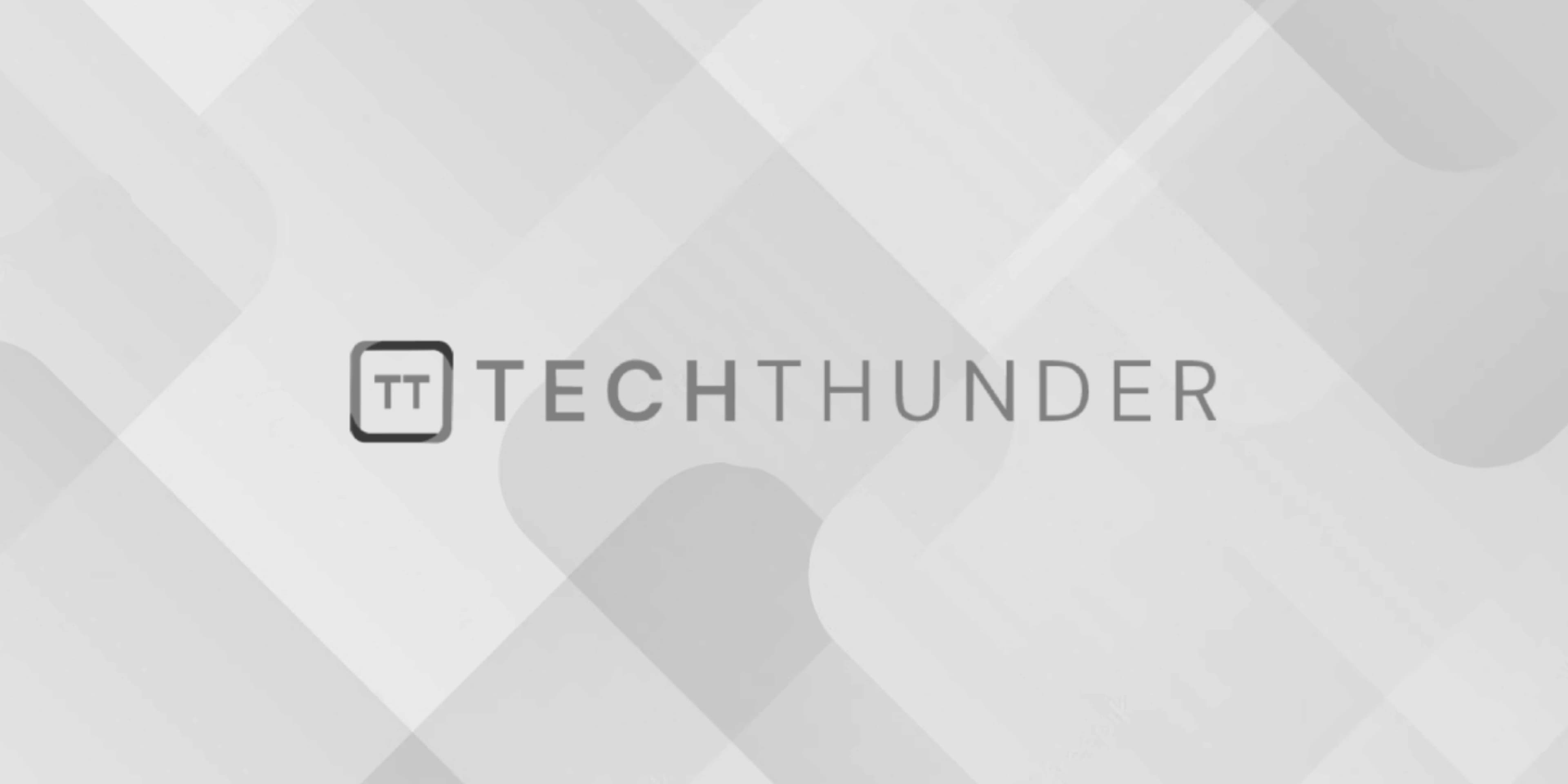
How to Create Custom Image Magnifier using jQuery
To create a custom image magnifier using jQuery, we can use a combination of HTML, CSS, and JavaScript (jQuery). The magnifier will display a zoomed-in version of the image when the user hovers over it. Here’s a step-by-step guide to creating a simple image magnifier:
HTML:
<!DOCTYPE html>
<html>
<head>
<title>Custom Image Magnifier</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div class="magnifier-container">
<div class="image-container">
<img src="image.jpg" alt="Image">
<div class="magnifier"></div>
</div>
</div>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script src="script.js"></script>
</body>
</html>
CSS (styles.css):
body {
font-family: Arial, sans-serif;
text-align: center;
margin: 0;
}
.magnifier-container {
max-width: 600px;
margin: 50px auto;
}
.image-container {
position: relative;
overflow: hidden;
}
img {
width: 100%;
height: auto;
}
.magnifier {
position: absolute;
border: 2px solid #ccc;
width: 200px;
height: 200px;
display: none;
pointer-events: none;
background-repeat: no-repeat;
background-size: 100% 100%;
z-index: 1;
}
JavaScript (script.js):
$(document).ready(function() {
$('.image-container').on('mousemove', function(e) {
const magnifier = $(this).find('.magnifier');
const image = $(this).find('img');
const magnifierSize = 200;
const containerOffset = $(this).offset();
const mouseX = e.pageX - containerOffset.left;
const mouseY = e.pageY - containerOffset.top;
const imageWidth = image.width();
const imageHeight = image.height();
const offsetX = -(mouseX / imageWidth) * (imageWidth - magnifierSize);
const offsetY = -(mouseY / imageHeight) * (imageHeight - magnifierSize);
magnifier.css({
left: mouseX - magnifierSize / 2,
top: mouseY - magnifierSize / 2,
backgroundPosition: `${offsetX}px ${offsetY}px`,
});
magnifier.show();
}).on('mouseleave', function() {
$(this).find('.magnifier').hide();
});
});
We create a custom image magnifier. When the user hovers over the image, the magnifier div is displayed at the mouse position, showing the zoomed-in version of the image. When the user moves the mouse within the image, the magnifier moves along, displaying the corresponding zoomed-in part of the image. To use this code, replace “image.jpg” with the path to your desired image.
You will have a simple custom image magnifier using jQuery. You can further customize the CSS to adjust the size and appearance of the magnifier. Additionally, you can add more images and magnifiers on the same page or use it as a starting point to implement more advanced image magnification features.