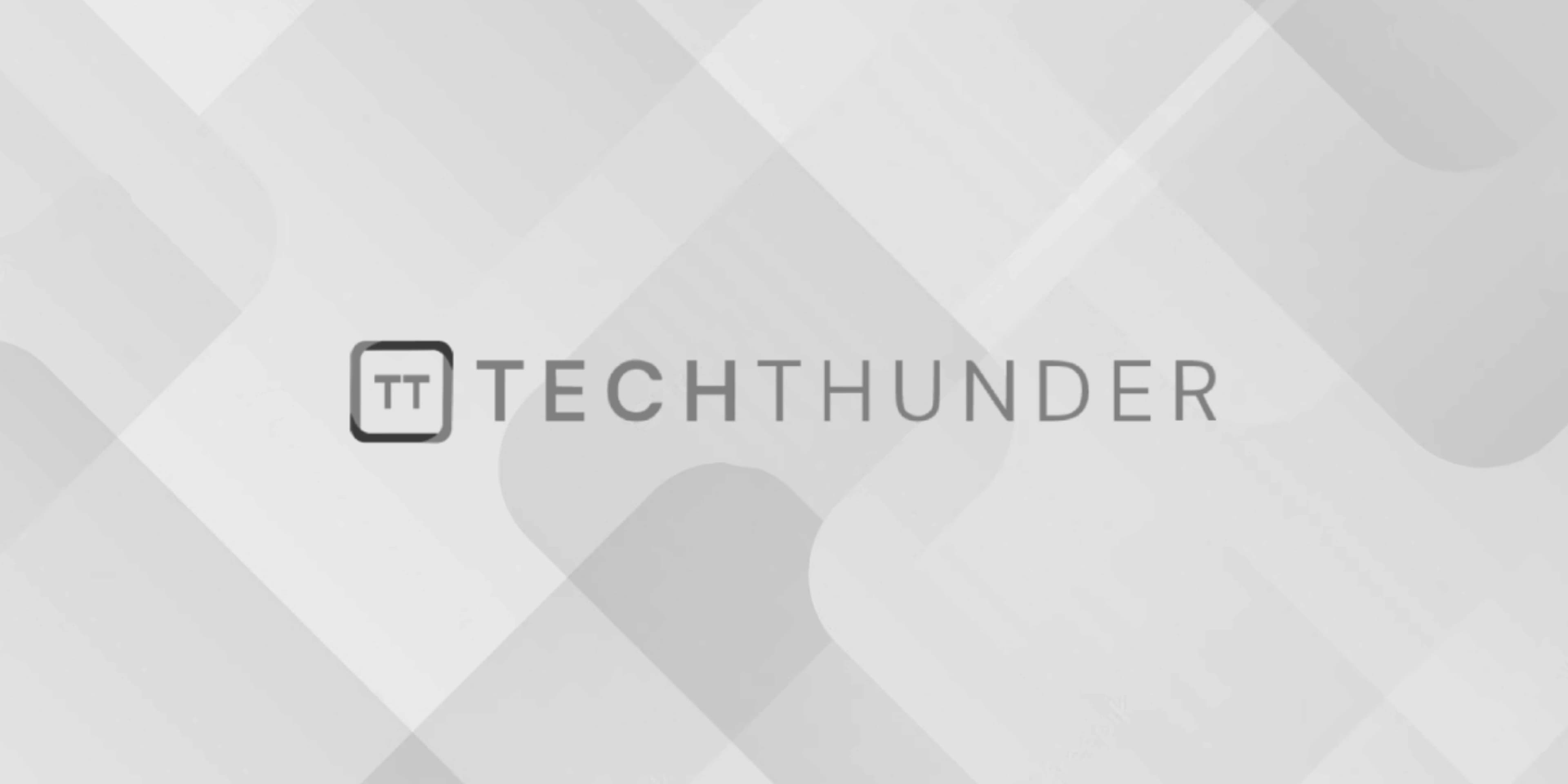
jQuery :hidden selector
The :hidden
selector in jQuery is used to select all elements that are currently hidden on a web page. An element is considered hidden if it has either of the following properties:
- It has a
display
property set to “none”. - It is an input element with the
type
attribute set to “hidden”. - It has a
width
and/orheight
set to 0, or its content overflows and is not visible.
Here’s the basic syntax of the :hidden
selector:
$(":hidden")
This selector can be used to manipulate or filter hidden elements in various ways, such as showing or hiding them, performing operations on them, or accessing their attributes and content.
Example:
<!DOCTYPE html>
<html>
<head>
<title>jQuery :hidden Selector Example</title>
<style>
.hidden-div {
display: none;
}
.zero-dimension-div {
width: 0;
height: 0;
}
</style>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<div class="hidden-div">This div is hidden using display: none.</div>
<input type="hidden" value="Hidden Input">
<div class="zero-dimension-div">This div has zero dimensions (width: 0, height: 0).</div>
<button id="showHidden">Show Hidden Elements</button>
<script>
$(document).ready(function() {
$('#showHidden').click(function() {
// Select all hidden elements and show them
$(":hidden").show();
});
});
</script>
</body>
</html>
In this example, we have three hidden elements: a <div>
with the class hidden-div
(hidden using display: none
), a hidden input element (<input type="hidden">
), and a <div>
with the class zero-dimension-div
(hidden because its dimensions are set to 0).
When you click the “Show Hidden Elements” button, the :hidden
selector is used to select all the hidden elements, and the show()
method is applied to them, making them visible on the page.
Please note that elements hidden with visibility: hidden
are not considered hidden by the :hidden
selector, as they still occupy space on the page. Only elements that are completely removed from the document flow (with display: none
or zero dimensions) will be matched by the :hidden
selector.