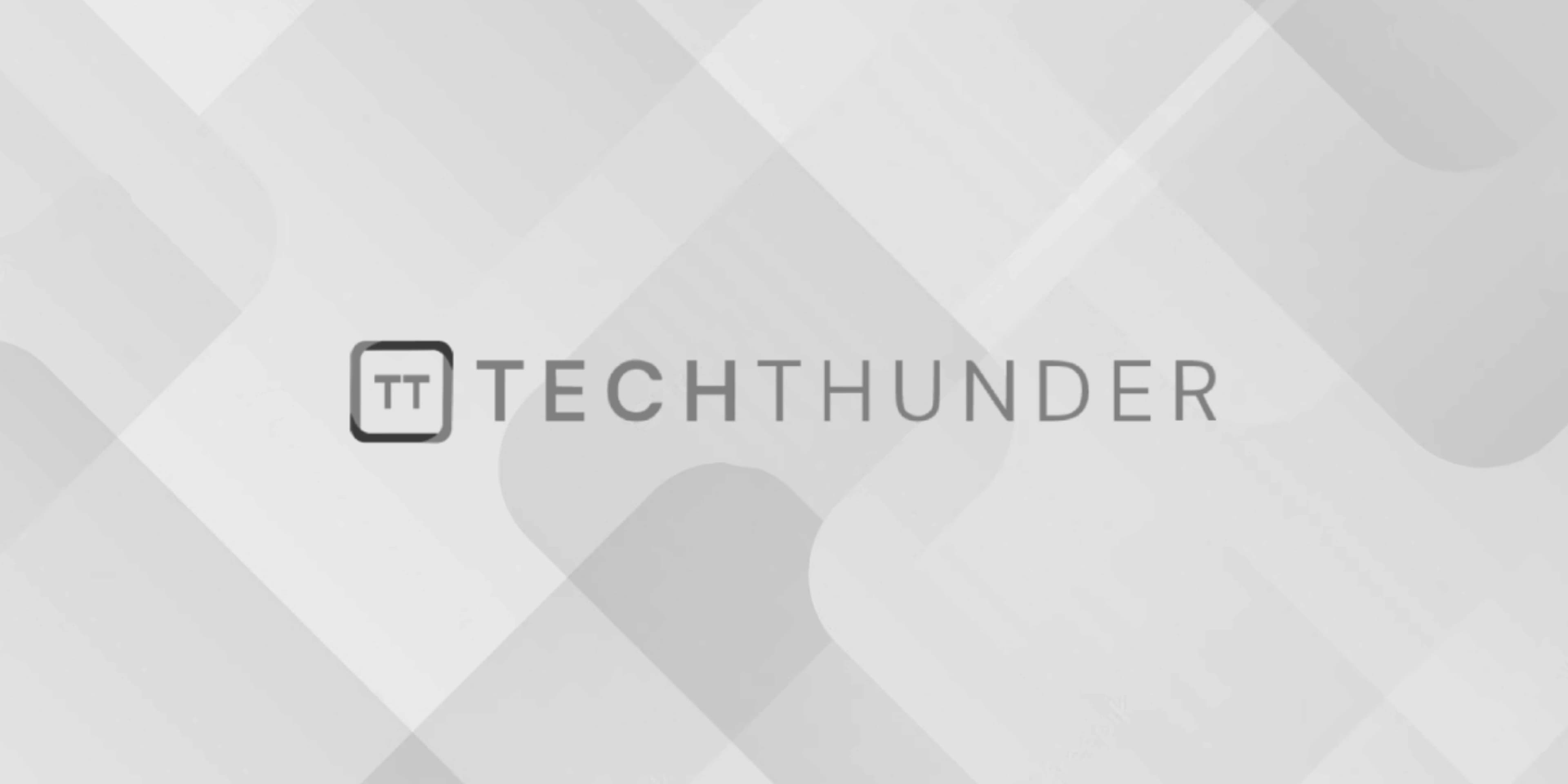
jQuery isFunction() method
The jQuery.isFunction()
method is a utility function in jQuery used to check whether a given value is a function or not. It is designed to provide a consistent way of determining if a variable is a function, even in older browsers where the native typeof
operator may not be sufficient for accurate function detection.
Here’s the basic syntax of the jQuery.isFunction()
method:
jQuery.isFunction(obj)
Parameters:
obj
: The variable or value to be tested.
Return Value:
The jQuery.isFunction()
method returns true
if the specified value is a function, and false
otherwise.
Example:
<!DOCTYPE html>
<html>
<head>
<title>jQuery isFunction() Method Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<script>
$(document).ready(function() {
var myFunction = function() {
alert("Hello, I am a function!");
};
var myString = "Hello, world!";
// Check if the variables are functions
var isMyFunction = jQuery.isFunction(myFunction);
var isMyStringFunction = jQuery.isFunction(myString);
// Log the results to the console
console.log("myFunction is a function:", isMyFunction); // true
console.log("myString is a function:", isMyStringFunction); // false
});
</script>
</body>
</html>
In this example, we have two variables: myFunction
and myString
. We use the jQuery.isFunction()
method to check whether each of these variables is a function or not. The method returns true
for the myFunction
variable because it is a function, and false
for the myString
variable because it is not a function.
Keep in mind that starting from jQuery version 3.0, the recommended way to check if a variable is a function is to use the native typeof
operator:
typeof obj === 'function'
Using typeof
for function detection is more widely supported in modern browsers and is the standard way to check for function types in JavaScript.
For modern projects, it is generally preferable to use the typeof
approach for better compatibility and performance. However, the jQuery.isFunction()
method remains available in jQuery for backward compatibility with older codebases or specific use cases.