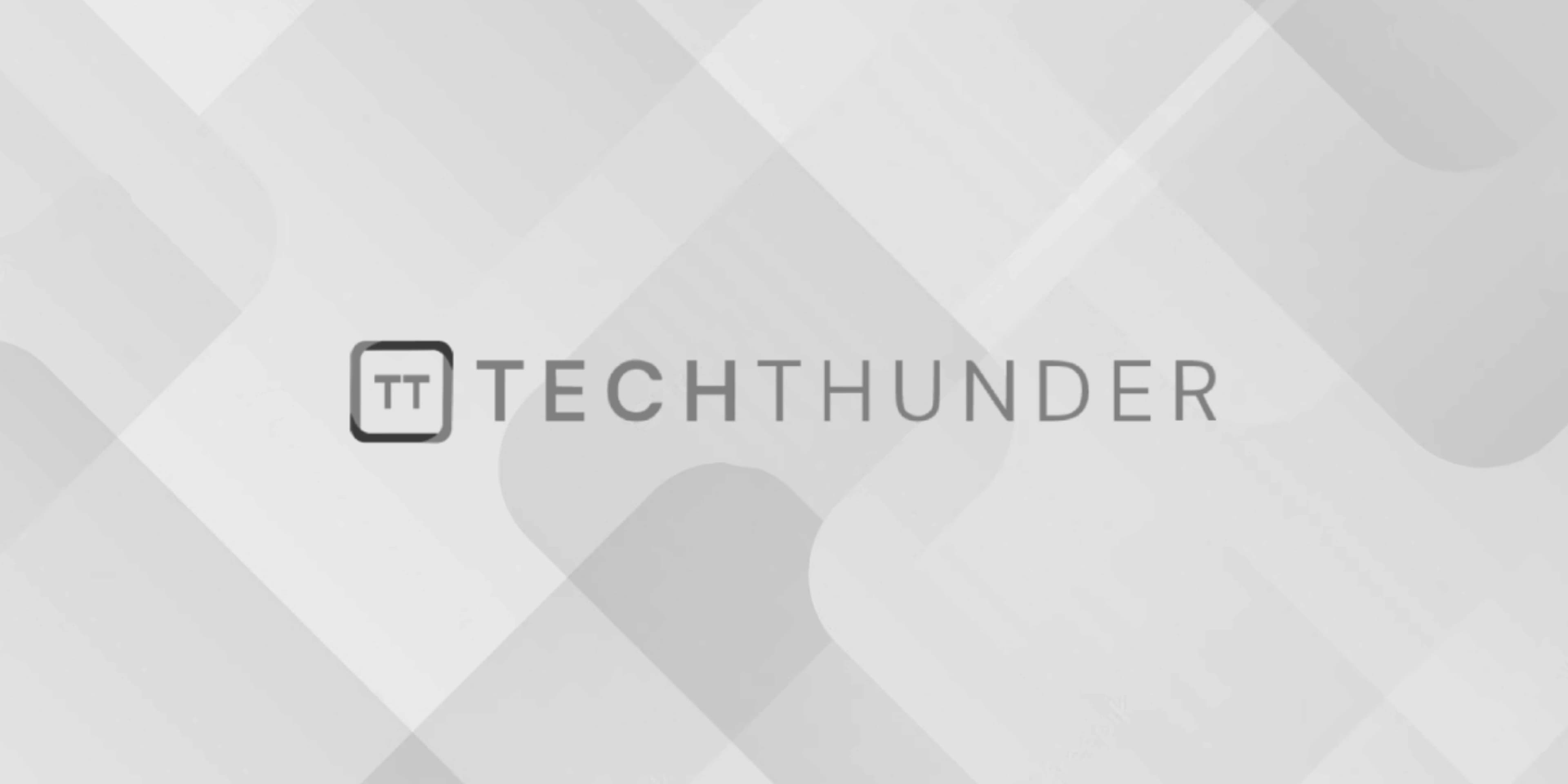
jQuery parseJSON() method
The jQuery.parseJSON()
method is used to parse a JSON string into a JavaScript object. JSON (JavaScript Object Notation) is a lightweight data interchange format widely used to exchange data between a server and a web application.
Here’s the basic syntax of the parseJSON()
method:
jQuery.parseJSON(jsonString)
Parameter:
jsonString
: The JSON string that you want to parse into a JavaScript object.
Return Value:
The parseJSON()
method returns a JavaScript object that corresponds to the parsed JSON data.
Example:
var jsonString = '{"name": "John", "age": 30, "city": "New York"}';
var parsedObject = jQuery.parseJSON(jsonString);
console.log(parsedObject.name); // Output: "John"
console.log(parsedObject.age); // Output: 30
console.log(parsedObject.city); // Output: "New York"
In this example, we have a JSON string jsonString
representing an object with properties such as “name,” “age,” and “city.” The parseJSON()
method is used to convert this JSON string into a JavaScript object, and then we can access the properties of the object using dot notation.
Keep in mind that the parseJSON()
method will throw an error if the provided JSON string is not valid. It is essential to ensure that the JSON string is well-formed before attempting to parse it using this method.
Note: Since jQuery 3.0, the preferred way to parse JSON is to use the native JSON.parse()
method provided by modern JavaScript environments. If you are using a version of jQuery that supports JSON.parse()
(3.0 and above), it is recommended to use JSON.parse()
instead of jQuery.parseJSON()
. Here’s how you can use JSON.parse()
:
var jsonString = '{"name": "John", "age": 30, "city": "New York"}';
var parsedObject = JSON.parse(jsonString);
console.log(parsedObject.name); // Output: "John"
console.log(parsedObject.age); // Output: 30
console.log(parsedObject.city); // Output: "New York"
Both jQuery.parseJSON()
and JSON.parse()
perform the same function of converting a JSON string into a JavaScript object, but using JSON.parse()
leverages the native support for JSON parsing in modern JavaScript environments.