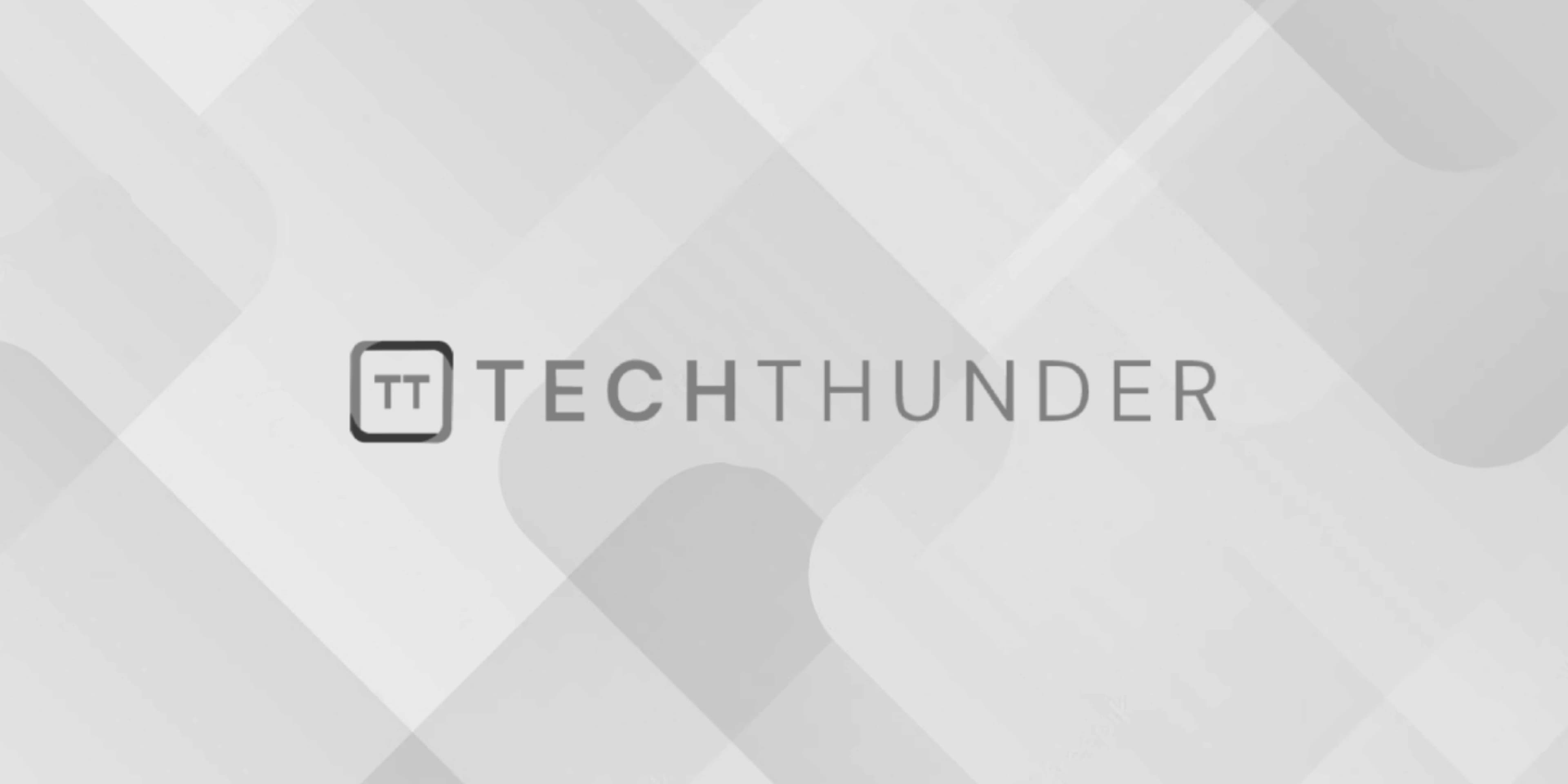
jQuery :gt() selector
The jQuery :gt()
selector is used to select all elements at an index greater than the specified index within a matched set of elements. It allows you to filter elements based on their position in the selection.
Here’s the basic syntax of the :gt()
selector:
$(selector:gt(index))
Parameters:
selector
: A selector expression used to select the elements you want to filter.index
: An integer value representing the minimum index for the selection. Elements at an index greater than this value will be included in the result.
Return Value:
The :gt()
selector returns a jQuery object containing the elements that match the condition based on their index.
Example:
<!DOCTYPE html>
<html>
<head>
<title>jQuery :gt() Selector Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<ul>
<li>Item 1</li>
<li>Item 2</li>
<li>Item 3</li>
<li>Item 4</li>
<li>Item 5</li>
</ul>
<script>
$(document).ready(function() {
// Select elements with an index greater than 2 (excluding index 2)
var selectedItems = $("li:gt(2)");
// Add a class to the selected elements for styling
selectedItems.addClass("selected");
});
</script>
<style>
.selected {
background-color: yellow;
}
</style>
</body>
</html>
In this example, we have an unordered list (<ul>
) containing five list items (<li>
). We use the :gt()
selector to select list items with an index greater than 2 (excluding the item at index 2). We then add a class called “selected” to these items to apply a yellow background to them.
When you run the code, you will see that the last two list items are highlighted with a yellow background.
The :gt()
selector is helpful when you want to target specific elements based on their position in the DOM. It allows you to select elements from a specific index onwards, providing you with fine-grained control over which elements to manipulate or style.