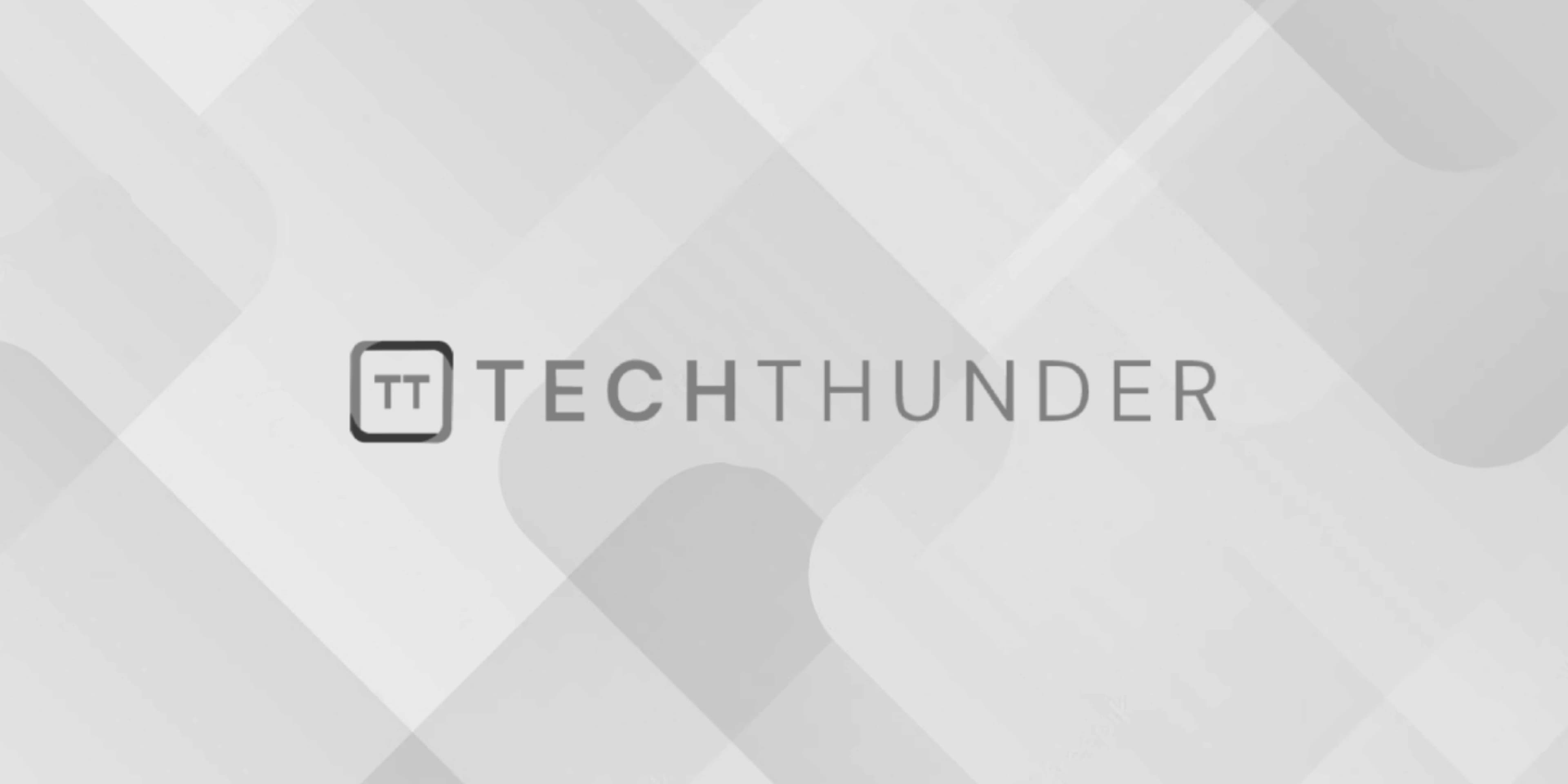
JQuery Sort
The Array.prototype.sort()
method sorts the elements of an array in place and returns the sorted array. You can use it to sort an array of elements in ascending or descending order based on a given criteria or sorting function.
Here’s an example of how you can use Array.prototype.sort()
with jQuery to sort a list of elements:
HTML:
<!DOCTYPE html>
<html>
<head>
<title>jQuery Sort Example</title>
</head>
<body>
<ul id="sortable">
<li>Apple</li>
<li>Banana</li>
<li>Orange</li>
<li>Mango</li>
</ul>
<button id="sortButton">Sort Alphabetically</button>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script src="script.js"></script>
</body>
</html>
JavaScript (script.js):
$(document).ready(function() {
// Initialize the jQuery UI sortable widget to enable drag-and-drop sorting
$("#sortable").sortable();
// Sort the list alphabetically when the button is clicked
$("#sortButton").click(function() {
sortListAlphabetically();
});
});
function sortListAlphabetically() {
var listItems = $("#sortable li").get();
listItems.sort(function(a, b) {
return $(a).text().localeCompare($(b).text());
});
$("#sortable").append(listItems);
}
We have an unordered list (<ul>
) with the ID sortable
, containing several list items (<li>
) with different fruit names. The jQuery UI sortable widget is initialized to enable drag-and-drop sorting of the list items.
When the “Sort Alphabetically” button is clicked, the sortListAlphabetically()
function is called. This function gets the list items, sorts them alphabetically using the Array.prototype.sort()
method with the help of the localeCompare()
function to compare text in a locale-sensitive way, and then appends the sorted list items back to the unordered list. This will result in a sorted list of fruits.
Keep in mind that this example uses jQuery UI for drag-and-drop sorting, but the actual sorting of the list items is done using JavaScript’s native Array.prototype.sort()
method.