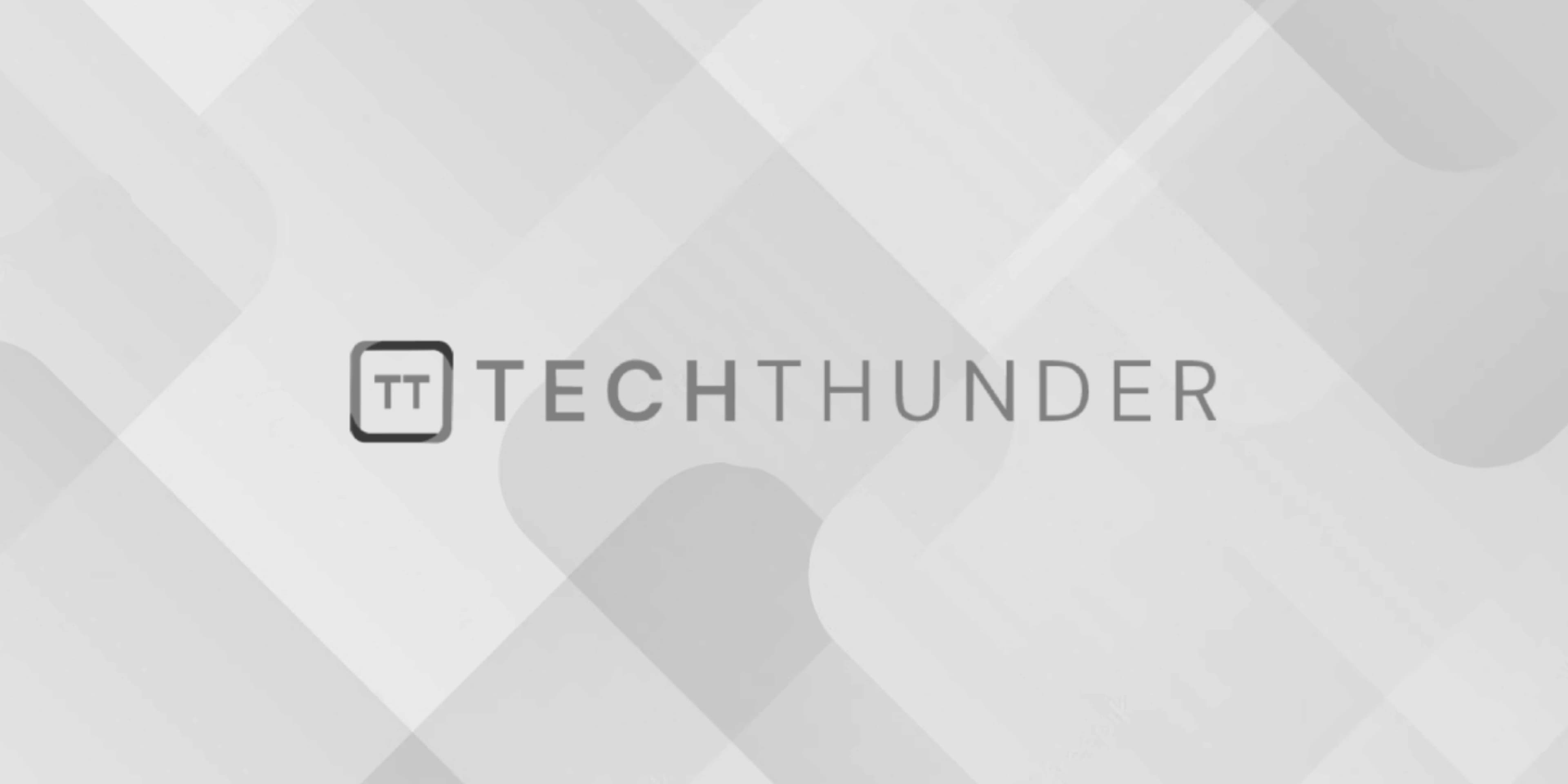
jQuery grep() method
The jQuery grep()
method is used to filter an array based on a specified condition and create a new array containing only the elements that match the condition. It is similar to the filter()
method but works specifically on regular JavaScript arrays.
Here’s the basic syntax of the grep()
method:
$.grep(array, function(elementOfArray, indexInArray) {
// Condition to filter elements
// Return true if the element should be included in the filtered array
});
Parameters:
array
: The array to be filtered.function(elementOfArray, indexInArray)
: A function that defines the filtering condition. It takes two parameters:elementOfArray
: The current element being processed in the array.indexInArray
: The index of the current element in the array.
Example:
<!DOCTYPE html>
<html>
<head>
<title>jQuery grep() Method Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<div id="output"></div>
<script>
$(document).ready(function() {
var numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
// Use grep() to filter even numbers from the array
var evenNumbers = $.grep(numbers, function(element) {
return element % 2 === 0;
});
// Display the filtered array
$('#output').text('Even Numbers: ' + evenNumbers.join(', '));
});
</script>
</body>
</html>
In this example, we have an array of numbers from 1 to 10. We use the grep()
method to filter out even numbers from the array. The condition element % 2 === 0
checks if the element is divisible by 2 (i.e., it is even). The resulting array, containing only the even numbers, is displayed in the “output” div.
The grep()
method is useful when you need to selectively filter elements from an array based on specific criteria. It allows you to create a new array with the elements that meet the condition defined in the filtering function.