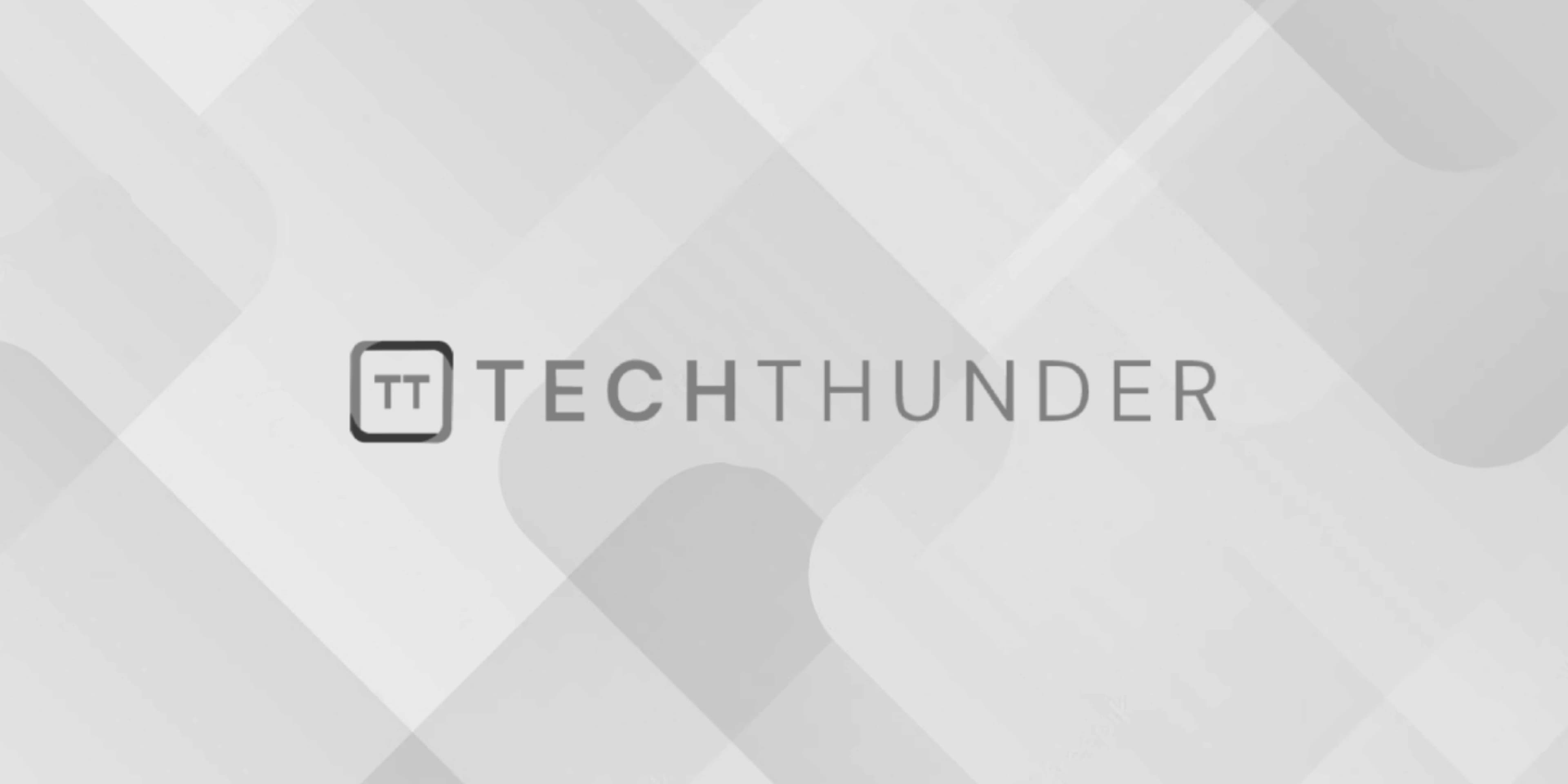
How to create simple map using jQuery
To create a simple map using jQuery, we can utilize the Google Maps JavaScript API, which allows us to embed maps and add markers and other elements to it. To use the Google Maps API, you will need to obtain an API key, which is free for basic usage.
Here’s a step-by-step guide to creating a simple map using jQuery and the Google Maps API:
- Get a Google Maps API Key:
- Go to the Google Cloud Console: https://console.cloud.google.com/
- Create a new project or select an existing one.
- Enable the “Google Maps JavaScript API” for your project.
- Create an API key for the selected project.
- Create the HTML structure:
<!DOCTYPE html>
<html>
<head>
<title>Simple Map with jQuery</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div id="map"></div>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script src="https://maps.googleapis.com/maps/api/js?key=YOUR_API_KEY&callback=initMap" async defer></script>
<script src="script.js"></script>
</body>
</html>
Replace YOUR_API_KEY
with the API key you obtained in step 1.
- Implement the JavaScript (script.js):
// Initialize the map
function initMap() {
// Coordinates for the center of the map (e.g., New York City)
const centerCoordinates = { lat: 40.7128, lng: -74.0060 };
// Create the map object
const map = new google.maps.Map(document.getElementById('map'), {
center: centerCoordinates,
zoom: 12, // Adjust the zoom level as needed
});
// Create a marker for the center coordinates
const marker = new google.maps.Marker({
position: centerCoordinates,
map: map,
title: 'Hello, this is the center!',
});
}
In this example, we use jQuery to asynchronously load the Google Maps API and call the initMap()
function when it’s ready. Inside the initMap()
function, we create a map centered at the specified coordinates (New York City, in this case) with a zoom level of 12. We also add a marker to the center of the map.
- Create the CSS (styles.css) – Optional:
You can customize the CSS to set the size and appearance of the map container (#map
) if needed.
You will have a simple map displayed on the webpage using the Google Maps API and jQuery. The map will center around the specified coordinates, and a marker will indicate the center point.
Remember to replace YOUR_API_KEY
with your actual Google Maps API key for it to work correctly. Additionally, you can customize the map by adding more markers, adjusting the initial coordinates, changing the zoom level, and implementing other features provided by the Google Maps JavaScript API.