;
}
#result {
font-size: 1.5rem;
font-weight: bold;
margin-top: 20px;
}
JavaScript (script.js):
$(document).ready(function() {
let currentPlayer = 'X';
let board = ['', '', '', '', '', '', '', '', ''];
$('.cell').on('click', function() {
const cellId = $(this).data('id');
if (board[cellId] === '' && !isGameOver()) {
board[cellId] = currentPlayer;
$(this).text(currentPlayer);
if (isWinner(currentPlayer)) {
$('#result').text(`${currentPlayer} wins!`);
} else if (isTie()) {
$('#result').text('It\'s a tie!');
} else {
currentPlayer = currentPlayer === 'X' ? 'O' : 'X';
}
}
});
function isWinner(player) {
const winningCombinations = [
[0, 1, 2], [3, 4, 5], [6, 7, 8], // Rows
[0, 3, 6], [1, 4, 7], [2, 5, 8], // Columns
[0, 4, 8], [2, 4, 6] // Diagonals
];
return winningCombinations.some(combination => {
return combination.every(index => board[index] === player);
});
}
function isTie() {
return board.every(cell => cell !== '');
}
function isGameOver() {
return isWinner('X') || isWinner('O') || isTie();
}
});
In this example, we create a simple Tic Tac Toe game with an HTML grid representing the game board. Each cell in the grid is a clickable element that corresponds to a position on the board. The game state is stored in the board
array, and the current player (either ‘X’ or ‘O’) is stored in the currentPlayer
variable.
When a player clicks on a cell, the corresponding position on the board is updated with the player’s symbol (‘X’ or ‘O’). The game checks for a winner or a tie after each move and displays the result in the #result
element. The game continues until a player wins or the game ends in a tie.
The CSS styles the game board and result message to make it visually appealing.
When you open the HTML file in your browser, you can play the Tic Tac Toe game by clicking on the cells. The game will display the result once a player wins or the game ends in a tie.