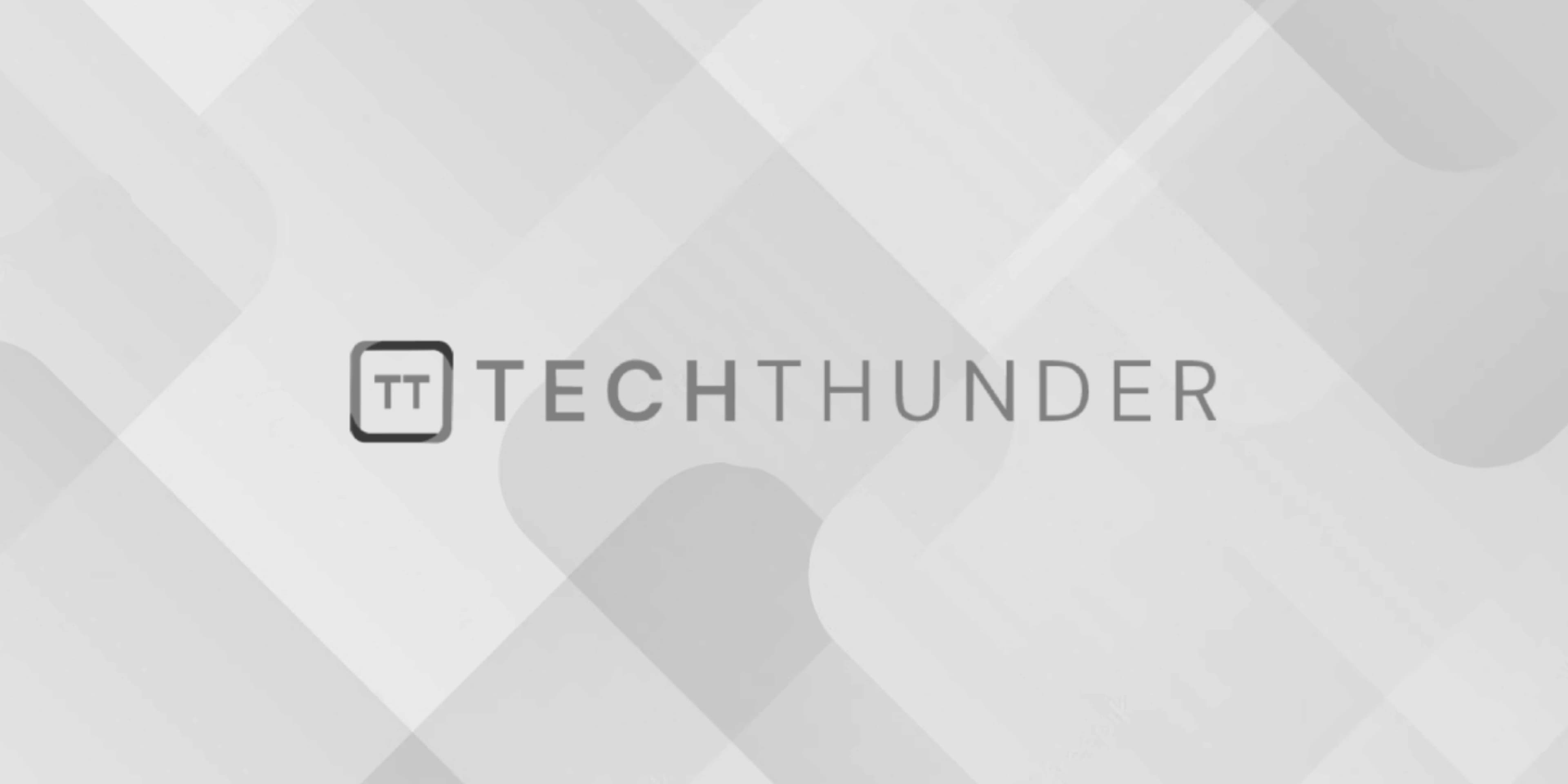
jQuery Timepicker
To create a timepicker using jQuery, you can use the “jQuery UI Timepicker” plugin, which extends the jQuery UI Datepicker to include a time input field. This plugin allows users to select a time value easily and supports various time formats and options.
Here’s a step-by-step guide on how to implement a timepicker using the “jQuery UI Timepicker” plugin:
Step 1: Include the necessary CSS and JavaScript files.
First, you need to include the jQuery library, jQuery UI library, and the “jQuery UI Timepicker” plugin.
<!DOCTYPE html>
<html>
<head>
<title>jQuery Timepicker Example</title>
<link rel="stylesheet" href="https://code.jquery.com/ui/1.12.1/themes/base/jquery-ui.css">
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/jquery-timepicker/1.13.18/jquery.timepicker.min.css">
</head>
<body>
<input type="text" id="timepicker">
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script src="https://code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery-timepicker/1.13.18/jquery.timepicker.min.js"></script>
<script src="script.js"></script>
</body>
</html>
Step 2: Initialize the timepicker using jQuery.
In your JavaScript file (script.js
), you can initialize the timepicker for the input field with the timepicker()
method.
$(document).ready(function() {
$('#timepicker').timepicker();
});
Step 3: Customize the Timepicker (Optional).
You can customize the timepicker by providing various options and settings when initializing it. For example:
$(document).ready(function() {
$('#timepicker').timepicker({
timeFormat: 'HH:mm', // Set the time format (24-hour format)
interval: 15, // Set the interval between times in minutes
minTime: '08:00', // Set the minimum time that can be selected
maxTime: '20:00', // Set the maximum time that can be selected
startTime: '09:00', // Set the default time
dynamic: false, // Disable dynamic mode (changes time options based on selection)
dropdown: true, // Use a dropdown instead of a slider
scrollbar: true // Show a scrollbar for the dropdown
// Add more options as needed
});
});
With the “jQuery UI Timepicker” plugin, you can easily create a timepicker that enhances user experience when selecting time values. The timepicker provides various customizable options to suit your specific requirements.